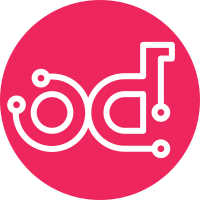
The sync includes change that drastically enhances performance on Python 2.6 with fresh simplejson library installed. The latest commit in oslo-incubator: - 2e1214b74b5448825d3db26e1308a51b468d3d5c Included commits: * gettextutils.py: - de4adbc pep8: fixed multiple violations - 9912e5d Add API for creating translation functions - 6cc96d0 Fix test_gettextutils on Python 3 - fd33d1e Fix gettextutil.Message handling of deep copy failures - 047b2e4 Change lazy translation to retain complete dict * importutils.py: - 1173e46 Remove ValueError when accessing sys.modules - 885828a Deleted duplicated method in cliutils. * jsonutils.py: - 0d7296f Add kwargs to jsonutils.load(s) functions - 18f2bc1 Enforce unicode json output for jsonutils.load[s]() - 9e5a393 jsonutils.load() accepts file pointer, not string - a6b2aec On Python <= 2.6, use simplejson if available - e3a1d9c Use six.moves.xmlrpc_client instead of xmlrpclib * strutils.py: - 8a0f567 Remove str() from LOG.* and exceptions - fd18c28 Fix safe_encode(): return bytes on Python 3 - 302c7c8 strutils: Allow safe_{encode,decode} to take bytes as input - bec3a5e Implements SI/IEC unit system conversion to bytes - e53fe85 strutils bool_from_string, allow specified default * timeutils.py: - 250cd88 Fixed a new pep8 error and a small typo Change-Id: Ib3dc0b713ed90396919feba018772243b3b9c90f Closes-Bug: 1314129
74 lines
2.3 KiB
Python
74 lines
2.3 KiB
Python
# Copyright 2011 OpenStack Foundation.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
Import related utilities and helper functions.
|
|
"""
|
|
|
|
import sys
|
|
import traceback
|
|
|
|
|
|
def import_class(import_str):
|
|
"""Returns a class from a string including module and class."""
|
|
mod_str, _sep, class_str = import_str.rpartition('.')
|
|
__import__(mod_str)
|
|
try:
|
|
return getattr(sys.modules[mod_str], class_str)
|
|
except AttributeError:
|
|
raise ImportError('Class %s cannot be found (%s)' %
|
|
(class_str,
|
|
traceback.format_exception(*sys.exc_info())))
|
|
|
|
|
|
def import_object(import_str, *args, **kwargs):
|
|
"""Import a class and return an instance of it."""
|
|
return import_class(import_str)(*args, **kwargs)
|
|
|
|
|
|
def import_object_ns(name_space, import_str, *args, **kwargs):
|
|
"""Tries to import object from default namespace.
|
|
|
|
Imports a class and return an instance of it, first by trying
|
|
to find the class in a default namespace, then failing back to
|
|
a full path if not found in the default namespace.
|
|
"""
|
|
import_value = "%s.%s" % (name_space, import_str)
|
|
try:
|
|
return import_class(import_value)(*args, **kwargs)
|
|
except ImportError:
|
|
return import_class(import_str)(*args, **kwargs)
|
|
|
|
|
|
def import_module(import_str):
|
|
"""Import a module."""
|
|
__import__(import_str)
|
|
return sys.modules[import_str]
|
|
|
|
|
|
def import_versioned_module(version, submodule=None):
|
|
module = 'glance.v%s' % version
|
|
if submodule:
|
|
module = '.'.join((module, submodule))
|
|
return import_module(module)
|
|
|
|
|
|
def try_import(import_str, default=None):
|
|
"""Try to import a module and if it fails return default."""
|
|
try:
|
|
return import_module(import_str)
|
|
except ImportError:
|
|
return default
|