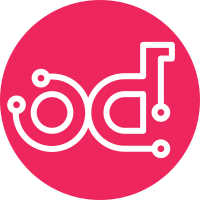
We need to keep hold of the strings which we create. We must also hold on to the array of strings which we assing to our git_strarray. We were not doing the latter, which meant that our strings may have been freed too early, leaving us with with memory access errors (though often not leading to a crash due to the custom allocator in python). As we need to keep hold of two/three pieces of information, this looks like a good place to introduce a context manager. This allows us to keep these pointers alive without burdening the call sites with a return of multiple objects they have no use for.
84 lines
2.6 KiB
Python
84 lines
2.6 KiB
Python
# -*- coding: utf-8 -*-
|
|
#
|
|
# Copyright 2010-2014 The pygit2 contributors
|
|
#
|
|
# This file is free software; you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License, version 2,
|
|
# as published by the Free Software Foundation.
|
|
#
|
|
# In addition to the permissions in the GNU General Public License,
|
|
# the authors give you unlimited permission to link the compiled
|
|
# version of this file into combinations with other programs,
|
|
# and to distribute those combinations without any restriction
|
|
# coming from the use of this file. (The General Public License
|
|
# restrictions do apply in other respects; for example, they cover
|
|
# modification of the file, and distribution when not linked into
|
|
# a combined executable.)
|
|
#
|
|
# This file is distributed in the hope that it will be useful, but
|
|
# WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
# General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with this program; see the file COPYING. If not, write to
|
|
# the Free Software Foundation, 51 Franklin Street, Fifth Floor,
|
|
# Boston, MA 02110-1301, USA.
|
|
|
|
# Import from the future
|
|
from __future__ import absolute_import
|
|
|
|
# Import from the Standard Library
|
|
from sys import version_info
|
|
|
|
# Import from pygit2
|
|
from .ffi import ffi
|
|
|
|
|
|
if version_info[0] < 3:
|
|
from .py2 import is_string, to_bytes, to_str
|
|
else:
|
|
from .py3 import is_string, to_bytes, to_str
|
|
|
|
|
|
|
|
def strarray_to_strings(arr):
|
|
l = [None] * arr.count
|
|
for i in range(arr.count):
|
|
l[i] = ffi.string(arr.strings[i]).decode()
|
|
|
|
return l
|
|
|
|
|
|
class StrArray(object):
|
|
"""A git_strarray wrapper
|
|
|
|
Use this in order to get a git_strarray* to pass to libgit2 out of a
|
|
list of strings. This has a context manager, which you should use, e.g.
|
|
|
|
with StrArray(list_of_strings) as arr:
|
|
C.git_function_that_takes_strarray(arr)
|
|
"""
|
|
|
|
def __init__(self, l):
|
|
if not isinstance(l, list):
|
|
raise TypeError("Value must be a list")
|
|
|
|
arr = ffi.new('git_strarray *')
|
|
strings = [None] * len(l)
|
|
for i in range(len(l)):
|
|
if not is_string(l[i]):
|
|
raise TypeError("Value must be a string")
|
|
|
|
strings[i] = ffi.new('char []', to_bytes(l[i]))
|
|
|
|
self._arr = ffi.new('char *[]', strings)
|
|
self._strings = strings
|
|
self.array = ffi.new('git_strarray *', [self._arr, len(strings)])
|
|
|
|
def __enter__(self):
|
|
return self.array
|
|
|
|
def __exit__(self, type, value, traceback):
|
|
pass
|