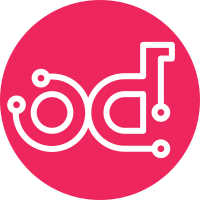
We were capped at a very old version of hacking. Hacking itself caps the various linters it uses to remain consistent, so our pep8 job was not checking quite a bit that current versions have added. This raises that limit to the latest to get up to the level of other projects and addresses the errors the updated linters uncovered. Change-Id: I89a9d73fbd59606a649e26077acebc5c42873d67 Co-authored-by: Sean McGinnis <sean.mcginnis@gmail.com>
91 lines
2.8 KiB
Python
91 lines
2.8 KiB
Python
# Copyright 2011 OpenStack Foundation.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
Time related utilities and helper functions.
|
|
"""
|
|
|
|
import datetime
|
|
|
|
import iso8601
|
|
from oslo_utils import encodeutils
|
|
|
|
# ISO 8601 extended time format with microseconds
|
|
_ISO8601_TIME_FORMAT_SUBSECOND = '%Y-%m-%dT%H:%M:%S.%f'
|
|
_ISO8601_TIME_FORMAT = '%Y-%m-%dT%H:%M:%S'
|
|
PERFECT_TIME_FORMAT = _ISO8601_TIME_FORMAT_SUBSECOND
|
|
|
|
|
|
def isotime(at=None, subsecond=False):
|
|
"""Stringify time in ISO 8601 format."""
|
|
if not at:
|
|
at = utcnow()
|
|
st = at.strftime(_ISO8601_TIME_FORMAT
|
|
if not subsecond
|
|
else _ISO8601_TIME_FORMAT_SUBSECOND)
|
|
tz = at.tzinfo.tzname(None) if at.tzinfo else 'UTC'
|
|
# Need to handle either iso8601 or python UTC format
|
|
st += ('Z' if tz in ['UTC', 'UTC+00:00'] else tz)
|
|
return st
|
|
|
|
|
|
def parse_isotime(timestr):
|
|
"""Parse time from ISO 8601 format."""
|
|
try:
|
|
return iso8601.parse_date(timestr)
|
|
except iso8601.ParseError as e:
|
|
raise ValueError(encodeutils.exception_to_unicode(e))
|
|
except TypeError as e:
|
|
raise ValueError(encodeutils.exception_to_unicode(e))
|
|
|
|
|
|
def utcnow(with_timezone=False):
|
|
"""Overridable version of utils.utcnow that can return a TZ-aware datetime.
|
|
"""
|
|
if utcnow.override_time:
|
|
try:
|
|
return utcnow.override_time.pop(0)
|
|
except AttributeError:
|
|
return utcnow.override_time
|
|
if with_timezone:
|
|
return datetime.datetime.now(tz=iso8601.iso8601.UTC)
|
|
return datetime.datetime.utcnow()
|
|
|
|
|
|
def normalize_time(timestamp):
|
|
"""Normalize time in arbitrary timezone to UTC naive object."""
|
|
offset = timestamp.utcoffset()
|
|
if offset is None:
|
|
return timestamp
|
|
return timestamp.replace(tzinfo=None) - offset
|
|
|
|
|
|
def iso8601_from_timestamp(timestamp, microsecond=False):
|
|
"""Returns an iso8601 formatted date from timestamp."""
|
|
return isotime(datetime.datetime.utcfromtimestamp(timestamp), microsecond)
|
|
|
|
|
|
utcnow.override_time = None
|
|
|
|
|
|
def delta_seconds(before, after):
|
|
"""Return the difference between two timing objects.
|
|
|
|
Compute the difference in seconds between two date, time, or
|
|
datetime objects (as a float, to microsecond resolution).
|
|
"""
|
|
delta = after - before
|
|
return datetime.timedelta.total_seconds(delta)
|