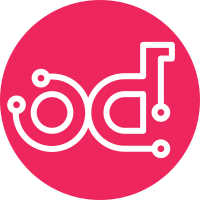
There were changes to the disk partitioner code in ironic project to support local boot, local boot with uefi, uefi support in agent driver, deploy whole disk, etc. These changes should be merged in ironic-lib before we replace all disk partitioner code in ironic with ironic-lib. The ironic changes were part of the following commit SHA. file: ironic/common/disk_partitioner.py 0f4eba18544469d826b4d2d4b420d83c646af463 8c07c4fda3e6a86a40aa00759652b99acbd73331 4e0a78633996d27e21d899661a6414bbea77a0d7 b7e8a8eb51dcd00ce06c9151ad7c8bca8391210e file: ironic/drivers/modules/deploy_utils.py e8eeba4c0d063eac3c283b4af92608c7a6d73e7d cb2e58207203b210d91dacca3c40d91ed1c45a24 987302450af73940794e53233129e90cd4b950f7 da9ed8d0eb1c5d467b41f61185501c26ea2e4ce5 222c84fff52be8383b26495c37df28cc5a0f98b9 dedb425770a6f91a54e990159843ac201dc2f1a5 Change-Id: I6afdaf6a173c1caa9daccb62cb15b630391f161b Partially-implements: blueprint partition-image-support-for-agent-driver
102 lines
3.0 KiB
Python
Executable File
102 lines
3.0 KiB
Python
Executable File
# Copyright 2010 United States Government as represented by the
|
|
# Administrator of the National Aeronautics and Space Administration.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""Ironic base exception handling.
|
|
|
|
Includes decorator for re-raising Ironic-type exceptions.
|
|
|
|
SHOULD include dedicated exception logging.
|
|
|
|
"""
|
|
|
|
import logging
|
|
import six
|
|
|
|
from oslo_config import cfg
|
|
|
|
from ironic_lib.openstack.common._i18n import _
|
|
from ironic_lib.openstack.common._i18n import _LE
|
|
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
exc_log_opts = [
|
|
cfg.BoolOpt('fatal_exception_format_errors',
|
|
default=False,
|
|
help='Make exception message format errors fatal.',
|
|
deprecated_group='DEFAULT'),
|
|
]
|
|
|
|
CONF = cfg.CONF
|
|
CONF.register_opts(exc_log_opts, group='ironic_lib')
|
|
|
|
|
|
class IronicException(Exception):
|
|
"""Base Ironic Exception
|
|
|
|
To correctly use this class, inherit from it and define
|
|
a 'message' property. That message will get printf'd
|
|
with the keyword arguments provided to the constructor.
|
|
|
|
"""
|
|
message = _("An unknown exception occurred.")
|
|
code = 500
|
|
headers = {}
|
|
safe = False
|
|
|
|
def __init__(self, message=None, **kwargs):
|
|
self.kwargs = kwargs
|
|
|
|
if 'code' not in self.kwargs:
|
|
try:
|
|
self.kwargs['code'] = self.code
|
|
except AttributeError:
|
|
pass
|
|
|
|
if not message:
|
|
try:
|
|
message = self.message % kwargs
|
|
|
|
except Exception as e:
|
|
# kwargs doesn't match a variable in the message
|
|
# log the issue and the kwargs
|
|
LOG.exception(_LE('Exception in string format operation'))
|
|
for name, value in kwargs.iteritems():
|
|
LOG.error("%s: %s" % (name, value))
|
|
|
|
if CONF.ironic_lib.fatal_exception_format_errors:
|
|
raise e
|
|
else:
|
|
# at least get the core message out if something happened
|
|
message = self.message
|
|
|
|
super(IronicException, self).__init__(message)
|
|
|
|
def format_message(self):
|
|
if self.__class__.__name__.endswith('_Remote'):
|
|
return self.args[0]
|
|
else:
|
|
return six.text_type(self)
|
|
|
|
|
|
class InstanceDeployFailure(IronicException):
|
|
message = _("Failed to deploy instance: %(reason)s")
|
|
|
|
|
|
class FileSystemNotSupported(IronicException):
|
|
message = _("Failed to create a file system. "
|
|
"File system %(fs)s is not supported.")
|