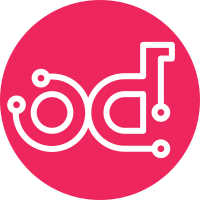
Two things were happening that this patch corrects: a) If adding an image fails, the glance add output says that adding the image failed, however, doing a glance index would show the image. This was because the call to get all public images was not correctly filtering the result for 'active' status images. The image failing to be added causes the image status to be 'killed', and so killed images should not appear in the output of glance index or glance detail. b) glance show <ID> was not showing the status of the image, so it was not clear that the image, while not added successfully, was still in the registry, but in a 'killed' status. I added a note to the output of the failed add command that the Glance registry may still have an image record, but the status would likely be in the 'killed' state. Added a functional test case that verified the behaviour in the bug and verified the fix, once coded.
69 lines
2.2 KiB
Python
69 lines
2.2 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright 2010-2011 OpenStack, LLC
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""Common utilities used in testing"""
|
|
|
|
import os
|
|
import socket
|
|
import subprocess
|
|
|
|
|
|
def execute(cmd, raise_error=True):
|
|
"""
|
|
Executes a command in a subprocess. Returns a tuple
|
|
of (exitcode, out, err), where out is the string output
|
|
from stdout and err is the string output from stderr when
|
|
executing the command.
|
|
|
|
:param cmd: Command string to execute
|
|
:param raise_error: If returncode is not 0 (success), then
|
|
raise a RuntimeError? Default: True)
|
|
"""
|
|
|
|
env = os.environ.copy()
|
|
|
|
# Make sure that we use the programs in the
|
|
# current source directory's bin/ directory.
|
|
env['PATH'] = os.path.join(os.getcwd(), 'bin') + ':' + env['PATH']
|
|
process = subprocess.Popen(cmd,
|
|
shell=True,
|
|
stdin=subprocess.PIPE,
|
|
stdout=subprocess.PIPE,
|
|
stderr=subprocess.PIPE,
|
|
env=env)
|
|
result = process.communicate()
|
|
(out, err) = result
|
|
exitcode = process.returncode
|
|
if process.returncode != 0 and raise_error:
|
|
msg = "Command %(cmd)s did not succeed. Returned an exit "\
|
|
"code of %(exitcode)d."\
|
|
"\n\nSTDOUT: %(out)s"\
|
|
"\n\nSTDERR: %(err)s" % locals()
|
|
raise RuntimeError(msg)
|
|
return exitcode, out, err
|
|
|
|
|
|
def get_unused_port():
|
|
"""
|
|
Returns an unused port on localhost.
|
|
"""
|
|
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
|
|
s.bind(('localhost', 0))
|
|
addr, port = s.getsockname()
|
|
s.close()
|
|
return port
|