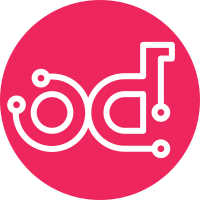
The changes here are substantial and widespread, but in summary: - We use cfg to parse the CLI and config files, rather than optparse and PasteDeploy - A schema is defined for all configuration options close to the code which uses the option - 2 ConfigOpts sub-classes are added to config.py basically just defining how to find config files; this means we can now use e.g. glance.conf for base config values which glance-api.conf can override - load_paste_app() is changed to load the paste app from the last config file in the stack and pass the app the ConfigOpts instance - The generic app and filter factories in wsgi.py are modified to pass a ConfigOpts instance to the apps and filters - A ConfigOpts subclass is added for the unit tests which writes out config values to a temporary config file and uses cfg to parse that I've tried to keep the switch as unobtrusive as possible leaving further cleanups for later e.g. - Moving PasteDeploy config out of the config files - I think it would be good to aim for having users modify the PasteDeploy config files only in fairly rare circumstances. To achieve this, we might define a number of common pipelines in the PasteDeploy config and allow the user to choose between those pipelines in the glance config. - We should add help strings to all the opts, even just for the sake of documenting them - We should move a bunch of the options into groups - e.g. all the rabbit options - We no longer rely on config files for default values, so the default config files could contain nothing but comments - i.e. explaining each option and showing what the default for it is - making it obvious where a user has explicitly set a value There are a couple of behavioural changes which I don't think are signifcant but are worth mentioning: - We used to support passing a config file as a positional argument but don't anymore; AFAICT, it was only used by glance-manage when launching servers and I've changed that to pass --config-file - log_opt_values() doesn't log unknown opts, so won't log any values for opts which get registered at runtime later Change-Id: Iafa998a2a8d860f1ad57e2cd2afee69686ed58ba
61 lines
2.1 KiB
Python
Executable File
61 lines
2.1 KiB
Python
Executable File
#!/usr/bin/env python
|
|
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright 2010 United States Government as represented by the
|
|
# Administrator of the National Aeronautics and Space Administration.
|
|
# Copyright 2011 OpenStack LLC.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
Glance Image Cache Invalid Cache Entry and Stalled Image cleaner
|
|
|
|
This is meant to be run as a periodic task from cron.
|
|
|
|
If something goes wrong while we're caching an image (for example the fetch
|
|
times out, or an exception is raised), we create an 'invalid' entry. These
|
|
entires are left around for debugging purposes. However, after some period of
|
|
time, we want to clean these up.
|
|
|
|
Also, if an incomplete image hangs around past the image_cache_stall_time
|
|
period, we automatically sweep it up.
|
|
"""
|
|
|
|
import gettext
|
|
import os
|
|
import sys
|
|
|
|
# If ../glance/__init__.py exists, add ../ to Python search path, so that
|
|
# it will override what happens to be installed in /usr/(local/)lib/python...
|
|
possible_topdir = os.path.normpath(os.path.join(os.path.abspath(sys.argv[0]),
|
|
os.pardir,
|
|
os.pardir))
|
|
if os.path.exists(os.path.join(possible_topdir, 'glance', '__init__.py')):
|
|
sys.path.insert(0, possible_topdir)
|
|
|
|
gettext.install('glance', unicode=1)
|
|
|
|
from glance.common import config
|
|
|
|
|
|
if __name__ == '__main__':
|
|
try:
|
|
conf = config.GlanceCacheConfigOpts()
|
|
conf()
|
|
|
|
app = config.load_paste_app(conf, 'glance-cleaner')
|
|
app.run()
|
|
except RuntimeError, e:
|
|
sys.exit("ERROR: %s" % e)
|