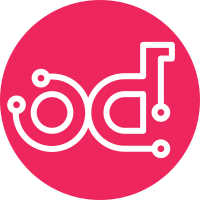
Currently, the patch does the following: Kicks off workflow from stack.update_or_create: Once the dependency graph is calculated, the leaves of the graph are all casted into the RPC worker bus. Worker RPC check_resource worfklow: Workers will then start working on each resource individually. Once a resource operation is finished, sync points are used to check if the parent resource can be worked on. Resources that finish early will wait for their siblings to finish. The sibling that finishes last will trigger the creation/updation/deletion of it's parent. This process then goes on for all nodes until the roots of the graph are processed. Marks stack as complete when roots have finished. Once the roots of the graph are successfully processed, the previous raw template which was needed for rollback in case something went wrong will now be deleted from the database. The stack is then marked as complete. Largely follows the convergence prototype code in github.com/zaneb/heat-convergence-prototype/blob/resumable/converge/converger.py Implements blueprint convergence-check-workflow Change-Id: I67cfdc452ba406198c96afba57aa4e756408105d
58 lines
1.7 KiB
Python
58 lines
1.7 KiB
Python
# Copyright (c) 2014 Hewlett-Packard Development Company, L.P.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
|
|
# implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
"""
|
|
Client side of the heat worker RPC API.
|
|
"""
|
|
|
|
from heat.common import messaging
|
|
from heat.rpc import worker_api
|
|
|
|
|
|
class WorkerClient(object):
|
|
'''Client side of the heat worker RPC API.
|
|
|
|
API version history::
|
|
|
|
1.0 - Initial version.
|
|
1.1 - Added check_resource.
|
|
'''
|
|
|
|
BASE_RPC_API_VERSION = '1.0'
|
|
|
|
def __init__(self):
|
|
self._client = messaging.get_rpc_client(
|
|
topic=worker_api.TOPIC,
|
|
version=self.BASE_RPC_API_VERSION)
|
|
|
|
@staticmethod
|
|
def make_msg(method, **kwargs):
|
|
return method, kwargs
|
|
|
|
def cast(self, ctxt, msg, version=None):
|
|
method, kwargs = msg
|
|
if version is not None:
|
|
client = self._client.prepare(version=version)
|
|
else:
|
|
client = self._client
|
|
client.cast(ctxt, method, **kwargs)
|
|
|
|
def check_resource(self, ctxt, resource_id,
|
|
current_traversal, data, is_update):
|
|
self.cast(ctxt, self.make_msg(
|
|
'check_resource', resource_id=resource_id,
|
|
current_traversal=current_traversal, data=data,
|
|
is_update=is_update))
|