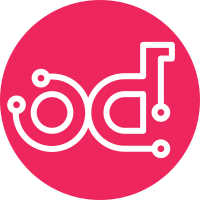
Introduce infrastructure: * Executor (The Asynchronous task executor) * ConnectionsPool (The pool of network connections) * ResumeableStream - allows resume streaming when error occures Change-Id: Ia68d60c2b9d685820a4c1916c8f1aa724f3a3f91 Implements: blueprint refactor-local-mirror-scripts Partial-Bug: #1487077
51 lines
1.8 KiB
Python
51 lines
1.8 KiB
Python
# -*- coding: utf-8 -*-
|
|
|
|
# Copyright 2015 Mirantis, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import six
|
|
|
|
from packetary.library import checksum
|
|
from packetary.tests import base
|
|
|
|
|
|
class TestChecksum(base.TestCase):
|
|
def test_checksum(self):
|
|
stream = six.BytesIO(b"line1\nline2\nline3\n")
|
|
checksums = {
|
|
checksum.md5: "cc3d5ed5fda53dfa81ea6aa951d7e1fe",
|
|
checksum.sha1: "8c84f6f36dd2230d3e9c954fa436e5fda90b1957",
|
|
checksum.sha256: "66663af9c7aa341431a8ee2ff27b72"
|
|
"abd06c9218f517bb6fef948e4803c19e03"
|
|
}
|
|
for chunksize in (8, 256):
|
|
for algo, expected in six.iteritems(checksums):
|
|
stream.seek(0)
|
|
self.assertEqual(
|
|
expected, algo(stream, chunksize)
|
|
)
|
|
|
|
def test_composite(self):
|
|
stream = six.BytesIO(b"line1\nline2\nline3\n")
|
|
result = checksum.composite('md5', 'sha1', 'sha256')(stream)
|
|
self.assertEqual(
|
|
[
|
|
"cc3d5ed5fda53dfa81ea6aa951d7e1fe",
|
|
"8c84f6f36dd2230d3e9c954fa436e5fda90b1957",
|
|
"66663af9c7aa341431a8ee2ff27b72"
|
|
"abd06c9218f517bb6fef948e4803c19e03"
|
|
],
|
|
result
|
|
)
|