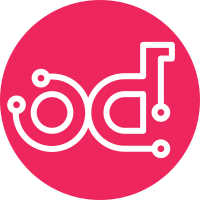
This creates a new module, suitemaker.py, which contains the code used to create a suite.GabbiSuite, which contains case.HTTPTestCase tests. This limits driver.py to being mostly code that is necessary to assemble the necessary information to create tests that are runnable by python test harnesses. This is distinguished from runner.py which assembles the necessary information to both create and _run_ tests itself. The idea here is that if the interface presented by test_suite_from_dict (both signature and that it returns a GabbiSuite) is preserved, then anything in suitemaker.py is welcome to change. The signature on test_suite_from_dict is the definition of what a caller (driver or runner or whatever else) needs to have learned: loader: the unittest.TestLoader which we might be able to default out of existence test_base_name: names help with test grouping so useful suite_dict: the main piece of the pie, the dict test_directory: the place where `data` can load files from host: the host any unqualified test goes to port: the port any unqualified test goes to fixture_module: the sole module from which fixtures come intercept: the wsgi app to intercept if any prefix: the url prefix host and intercept are mutually exclusive One can image it might be possible to replace host, port, prefix with a URL instead. If that were done than host and url would be mutually exclusive. If the signature of test_suite_from_dict changes then stuff in both driver and runner, in how they assemble the required info, would need to change. Note: For reasons unclear these changes have exposed some state management issues in test_runner, noted in a NOTE.
121 lines
4.9 KiB
Python
121 lines
4.9 KiB
Python
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import unittest
|
|
|
|
from gabbi import exception
|
|
from gabbi import suitemaker
|
|
|
|
|
|
class SuiteMakerTest(unittest.TestCase):
|
|
|
|
def setUp(self):
|
|
super(SuiteMakerTest, self).setUp()
|
|
self.loader = unittest.defaultTestLoader
|
|
|
|
def test_tests_key_required(self):
|
|
test_yaml = {'name': 'house', 'url': '/'}
|
|
|
|
with self.assertRaises(exception.GabbiFormatError) as failure:
|
|
suitemaker.test_suite_from_dict(self.loader, 'foo', test_yaml, '.',
|
|
'localhost', 80, None, None)
|
|
self.assertEqual('malformed test file, "tests" key required',
|
|
str(failure.exception))
|
|
|
|
def test_upper_dict_required(self):
|
|
test_yaml = [{'name': 'house', 'url': '/'}]
|
|
with self.assertRaises(exception.GabbiFormatError) as failure:
|
|
suitemaker.test_suite_from_dict(self.loader, 'foo', test_yaml, '.',
|
|
'localhost', 80, None, None)
|
|
self.assertEqual('malformed test file, invalid format',
|
|
str(failure.exception))
|
|
|
|
def test_inner_list_required(self):
|
|
test_yaml = {'tests': {'name': 'house', 'url': '/'}}
|
|
with self.assertRaises(exception.GabbiFormatError) as failure:
|
|
suitemaker.test_suite_from_dict(self.loader, 'foo', test_yaml, '.',
|
|
'localhost', 80, None, None)
|
|
self.assertIn('test chunk is not a dict at',
|
|
str(failure.exception))
|
|
|
|
def test_name_key_required(self):
|
|
test_yaml = {'tests': [{'url': '/'}]}
|
|
|
|
with self.assertRaises(exception.GabbiFormatError) as failure:
|
|
suitemaker.test_suite_from_dict(self.loader, 'foo', test_yaml, '.',
|
|
'localhost', 80, None, None)
|
|
self.assertEqual('Test name missing in a test in foo.',
|
|
str(failure.exception))
|
|
|
|
def test_url_key_required(self):
|
|
test_yaml = {'tests': [{'name': 'missing url'}]}
|
|
|
|
with self.assertRaises(exception.GabbiFormatError) as failure:
|
|
suitemaker.test_suite_from_dict(self.loader, 'foo', test_yaml, '.',
|
|
'localhost', 80, None, None)
|
|
self.assertEqual('Test url missing in test foo_missing_url.',
|
|
str(failure.exception))
|
|
|
|
def test_unsupported_key_errors(self):
|
|
test_yaml = {'tests': [{
|
|
'url': '/',
|
|
'name': 'simple',
|
|
'bad_key': 'wow',
|
|
}]}
|
|
|
|
with self.assertRaises(exception.GabbiFormatError) as failure:
|
|
suitemaker.test_suite_from_dict(self.loader, 'foo', test_yaml, '.',
|
|
'localhost', 80, None, None)
|
|
self.assertIn("Invalid test keys used in test foo_simple:",
|
|
str(failure.exception))
|
|
|
|
def test_method_url_pair_format_error(self):
|
|
test_yaml = {'defaults': {'GET': '/foo'}, 'tests': []}
|
|
with self.assertRaises(exception.GabbiFormatError) as failure:
|
|
suitemaker.test_suite_from_dict(self.loader, 'foo', test_yaml, '.',
|
|
'localhost', 80, None, None)
|
|
self.assertIn('"METHOD: url" pairs not allowed in defaults',
|
|
str(failure.exception))
|
|
|
|
def test_method_url_pair_duplication_format_error(self):
|
|
test_yaml = {'tests': [{
|
|
'GET': '/',
|
|
'POST': '/',
|
|
'name': 'duplicate methods',
|
|
}]}
|
|
with self.assertRaises(exception.GabbiFormatError) as failure:
|
|
suitemaker.test_suite_from_dict(self.loader, 'foo', test_yaml, '.',
|
|
'localhost', 80, None, None)
|
|
self.assertIn(
|
|
'duplicate method/URL directive in "foo_duplicate_methods"',
|
|
str(failure.exception)
|
|
)
|
|
|
|
def test_dict_on_invalid_key(self):
|
|
test_yaml = {'tests': [{
|
|
'name': '...',
|
|
'GET': '/',
|
|
'response_html': {
|
|
'foo': 'hello',
|
|
'bar': 'world',
|
|
}
|
|
}]}
|
|
|
|
with self.assertRaises(exception.GabbiFormatError) as failure:
|
|
suitemaker.test_suite_from_dict(self.loader, 'foo', test_yaml, '.',
|
|
'localhost', 80, None, None)
|
|
self.assertIn(
|
|
"invalid key in test: 'response_html'",
|
|
str(failure.exception)
|
|
)
|