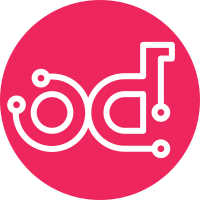
There are some deprecated commands being used in the javelin setup they should be removed since we are removing all the deprecated glance client commands in this patch http://review.openstack.org/#/c/108804/ Change-Id: Ieb05c7ba2959c26c987667dba751e94b899772af
151 lines
4.6 KiB
Bash
Executable File
151 lines
4.6 KiB
Bash
Executable File
#!/usr/bin/env bash
|
|
|
|
# ``setup-javelin``
|
|
|
|
# Keep track of the grenade directory
|
|
GRENADE_DIR=$(cd $(dirname "$0") && pwd)
|
|
|
|
# Import common functions
|
|
source $GRENADE_DIR/functions
|
|
|
|
# Determine what system we are running on. This provides ``os_VENDOR``,
|
|
# ``os_RELEASE``, ``os_UPDATE``, ``os_PACKAGE``, ``os_CODENAME``
|
|
# and ``DISTRO``
|
|
GetDistro
|
|
|
|
# Source params
|
|
source $GRENADE_DIR/grenaderc
|
|
|
|
# For debugging
|
|
set -o xtrace
|
|
|
|
|
|
# Create Javelin Project
|
|
# ======================
|
|
|
|
# Get DevStack Configuration
|
|
source $BASE_DEVSTACK_DIR/openrc admin admin
|
|
|
|
# Our configuration
|
|
source $GRENADE_DIR/javelin.conf
|
|
|
|
DEFAULT_INSTANCE_TYPE=${DEFAULT_INSTANCE_TYPE:-m1.tiny}
|
|
|
|
|
|
# Add To Keystone
|
|
# ---------------
|
|
|
|
function get_id () {
|
|
echo `"$@" | awk '/ id / { print $4 }'`
|
|
}
|
|
|
|
# Create javelin project and user
|
|
JAVELIN_TENANT=$(keystone tenant-list | awk "/ $JPROJECT / { print \$2 }")
|
|
[[ -z "$JAVELIN_TENANT" ]] && \
|
|
JAVELIN_TENANT=$(get_id keystone tenant-create --name=$JPROJECT)
|
|
|
|
JAVELIN_USER=$(keystone user-list | awk "/ $JUSER / { print \$2 }")
|
|
[[ -z "$JAVELIN_USER" ]] && \
|
|
JAVELIN_USER=$(get_id keystone user-create --name=$JUSER \
|
|
--pass="$JPASSWORD" \
|
|
--email=bob@javelin.org)
|
|
|
|
MEMBER_ROLE=$(keystone role-list | awk "/ Member / { print \$2 }")
|
|
keystone user-role-add --tenant_id $JAVELIN_TENANT \
|
|
--user_id $JAVELIN_USER \
|
|
--role_id $MEMBER_ROLE
|
|
|
|
|
|
# Switch Identities
|
|
# -----------------
|
|
|
|
source $BASE_DEVSTACK_DIR/openrc $JPROJECT $JUSER
|
|
export OS_PASSWORD=$JPASSWORD
|
|
|
|
|
|
# Add Custom Image
|
|
# ----------------
|
|
|
|
# It's really cirros with our own name
|
|
# Assumes DevStack.$BASE has already set this up
|
|
CIRROS_IMAGE=${DEFAULT_IMAGE_NAME:-cirros-0.3.2-x86_64-uec}
|
|
CIRROS_DIR=$BASE_DEVSTACK_DIR/files/images/$CIRROS_IMAGE
|
|
KERNEL=$CIRROS_DIR/${CIRROS_IMAGE/uec/}vmlinuz
|
|
RAMDISK=$CIRROS_DIR/${CIRROS_IMAGE/uec/}initrd
|
|
ROOTDISK=$CIRROS_DIR/${CIRROS_IMAGE/uec/}blank.img
|
|
JAVELIN_IMAGE=${CIRROS_IMAGE/cirros/javelin}
|
|
IMAGE_ID=$(cat /proc/sys/kernel/random/uuid)
|
|
|
|
IMAGE=$(nova image-list | awk "/ $JAVELIN_IMAGE.img / { print \$2 }")
|
|
if [[ -z "$IMAGE" ]]; then
|
|
glance image-create --name $JAVELIN_IMAGE-kernel --is-public True --container-format aki --disk-format aki < "$KERNEL_ID"
|
|
glance image-create --name $JAVELIN_IMAGE-ramdisk --is-public True --container-format ari --disk-format ari < "$RAMDISK_ID"
|
|
glance image-create --name $JAVELIN_IMAGE.img --is-public True --id $IMAGE_ID --container-format ami --disk-format ami --property kernel_id=$KERNEL_ID --property ramdisk_id=$RAMDISK_ID < "${ROOTDISK}"
|
|
|
|
IMAGE=$IMAGE_ID
|
|
die_if_not_set $LINENO IMAGE "Failure getting image"
|
|
fi
|
|
|
|
|
|
# Add Custom Security Group
|
|
# -------------------------
|
|
|
|
# Create our secgroup
|
|
if ! nova secgroup-list | grep -q $JAVELIN_SECGROUP; then
|
|
nova secgroup-create $JAVELIN_SECGROUP "$JPROJECT access rules"
|
|
if ! timeout $ACTIVE_TIMEOUT sh -c "while ! nova secgroup-list | grep -q $JAVELIN_SECGROUP; do sleep 1; done"; then
|
|
die $LINENO "$JAVELIN_SECGROUP security group not created"
|
|
fi
|
|
fi
|
|
|
|
# Add some rules
|
|
if ! nova secgroup-list-rules $JAVELIN_SECGROUP | grep tcp | grep -q 22; then
|
|
nova secgroup-add-rule $JAVELIN_SECGROUP icmp -1 -1 0.0.0.0/0
|
|
nova secgroup-add-rule $JAVELIN_SECGROUP tcp 22 22 0.0.0.0/0
|
|
fi
|
|
|
|
|
|
# Identify A Flavor
|
|
# -----------------
|
|
|
|
FLAVOR=`nova flavor-list | grep $DEFAULT_INSTANCE_TYPE | get_field 1`
|
|
if [[ -z "$FLAVOR" ]]; then
|
|
# Grab the first flavor in the list to launch if default doesn't exist
|
|
FLAVOR=`nova flavor-list | head -n 4 | tail -n 1 | get_field 1`
|
|
fi
|
|
|
|
|
|
# Create a Volume
|
|
# ---------------
|
|
|
|
if ! nova volume-list | grep -q $JAVELIN_VOLUME; then
|
|
nova volume-create --display_name=$JAVELIN_VOLUME 1
|
|
if ! timeout $ACTIVE_TIMEOUT sh -c "while ! nova volume-list | grep $JAVELIN_VOLUME | grep -q available; do sleep 1; done"; then
|
|
die $LINENO "$JAVELIN_VOLUME volume not created"
|
|
fi
|
|
fi
|
|
|
|
# Create An Instance
|
|
# ------------------
|
|
|
|
# Boot an instance
|
|
BOOT_TXT=$(nova boot --flavor "$FLAVOR" --image "$IMAGE" $JSERVER | awk "/adminPass/ { print \$2 \"=\" \$4 };/ id / { print \$2 \"=\" \$4 }"; exit ${PIPESTATUS[0]})
|
|
ret=$?
|
|
if [[ ! $ret = 0 ]]; then
|
|
die $LINENO "Failed to boot $JSERVER"
|
|
fi
|
|
eval $BOOT_TXT
|
|
|
|
# Check that the status is active within ACTIVE_TIMEOUT seconds
|
|
if ! timeout $ACTIVE_TIMEOUT sh -c "while ! nova show $id | grep status | grep -q ACTIVE; do sleep 1; done"; then
|
|
die $LINENO "server didn't become active!"
|
|
fi
|
|
|
|
# Put some stuff in Swift
|
|
# -----------------------
|
|
|
|
swift upload javelin /etc/hosts
|
|
if ! swift list javelin | grep -q hosts; then
|
|
die $LINENO "Swift upload failed"
|
|
fi
|