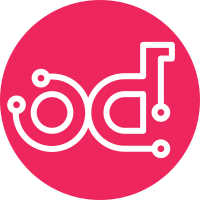
It was moved out of app.js dependencies and made a separate entry point - this will guarantee it will be loaded before any other module which might use ES6-specific objects and methods. Change-Id: I4899d53112720131cf8634d8a7f489878d096639
52 lines
1.6 KiB
JavaScript
52 lines
1.6 KiB
JavaScript
/*eslint-disable strict*/
|
|
module.exports = {
|
|
entry: [
|
|
'babel-core/polyfill',
|
|
'./static/app.js'
|
|
],
|
|
output: {
|
|
path: __dirname + '/static/build/',
|
|
publicPath: '/static/build/',
|
|
filename: 'bundle.js',
|
|
chunkFilename: null,
|
|
sourceMapFilename: 'bundle.js.map'
|
|
},
|
|
module: {
|
|
loaders: [
|
|
{
|
|
test: /\.js$/,
|
|
loader: 'babel',
|
|
exclude: [/(node_modules|vendor\/custom)\//, /\/expression\/parser\.js$/],
|
|
query: {cacheDirectory: true}
|
|
},
|
|
{test: /\/expression\/parser\.js$/, loader: 'exports?parser'},
|
|
{test: require.resolve('jquery'), loader: 'expose?jQuery!expose?$'},
|
|
{test: /\.css$/, loader: 'style!css!postcss'},
|
|
{test: /\.less$/, loader: 'style!css!postcss!less'},
|
|
{test: /\.html$/, loader: 'raw'},
|
|
{test: /\.json$/, loader: 'json'},
|
|
{test: /\.jison$/, loader: 'jison'},
|
|
{test: /\.(gif|png)$/, loader: 'file'},
|
|
{test: /\.(woff|woff2|ttf|eot|svg)(\?v=\d+\.\d+\.\d+)?$/, loader: 'file'}
|
|
]
|
|
},
|
|
resolve: {
|
|
modulesDirectories: ['static', 'node_modules', 'vendor/custom'],
|
|
extensions: ['', '.js'],
|
|
alias: {
|
|
underscore: 'lodash',
|
|
react: 'react/addons'
|
|
}
|
|
},
|
|
node: {},
|
|
plugins: [],
|
|
postcss: function() {
|
|
return [require('autoprefixer')];
|
|
},
|
|
devtool: 'cheap-module-source-map',
|
|
watchOptions: {
|
|
aggregateTimeout: 300,
|
|
poll: 1000
|
|
}
|
|
};
|