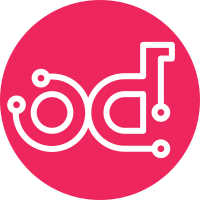
Use error code 2 to indicate that we are exiting a program without doing any work. This leaves error code 1 to mean that the program actually had an error, and we can check for those errors and report them. Add --debug and -v flag where missing in calls to python3-first app. Change-Id: Ia6f39dc8eee3505ac573670a9e44ae225ed48334 Signed-off-by: Doug Hellmann <doug@doughellmann.com>
117 lines
2.6 KiB
Bash
Executable File
117 lines
2.6 KiB
Bash
Executable File
#!/bin/bash -e
|
|
|
|
bindir=$(dirname $0)
|
|
source $bindir/functions
|
|
|
|
echo $0 $*
|
|
echo
|
|
|
|
function usage {
|
|
echo "do_repo.sh REPO_DIR BRANCH TASK"
|
|
}
|
|
|
|
repo="$1"
|
|
branch="$2"
|
|
task="$3"
|
|
|
|
if [ -z "$repo" ]; then
|
|
usage
|
|
echo "Need to specify a repo!"
|
|
exit 1
|
|
fi
|
|
|
|
if [ -z "$branch" ]; then
|
|
usage
|
|
echo "Need to specify a branch!"
|
|
exit 1
|
|
fi
|
|
|
|
if [ -z "$task" ]; then
|
|
usage
|
|
echo "Need to specify a task!"
|
|
exit 1
|
|
fi
|
|
|
|
commit_message="import zuul job settings from project-config
|
|
|
|
This is a mechanically generated patch to complete step 1 of moving
|
|
the zuul job settings out of project-config and into each project
|
|
repository.
|
|
|
|
Because there will be a separate patch on each branch, the branch
|
|
specifiers for branch-specific jobs have been removed.
|
|
|
|
Because this patch is generated by a script, there may be some
|
|
cosmetic changes to the layout of the YAML file(s) as the contents are
|
|
normalized.
|
|
|
|
See the python3-first goal document for details:
|
|
https://governance.openstack.org/tc/goals/stein/python3-first.html
|
|
|
|
Story: #2002586
|
|
Task: #$task
|
|
|
|
"
|
|
|
|
enable_tox
|
|
|
|
set -x
|
|
|
|
# NOTE(dhellmann): "git review -s" will just hang waiting for input if
|
|
# there is a problem setting up the gerrit remote. One cause of such
|
|
# trouble is a mis-match between the project setting within the
|
|
# .gitreview file and the actual repo name.
|
|
actual=$(cat $repo/.gitreview | grep project | cut -f2 -d=)
|
|
expected="$(basename $(dirname $repo))/$(basename $repo)"
|
|
if [ "$actual" != "$expected" -a "$actual" != "${expected}.git" ]; then
|
|
echo "WARNING: git review -s is likely to fail for $expected because .gitreview says $actual"
|
|
else
|
|
git -C "$repo" review -s
|
|
fi
|
|
|
|
new_branch=python3-first-$(basename $branch)
|
|
|
|
if git -C "$repo" branch | grep -q $new_branch; then
|
|
echo "$new_branch already exists, reusing"
|
|
git -C "$repo" checkout $new_branch
|
|
else
|
|
echo "creating $new_branch"
|
|
git -C "$repo" checkout -- .
|
|
git -C "$repo" clean -f -d
|
|
|
|
if ! git -C "$repo" checkout -q origin/$branch ; then
|
|
echo "Could not check out origin/$branch in $repo"
|
|
exit 2
|
|
fi
|
|
|
|
git -C "$repo" checkout -b $new_branch
|
|
fi
|
|
|
|
|
|
python3-first -v --debug jobs update "$repo"
|
|
RC=$?
|
|
if [ $RC -eq 2 ]; then
|
|
echo "No changes"
|
|
exit 1
|
|
fi
|
|
if [ $RC -ne 0 ]; then
|
|
echo "FAIL"
|
|
exit $RC
|
|
fi
|
|
|
|
if ! git -C "$repo" diff --ignore-all-space; then
|
|
echo "No changes other than whitespace"
|
|
git -C "$repo" checkout -- .
|
|
exit 1
|
|
fi
|
|
|
|
git -C "$repo" add .
|
|
# NOTE(dhellmann): Some repositories have '.*' excluded by default so
|
|
# adding a new file requires a force flag.
|
|
if [ -f "$repo/.zuul.yaml" ]; then
|
|
git -C "$repo" add -f .zuul.yaml
|
|
fi
|
|
git -C "$repo" commit -m "$commit_message"
|
|
|
|
git -C "$repo" show
|