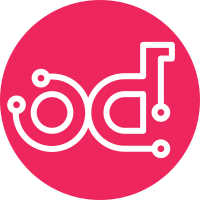
On this panel, aggregates could be added, deleted and edited. This patch takes the aggregates panel out of System Info and puts it back in the main admin panel list, as now, aggregates are not static information. The host can be associated to a host aggregate on this panel as well. Change-Id: I4ef2d87c33981db36d4ebd3de2f4841cdfa9dbfd Closes-Bug: #1261932 Implements: blueprint manage-host-aggregates Co-Authored-By: Santiago Baldassin <santiago.b.baldassin@intel.com> Co-Authored-By: Alejandro Paredes <alejandro.e.paredes@intel.com>
147 lines
4.7 KiB
Python
147 lines
4.7 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from django import template
|
|
from django.template import defaultfilters as filters
|
|
from django.utils.translation import ugettext_lazy as _
|
|
|
|
from horizon import tables
|
|
from horizon.utils import filters as utils_filters
|
|
|
|
|
|
class ServiceFilterAction(tables.FilterAction):
|
|
def filter(self, table, services, filter_string):
|
|
q = filter_string.lower()
|
|
|
|
def comp(service):
|
|
if q in service.type.lower():
|
|
return True
|
|
return False
|
|
|
|
return filter(comp, services)
|
|
|
|
|
|
def get_stats(service):
|
|
return template.loader.render_to_string('admin/services/_stats.html',
|
|
{'service': service})
|
|
|
|
|
|
def get_enabled(service, reverse=False):
|
|
options = ["Enabled", "Disabled"]
|
|
if reverse:
|
|
options.reverse()
|
|
# if not configured in this region, neither option makes sense
|
|
if service.host:
|
|
return options[0] if not service.disabled else options[1]
|
|
return None
|
|
|
|
|
|
class ServicesTable(tables.DataTable):
|
|
id = tables.Column('id', verbose_name=_('Id'), hidden=True)
|
|
name = tables.Column("name", verbose_name=_('Name'))
|
|
service_type = tables.Column('__unicode__', verbose_name=_('Service'))
|
|
host = tables.Column('host', verbose_name=_('Host'))
|
|
enabled = tables.Column(get_enabled,
|
|
verbose_name=_('Enabled'),
|
|
status=True)
|
|
|
|
class Meta:
|
|
name = "services"
|
|
verbose_name = _("Services")
|
|
table_actions = (ServiceFilterAction,)
|
|
multi_select = False
|
|
status_columns = ["enabled"]
|
|
|
|
|
|
def get_available(zone):
|
|
return zone.zoneState['available']
|
|
|
|
|
|
class NovaServiceFilterAction(tables.FilterAction):
|
|
def filter(self, table, services, filter_string):
|
|
q = filter_string.lower()
|
|
|
|
def comp(service):
|
|
if q in service.type.lower():
|
|
return True
|
|
return False
|
|
|
|
return filter(comp, services)
|
|
|
|
|
|
class NovaServicesTable(tables.DataTable):
|
|
binary = tables.Column("binary", verbose_name=_('Name'))
|
|
host = tables.Column('host', verbose_name=_('Host'))
|
|
zone = tables.Column('zone', verbose_name=_('Zone'))
|
|
status = tables.Column('status', verbose_name=_('Status'))
|
|
state = tables.Column('state', verbose_name=_('State'))
|
|
updated_at = tables.Column('updated_at',
|
|
verbose_name=_('Updated At'),
|
|
filters=(utils_filters.parse_isotime,
|
|
filters.timesince))
|
|
|
|
def get_object_id(self, obj):
|
|
return "%s-%s-%s" % (obj.binary, obj.host, obj.zone)
|
|
|
|
class Meta:
|
|
name = "nova_services"
|
|
verbose_name = _("Compute Services")
|
|
table_actions = (NovaServiceFilterAction,)
|
|
multi_select = False
|
|
|
|
|
|
class NetworkAgentsFilterAction(tables.FilterAction):
|
|
def filter(self, table, agents, filter_string):
|
|
q = filter_string.lower()
|
|
|
|
def comp(agent):
|
|
if q in agent.agent_type.lower():
|
|
return True
|
|
return False
|
|
|
|
return filter(comp, agents)
|
|
|
|
|
|
def get_network_agent_status(agent):
|
|
if agent.admin_state_up:
|
|
return _('Enabled')
|
|
|
|
return _('Disabled')
|
|
|
|
|
|
def get_network_agent_state(agent):
|
|
if agent.alive:
|
|
return _('Up')
|
|
|
|
return _('Down')
|
|
|
|
|
|
class NetworkAgentsTable(tables.DataTable):
|
|
agent_type = tables.Column('agent_type', verbose_name=_('Type'))
|
|
binary = tables.Column("binary", verbose_name=_('Name'))
|
|
host = tables.Column('host', verbose_name=_('Host'))
|
|
status = tables.Column(get_network_agent_status, verbose_name=_('Status'))
|
|
state = tables.Column(get_network_agent_state, verbose_name=_('State'))
|
|
heartbeat_timestamp = tables.Column('heartbeat_timestamp',
|
|
verbose_name=_('Updated At'),
|
|
filters=(utils_filters.parse_isotime,
|
|
filters.timesince))
|
|
|
|
def get_object_id(self, obj):
|
|
return "%s-%s" % (obj.binary, obj.host)
|
|
|
|
class Meta:
|
|
name = "network_agents"
|
|
verbose_name = _("Network Agents")
|
|
table_actions = (NetworkAgentsFilterAction,)
|
|
multi_select = False
|