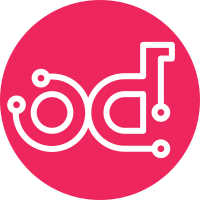
* Add CentOS6 and CentOS7 separation to l23_os fact. * Use l23_os fact instead of osfamily. * Add CentOS7/RedHat7 support to l23_stored_config resource. * Remove *vconfig* package due to we do not use *vconfig* to configure vlan interfaces hence we do not need to install it. CentOS7/RedHat7 does not have this package anymore as well. Change-Id: Ia6cb9e4efc9465030fe0ed8c2e46922db1513a74 Closes-bug: #1503157
308 lines
7.1 KiB
Ruby
308 lines
7.1 KiB
Ruby
require 'spec_helper'
|
|
|
|
describe 'l23network::l3::ifconfig', :type => :define do
|
|
context 'without IP address definition' do
|
|
let(:title) { 'without IP address definition' }
|
|
let(:facts) { {
|
|
:osfamily => 'Debian',
|
|
:operatingsystem => 'Ubuntu',
|
|
:l23_os => 'ubuntu',
|
|
:kernel => 'Linux'
|
|
} }
|
|
|
|
let(:params) { {
|
|
:interface => 'eth4',
|
|
:ipaddr => 'none'
|
|
} }
|
|
|
|
let(:pre_condition) { [
|
|
"class {'l23network': }"
|
|
] }
|
|
|
|
before(:each) do
|
|
puppet_debug_override()
|
|
end
|
|
|
|
it do
|
|
should compile.with_all_deps
|
|
end
|
|
|
|
it do
|
|
should contain_l23_stored_config('eth4').only_with({
|
|
'ensure' => 'present',
|
|
'name' => 'eth4',
|
|
'method' => 'manual',
|
|
'ipaddr' => 'none',
|
|
'ipaddr_aliases' => nil,
|
|
})
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('eth4').with({
|
|
'ensure' => 'present',
|
|
'ipaddr' => 'none',
|
|
'gateway' => nil,
|
|
})
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('eth4').that_requires('L23_stored_config[eth4]')
|
|
should contain_l3_ifconfig('eth4').that_requires('L23network::L2::Port[eth4]')
|
|
end
|
|
end
|
|
|
|
context 'with IP address and default gateway' do
|
|
let(:title) { 'ifconfig simple test' }
|
|
let(:facts) { {
|
|
:osfamily => 'Debian',
|
|
:operatingsystem => 'Ubuntu',
|
|
:l23_os => 'ubuntu',
|
|
:kernel => 'Linux'
|
|
} }
|
|
|
|
let(:params) { {
|
|
:interface => 'eth4',
|
|
:ipaddr => ['10.20.20.2/24'],
|
|
:gateway => '10.20.20.1',
|
|
} }
|
|
|
|
before(:each) do
|
|
puppet_debug_override()
|
|
end
|
|
|
|
it do
|
|
should compile.with_all_deps
|
|
end
|
|
|
|
it do
|
|
should contain_l23_stored_config('eth4').with({
|
|
'ensure' => 'present',
|
|
'name' => 'eth4',
|
|
'method' => 'static',
|
|
'ipaddr' => '10.20.20.2/24',
|
|
'gateway' => '10.20.20.1',
|
|
'gateway_metric' => nil,
|
|
})
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('eth4').with({
|
|
'ensure' => 'present',
|
|
'ipaddr' => '10.20.20.2/24',
|
|
'gateway' => '10.20.20.1',
|
|
'gateway_metric' => nil,
|
|
})
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('eth4').that_requires('L23_stored_config[eth4]')
|
|
should contain_l3_ifconfig('eth4').that_requires('L23network::L2::Port[eth4]')
|
|
end
|
|
end
|
|
|
|
context 'with default gateway and metric' do
|
|
let(:title) { 'ifconfig simple test' }
|
|
let(:facts) { {
|
|
:osfamily => 'Debian',
|
|
:operatingsystem => 'Ubuntu',
|
|
:l23_os => 'ubuntu',
|
|
:kernel => 'Linux'
|
|
} }
|
|
|
|
let(:params) { {
|
|
:interface => 'eth4',
|
|
:ipaddr => ['10.20.30.2/24'],
|
|
:gateway => '10.20.30.1',
|
|
:gateway_metric => 321,
|
|
} }
|
|
|
|
before(:each) do
|
|
puppet_debug_override()
|
|
end
|
|
|
|
it do
|
|
should compile.with_all_deps
|
|
end
|
|
|
|
it do
|
|
should contain_l23_stored_config('eth4').with({
|
|
'ensure' => 'present',
|
|
'name' => 'eth4',
|
|
'method' => 'static',
|
|
'ipaddr' => '10.20.30.2/24',
|
|
'gateway' => '10.20.30.1',
|
|
'gateway_metric' => 321,
|
|
})
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('eth4').with({
|
|
'ensure' => 'present',
|
|
'ipaddr' => '10.20.30.2/24',
|
|
'gateway' => '10.20.30.1',
|
|
'gateway_metric' => 321,
|
|
})
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('eth4').that_requires('L23_stored_config[eth4]')
|
|
should contain_l3_ifconfig('eth4').that_requires('L23network::L2::Port[eth4]')
|
|
end
|
|
end
|
|
|
|
context 'with multiple IP addresses' do
|
|
let(:title) { 'with multiple IP addresses' }
|
|
let(:facts) { {
|
|
:osfamily => 'Debian',
|
|
:operatingsystem => 'Ubuntu',
|
|
:l23_os => 'ubuntu',
|
|
:kernel => 'Linux'
|
|
} }
|
|
|
|
let(:params) { {
|
|
:interface => 'eth4',
|
|
:ipaddr => ['10.20.20.2/24', '10.30.30.3/24', '10.40.40.4/24'],
|
|
} }
|
|
|
|
before(:each) do
|
|
puppet_debug_override()
|
|
end
|
|
|
|
it do
|
|
should compile.with_all_deps
|
|
end
|
|
|
|
it do
|
|
should contain_l23_stored_config('eth4').with({
|
|
'ensure' => 'present',
|
|
'name' => 'eth4',
|
|
'method' => 'static',
|
|
'ipaddr' => '10.20.20.2/24',
|
|
'ipaddr_aliases' => ['10.30.30.3/24', '10.40.40.4/24'],
|
|
'gateway' => nil,
|
|
'gateway_metric' => nil,
|
|
})
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('eth4').with({
|
|
'ensure' => 'present',
|
|
'ipaddr' => ['10.20.20.2/24', '10.30.30.3/24', '10.40.40.4/24'],
|
|
'gateway' => nil,
|
|
'gateway_metric' => nil,
|
|
})
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('eth4').that_requires('L23_stored_config[eth4]')
|
|
should contain_l3_ifconfig('eth4').that_requires('L23network::L2::Port[eth4]')
|
|
end
|
|
end
|
|
|
|
context 'l3_ifconfig before port' do
|
|
let(:title) { 'ifconfig simple test' }
|
|
let(:facts) { {
|
|
:osfamily => 'Debian',
|
|
:operatingsystem => 'Ubuntu',
|
|
:l23_os => 'ubuntu',
|
|
:kernel => 'Linux'
|
|
} }
|
|
|
|
let :pre_condition do
|
|
'l23network::l2::port{"port-test":}'
|
|
end
|
|
|
|
let(:params) { {
|
|
:interface => 'port-test',
|
|
:ipaddr => ['10.10.10.1/24'],
|
|
} }
|
|
|
|
before(:each) do
|
|
puppet_debug_override()
|
|
end
|
|
|
|
it do
|
|
should compile.with_all_deps
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('port-test').that_requires('L23network::L2::Port[port-test]')
|
|
end
|
|
end
|
|
|
|
context 'l3_ifconfig before bridge' do
|
|
let(:title) { 'ifconfig simple test' }
|
|
let(:facts) { {
|
|
:osfamily => 'Debian',
|
|
:operatingsystem => 'Ubuntu',
|
|
:l23_os => 'ubuntu',
|
|
:kernel => 'Linux'
|
|
} }
|
|
|
|
let :pre_condition do
|
|
'l23network::l2::bridge{"br-test":}'
|
|
end
|
|
|
|
let(:params) { {
|
|
:interface => 'br-test',
|
|
:ipaddr => ['10.10.10.10/24'],
|
|
} }
|
|
|
|
before(:each) do
|
|
puppet_debug_override()
|
|
end
|
|
|
|
it do
|
|
should compile.with_all_deps
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('br-test').that_requires('L23network::L2::Bridge[br-test]')
|
|
end
|
|
end
|
|
|
|
context 'l3_ifconfig before bond' do
|
|
let(:title) { 'ifconfig simple test' }
|
|
let(:facts) { {
|
|
:osfamily => 'Debian',
|
|
:operatingsystem => 'Ubuntu',
|
|
:kernel => 'Linux',
|
|
:l23_os => 'ubuntu',
|
|
:l3_fqdn_hostname => 'stupid_hostname',
|
|
} }
|
|
|
|
let(:pre_condition) { [
|
|
"class {'l23network': }"
|
|
] }
|
|
|
|
let :pre_condition do
|
|
'l23network::l2::bond{"bond-test":
|
|
interfaces => ["eth2", "eth3"],
|
|
bond_properties => {
|
|
mode => "802.3ad",
|
|
},
|
|
provider => "lnx",
|
|
}'
|
|
end
|
|
|
|
let(:params) { {
|
|
:interface => 'bond-test',
|
|
:ipaddr => ['10.10.10.11/24'],
|
|
} }
|
|
|
|
before(:each) do
|
|
puppet_debug_override()
|
|
end
|
|
|
|
it do
|
|
should compile.with_all_deps
|
|
end
|
|
|
|
it do
|
|
should contain_l3_ifconfig('bond-test').that_requires('L23network::L2::Bond[bond-test]')
|
|
end
|
|
end
|
|
|
|
end
|
|
# vim: set ts=2 sw=2 et
|