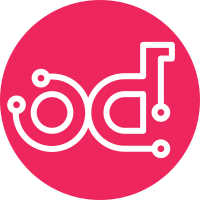
The current cfn-init software-config hook doesn't work at all since cfn_helper is installed in a venv, not in site-packages. This change switches to writing out metadata to /var/cache/heat-cfntools/last_metadata and invoking cfn-init. /var/lib/heat-cfntools/cfn-init-data cannot be used since that is already populated with all boot metadata, which may still be used by os-collect-config bootstrapping. Change-Id: I7252a6f12223613b55b4b6417383673faa0d52b3 Closes-Bug: #1321513
50 lines
1.5 KiB
Python
Executable File
50 lines
1.5 KiB
Python
Executable File
#!/usr/bin/env python
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
'''
|
|
A fake config tool for unit testing the software-config hooks.
|
|
|
|
JSON containing the current environment variables and command line arguments
|
|
are written to the file specified by the path in environment variable
|
|
TEST_STATE_PATH.
|
|
|
|
Environment variable TEST_RESPONSE defines JSON specifying what files to write
|
|
out, and what to print to stdout and stderr.
|
|
'''
|
|
|
|
import json
|
|
import os
|
|
import sys
|
|
|
|
|
|
def main(argv=sys.argv):
|
|
with open(os.environ.get('TEST_STATE_PATH'), 'w') as f:
|
|
json.dump({'env': dict(os.environ), 'args': argv}, f)
|
|
|
|
if 'TEST_RESPONSE' not in os.environ:
|
|
return
|
|
|
|
response = json.loads(os.environ.get('TEST_RESPONSE'))
|
|
for k, v in response.get('files', {}).iteritems():
|
|
open(k, 'w')
|
|
with open(k, 'w') as f:
|
|
f.write(v)
|
|
|
|
sys.stdout.write(response.get('stdout', ''))
|
|
sys.stderr.write(response.get('stderr', ''))
|
|
return response.get('returncode', 0)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
sys.exit(main(sys.argv))
|