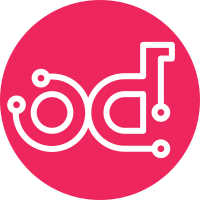
This patch adds the infrastructure needed to move the configuration of Horizon into oslo.config-compatible configuration file, instead of the Django's Python-based configuration. It doesn't actually define any configuration options, just the mechanism for loading them and the additional types necessary to handle Horizon's complex configuration, and the integration with oslo-config-generator. Subsequent patches will add groups of options, making it possible to use them in the local_settings.conf file instead of the local_settings.py file. Note, that the options specified in the local_settings.py file will continue to work. Partially-Implements: blueprint ini-based-configuration Change-Id: I2ed79ef0c6ac6d3816bba13346b7d001c46a7e80
69 lines
2.2 KiB
Python
69 lines
2.2 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
This module contains utility functions for loading Horizon's
|
|
configuration from .ini files using the oslo.config library.
|
|
"""
|
|
|
|
import six
|
|
|
|
from oslo_config import cfg
|
|
|
|
# XXX import the actual config groups here
|
|
# from openstack_dashboard.config import config_compress
|
|
|
|
|
|
def load_config(files=None, root_path=None, local_path=None):
|
|
"""Load the configuration from specified files."""
|
|
|
|
config = cfg.ConfigOpts()
|
|
config.register_opts([
|
|
cfg.Opt('root_path', default=root_path),
|
|
cfg.Opt('local_path', default=local_path),
|
|
])
|
|
# XXX register actual config groups here
|
|
# theme_group = config_theme.register_config(config)
|
|
if files is not None:
|
|
config(args=[], default_config_files=files)
|
|
return config
|
|
|
|
|
|
def apply_config(config, target):
|
|
"""Apply the configuration on the specified settings module."""
|
|
# TODO(rdopiera) fill with actual config groups
|
|
# apply_config_group(config.email, target, 'email')
|
|
|
|
|
|
def apply_config_group(config_group, target, prefix=None):
|
|
for key, value in six.iteritems(config_group):
|
|
name = key.upper()
|
|
if prefix:
|
|
name = '_'.join([prefix.upper(), name])
|
|
target[name] = value
|
|
|
|
|
|
def list_options():
|
|
# This is a really nasty hack to make the translatable strings
|
|
# work without having to initialize Django and read all the settings.
|
|
from django.apps import registry
|
|
from django.conf import settings
|
|
|
|
settings.configure()
|
|
registry.apps.check_apps_ready = lambda: True
|
|
|
|
config = load_config()
|
|
return [
|
|
(name, [d['opt'] for d in group._opts.values()])
|
|
for (name, group) in config._groups.items()
|
|
]
|