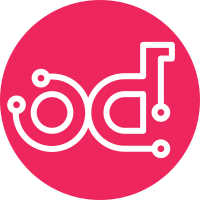
This adds a mapping for all process names described in BASE_SERVICES and TARGET_SERVICES, allowing check-sanity to skip checking for running processes of services that are disabled according to ENABLED_SERVICES. It also updates grenade.sh and save-state to only dump databases that exist. These changes allow grenade to pass against deployments that have disabled non-essential services in devstack. Change-Id: Ic4b2bab88b12f33f939b9bf7aca47e930d799f3f Closes-bug: #1355499
126 lines
3.6 KiB
Bash
Executable File
126 lines
3.6 KiB
Bash
Executable File
#!/usr/bin/env bash
|
|
|
|
# ``upgrade-sanity``
|
|
|
|
# this is a set of sanity checks that should be run after upgrade to make sure
|
|
# the environment actually looks like we expect, if not, die horribly
|
|
|
|
echo "*********************************************************************"
|
|
echo "Begin $0"
|
|
echo "*********************************************************************"
|
|
|
|
# Keep track of the devstack directory
|
|
GRENADE_DIR=$(cd $(dirname "$0") && pwd)
|
|
|
|
# Import common functions
|
|
source $GRENADE_DIR/functions
|
|
|
|
# Determine what system we are running on. This provides ``os_VENDOR``,
|
|
# ``os_RELEASE``, ``os_UPDATE``, ``os_PACKAGE``, ``os_CODENAME``
|
|
# and ``DISTRO``
|
|
GetDistro
|
|
|
|
# Source params
|
|
source $GRENADE_DIR/grenaderc
|
|
|
|
# We need the OS_ credentials
|
|
source $TARGET_DEVSTACK_DIR/openrc
|
|
source $TARGET_DEVSTACK_DIR/functions
|
|
source $TARGET_DEVSTACK_DIR/localrc
|
|
|
|
|
|
function is_a_service {
|
|
local name=$1
|
|
if [[ ! $name =~ '-' ]]; then
|
|
echo "$name does not look like a valid service, skipping log check"
|
|
return 1
|
|
fi
|
|
if [[ $name =~ ^ceilometer ]]; then
|
|
echo "Ceilometer not yet supported, skipping check for $name"
|
|
return 1
|
|
fi
|
|
if [[ $name =~ ^h- ]]; then
|
|
echo "Heat not yet supported, skipping check for $name"
|
|
return 1
|
|
fi
|
|
if [[ "${DO_NOT_UPGRADE_SERVICES}" =~ "${service}" ]]; then
|
|
echo "$service not upgraded, skipping check"
|
|
return 1
|
|
fi
|
|
if [ "$name" == "q-fwaas" ]; then
|
|
echo "$name is a Neutron service plugin and doesn't run in its own process, skipping check"
|
|
return 1
|
|
fi
|
|
if [ "$name" == "q-l3" ] && [[ "$ENABLED_SERVICES" =~ "q-vpn" ]]; then
|
|
echo "$name is running as a part of q-vpn service, skipping check"
|
|
return 1
|
|
fi
|
|
return 0
|
|
}
|
|
|
|
function test_all_enabled_services {
|
|
local tmpsvcs="${ENABLED_SERVICES}"
|
|
local service
|
|
local log
|
|
local failed=0
|
|
local not_running=""
|
|
for service in ${tmpsvcs//,/ }; do
|
|
if is_a_service $service; then
|
|
log=${SCREEN_LOGDIR}/screen-$service.log
|
|
if [[ ! -e $log ]]; then
|
|
echo "Couldn't find log for $service at $log"
|
|
not_running="$not_running,$service"
|
|
failed=1
|
|
fi
|
|
fi
|
|
done
|
|
|
|
if [[ $failed -eq 1 ]]; then
|
|
echo "Expected running services not running: $not_running"
|
|
exit 1
|
|
fi
|
|
}
|
|
|
|
# For a given process name, resolve the corresponding devstack service
|
|
# name as it would appear in ENABLED_SERVICES.
|
|
function process_to_service {
|
|
case "$1" in
|
|
"nova-api") echo "n-api" ;;
|
|
"nova-conductor") echo "n-cond" ;;
|
|
"nova-compute") echo "n-cpu" ;;
|
|
"keystone") echo "key" ;;
|
|
"glance-api") echo "g-api" ;;
|
|
"cinder-api") echo "c-api" ;;
|
|
"swift-object-server") echo "s-obj" ;;
|
|
"swift-proxy-server") echo "s-proxy" ;;
|
|
*) echo "$1" ;;
|
|
esac
|
|
}
|
|
|
|
test_all_enabled_services
|
|
|
|
# all the services should actually be running that we expect
|
|
NOT_RUNNING=""
|
|
RUNNING=""
|
|
for proc_name in ${TARGET_SERVICES}; do
|
|
if is_service_enabled "$(process_to_service $proc_name)" ; then
|
|
if ! is_running ${proc_name}; then
|
|
NOT_RUNNING="$NOT_RUNNING $proc_name"
|
|
else
|
|
RUNNING="$RUNNING $proc_name"
|
|
fi
|
|
fi
|
|
done
|
|
|
|
if [[ -n "$NOT_RUNNING" ]]; then
|
|
echo "The following services are not running after upgrade: $NOT_RUNNING"
|
|
$GRENADE_DIR/tools/worlddump.py -d $LOGDIR
|
|
exit 1
|
|
else
|
|
echo "the following services are running: $RUNNING"
|
|
fi
|
|
|
|
echo "*********************************************************************"
|
|
echo "SUCCESS: End $0"
|
|
echo "*********************************************************************"
|