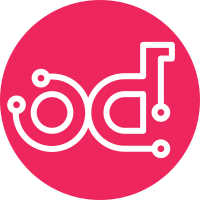
The freezer scheduler is to be executed as daemon process on the client machines It has the following responsibilities: * when using the api: - register -if necessary- as a client in the api - download the list of jobs from the api - schedule the jobs for execution - launch the freezer client at the scheduled time - collect metadata and exit codes and upload them to the api - periodically poll the api for new/updated jobs - if a job is part of a session (a coordinated group of jobs) it updates the session status when job starts/stops * when not using the api - load jobs configurations from files - schedule the jobs for execution - launch the freezer client at the scheduled time The freezer scheduler can also be used to manage jobs and sessions using the following positional parameters: job-list job-get job-create job-delete job-start job-stop session-list session-get session-create session-delete session-list-job session-add-job session-remove-job or to register the client in the api using the positional parameter: register Implements blueprint: freezer-scheduler-start Change-Id: I06ae202a0f464f7240c137744a5b54d1177cabd9
133 lines
6.0 KiB
Python
133 lines
6.0 KiB
Python
"""
|
|
Copyright 2015 Hewlett-Packard
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
|
|
This product includes cryptographic software written by Eric Young
|
|
(eay@cryptsoft.com). This product includes software written by Tim
|
|
Hudson (tjh@cryptsoft.com).
|
|
========================================================================
|
|
"""
|
|
|
|
import unittest
|
|
from mock import Mock, patch
|
|
|
|
from freezer.apiclient import exceptions
|
|
from freezer.apiclient import actions
|
|
|
|
|
|
class TestActionManager(unittest.TestCase):
|
|
|
|
def setUp(self):
|
|
self.mock_client = Mock()
|
|
self.mock_response = Mock()
|
|
self.mock_client.endpoint = 'http://testendpoint:9999'
|
|
self.mock_client.auth_token = 'testtoken'
|
|
self.mock_client.client_id = 'test_client_id_78900987'
|
|
self.action_manager = actions.ActionManager(self.mock_client)
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_create(self, mock_requests):
|
|
self.assertEqual(self.action_manager.endpoint, 'http://testendpoint:9999/v1/actions/')
|
|
self.assertEqual(self.action_manager.headers, {'X-Auth-Token': 'testtoken'})
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_create_ok(self, mock_requests):
|
|
self.mock_response.status_code = 201
|
|
self.mock_response.json.return_value = {'action_id': 'qwerqwer'}
|
|
mock_requests.post.return_value = self.mock_response
|
|
retval = self.action_manager.create({'action': 'metadata'})
|
|
self.assertEqual(retval, 'qwerqwer')
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_create_fail_when_api_return_error_code(self, mock_requests):
|
|
self.mock_response.status_code = 500
|
|
mock_requests.post.return_value = self.mock_response
|
|
self.assertRaises(exceptions.ApiClientException, self.action_manager.create, {'action': 'metadata'})
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_delete_ok(self, mock_requests):
|
|
self.mock_response.status_code = 204
|
|
mock_requests.delete.return_value = self.mock_response
|
|
retval = self.action_manager.delete('test_action_id')
|
|
self.assertIsNone(retval)
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_delete_fail(self, mock_requests):
|
|
self.mock_response.status_code = 500
|
|
mock_requests.delete.return_value = self.mock_response
|
|
self.assertRaises(exceptions.ApiClientException, self.action_manager.delete, 'test_action_id')
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_get_ok(self, mock_requests):
|
|
self.mock_response.status_code = 200
|
|
self.mock_response.json.return_value = {'action_id': 'qwerqwer'}
|
|
mock_requests.get.return_value = self.mock_response
|
|
retval = self.action_manager.get('test_action_id')
|
|
self.assertEqual(retval, {'action_id': 'qwerqwer'})
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_get_fails_on_error_different_from_404(self, mock_requests):
|
|
self.mock_response.status_code = 500
|
|
mock_requests.get.return_value = self.mock_response
|
|
self.assertRaises(exceptions.ApiClientException, self.action_manager.get, 'test_action_id')
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_get_none(self, mock_requests):
|
|
self.mock_response.status_code = 404
|
|
mock_requests.get.return_value = self.mock_response
|
|
retval = self.action_manager.get('test_action_id')
|
|
self.assertIsNone(retval)
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_list_ok(self, mock_requests):
|
|
self.mock_response.status_code = 200
|
|
action_list = [{'action_id_0': 'bomboloid'}, {'action_id_1': 'asdfasdf'}]
|
|
self.mock_response.json.return_value = {'actions': action_list}
|
|
mock_requests.get.return_value = self.mock_response
|
|
retval = self.action_manager.list()
|
|
self.assertEqual(retval, action_list)
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_list_error(self, mock_requests):
|
|
self.mock_response.status_code = 404
|
|
action_list = [{'action_id_0': 'bomboloid'}, {'action_id_1': 'asdfasdf'}]
|
|
self.mock_response.json.return_value = {'clients': action_list}
|
|
mock_requests.get.return_value = self.mock_response
|
|
self.assertRaises(exceptions.ApiClientException, self.action_manager.list)
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_update_ok(self, mock_requests):
|
|
self.mock_response.status_code = 200
|
|
self.mock_response.json.return_value = {
|
|
"patch": {"status": "bamboozled"},
|
|
"version": 12,
|
|
"action_id": "d454beec-1f3c-4d11-aa1a-404116a40502"
|
|
}
|
|
mock_requests.patch.return_value = self.mock_response
|
|
retval = self.action_manager.update('d454beec-1f3c-4d11-aa1a-404116a40502', {'status': 'bamboozled'})
|
|
self.assertEqual(retval, 12)
|
|
|
|
@patch('freezer.apiclient.actions.requests')
|
|
def test_update_raise_MetadataUpdateFailure_when_api_return_error_code(self, mock_requests):
|
|
self.mock_response.json.return_value = {
|
|
"patch": {"status": "bamboozled"},
|
|
"version": 12,
|
|
"action_id": "d454beec-1f3c-4d11-aa1a-404116a40502"
|
|
}
|
|
self.mock_response.status_code = 404
|
|
self.mock_response.text = '{"title": "Not Found","description":"No document found with ID d454beec-1f3c-4d11-aa1a-404116a40502x"}'
|
|
mock_requests.patch.return_value = self.mock_response
|
|
self.assertRaises(exceptions.ApiClientException, self.action_manager.update,
|
|
'd454beec-1f3c-4d11-aa1a-404116a40502', {'status': 'bamboozled'})
|