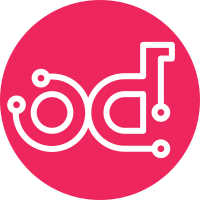
In mitaka, a new feature is introduced to ignore a given set of errors and is used here to fix the zuul faliure. In addition, it fixes the invalid template errors. For those templates which causes circular error is moved to invalid folder NOTE: openshift-origin/centos65/highly-available is marked as invald to make the build jobs to pass. depends-on: I4a3043fd17b69a346d447dfbd17488040cf9b387 Change-Id: I7b5b72cb8f6ff53b82edf92799a57917c718e032 Closes-bug: #1554380
53 lines
1.3 KiB
Python
Executable File
53 lines
1.3 KiB
Python
Executable File
#!/usr/bin/env python
|
|
|
|
import os
|
|
import re
|
|
import subprocess
|
|
import sys
|
|
|
|
|
|
EXCLUDED_DIRS = ('contrib', 'elements', 'invalid')
|
|
|
|
|
|
def main(args):
|
|
if len(args) != 1:
|
|
raise SystemExit("Takes one argument, the path to the templates")
|
|
|
|
path = args[0]
|
|
got_error = False
|
|
for root, dirs, files in os.walk(path):
|
|
for excluded in EXCLUDED_DIRS:
|
|
if excluded in dirs:
|
|
dirs.remove(excluded)
|
|
for name in files:
|
|
if name.endswith((".yaml", ".template")):
|
|
got_error = validate(root, name) or got_error
|
|
sys.exit(int(got_error))
|
|
|
|
|
|
def validate(base, name):
|
|
basename, ext = os.path.splitext(name)
|
|
if basename.endswith("_env"):
|
|
return False
|
|
args = ["heat",
|
|
"template-validate",
|
|
"-f",
|
|
os.path.join(base, name),
|
|
"--ignore-errors",
|
|
'99001']
|
|
base_env = "%s_env%s" % (basename, ext)
|
|
env = os.path.join(base, base_env)
|
|
if os.path.exists(env):
|
|
args.extend(["-e", env])
|
|
try:
|
|
subprocess.check_output(args, stderr=subprocess.STDOUT)
|
|
except subprocess.CalledProcessError as e:
|
|
print "Got error validating %s/%s , %s" % (base, name, e.output)
|
|
return True
|
|
else:
|
|
return False
|
|
|
|
|
|
if __name__ == '__main__':
|
|
main(sys.argv[1:])
|