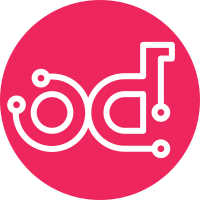
The simulatir tool can be used either to virtually run the deployment to find out the task run order or to plot the entire graph or its subset. > Run deployment from the YAML dumped by Astute astute-simulator -y /path/to/yaml/file.yaml > Run deployments with task failure emulation astute-simulator -y /path/to/yaml/file.yaml -f ntp-client/2,heat-db/1 -P > Using node and task name filters astute-simulator -y /path/to/yaml/file.yaml -g openstack -G '^2$' -p Related-bug: #1569839 Change-Id: I6f583347e2f039a470410900c38d7a1d70151b56
57 lines
1.7 KiB
Ruby
Executable File
57 lines
1.7 KiB
Ruby
Executable File
#!/usr/bin/env ruby
|
|
# Copyright 2015 Mirantis, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
require_relative '../lib/fuel_deployment/simulator'
|
|
|
|
simulator = Astute::Simulator.new
|
|
cluster = Deployment::TestCluster.new
|
|
cluster.id = 'node_concurrency'
|
|
|
|
node1 = Deployment::TestNode.new 'node1', cluster
|
|
node2 = Deployment::TestNode.new 'node2', cluster
|
|
node3 = Deployment::TestNode.new 'node3', cluster
|
|
node4 = Deployment::TestNode.new 'node4', cluster
|
|
node5 = Deployment::TestNode.new 'node5', cluster
|
|
node6 = Deployment::TestNode.new 'node6', cluster
|
|
|
|
node1.add_new_task('task1')
|
|
node1.add_new_task('final')
|
|
|
|
node2.add_new_task('task1')
|
|
node2.add_new_task('final')
|
|
|
|
node3.add_new_task('task1')
|
|
node3.add_new_task('final')
|
|
|
|
node4.add_new_task('task1')
|
|
node4.add_new_task('final')
|
|
|
|
node5.add_new_task('task1')
|
|
node5.add_new_task('final')
|
|
|
|
node6.add_new_task('task1')
|
|
node6.add_new_task('final')
|
|
|
|
node1['final'].after node1['task1']
|
|
node2['final'].after node2['task1']
|
|
node3['final'].after node3['task1']
|
|
node4['final'].after node4['task1']
|
|
node5['final'].after node5['task1']
|
|
node6['final'].after node6['task1']
|
|
|
|
cluster.node_concurrency.maximum = 2
|
|
|
|
simulator.run cluster
|