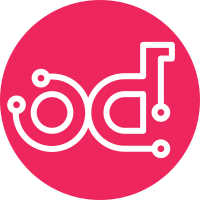
'priority' attribute has be handled in drawer [1][2], but the behavior is same as in rows. The behavior is that the items which have lower priority are hidden from both of row and drawer when wid$ Drawer is used for the supplements against the row, so it should be handled conversely. It means that items appear in drawer when they are hidden from row. This patch fixes it. [1] https://bugs.launchpad.net/horizon/+bug/1648332 [2] https://review.openstack.org/#/c/397132/ Change-Id: Ia0487a1d95ba16d764546cdb374fe786a0f0f648 Closes-Bug: #1651658
90 lines
3.3 KiB
JavaScript
90 lines
3.3 KiB
JavaScript
/*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
(function() {
|
|
'use strict';
|
|
|
|
describe('hz-resource-property directive', function() {
|
|
var $compile, $rootScope, $scope, registry, tableColumnInfo;
|
|
var testResource = {
|
|
getProperties: function() {
|
|
return {name: {label: 'display name'}};
|
|
},
|
|
getTableColumns: function() {
|
|
return tableColumnInfo;
|
|
}
|
|
};
|
|
|
|
beforeEach(module('templates'));
|
|
beforeEach(module('horizon.framework'));
|
|
|
|
beforeEach(inject(function(_$compile_, _$rootScope_, $injector) {
|
|
registry = $injector.get('horizon.framework.conf.resource-type-registry.service');
|
|
$compile = _$compile_;
|
|
$rootScope = _$rootScope_;
|
|
$scope = $rootScope.$new();
|
|
}));
|
|
|
|
it("sets class when item's priority is set 1", function() {
|
|
$scope.item = {name: 'value of name'};
|
|
tableColumnInfo = [{id: 'name', priority: 1 }];
|
|
spyOn(registry, 'getResourceType').and.returnValue(testResource);
|
|
var element = $compile(
|
|
"<hz-resource-property" +
|
|
" resource-type-name='resourceTypeName' item='item' prop-name='name'>" +
|
|
"</hz-resource-property>")($scope);
|
|
$scope.$digest();
|
|
expect(element.hasClass("rsp-p1")).toBeTruthy();
|
|
});
|
|
|
|
it("sets class when item's priority is set 2", function() {
|
|
$scope.item = {name: 'value of name'};
|
|
tableColumnInfo = [{id: 'name', priority: 2 }];
|
|
spyOn(registry, 'getResourceType').and.returnValue(testResource);
|
|
var element = $compile(
|
|
"<hz-resource-property" +
|
|
" resource-type-name='resourceTypeName' item='item' prop-name='name'>" +
|
|
"</hz-resource-property>")($scope);
|
|
$scope.$digest();
|
|
expect(element.hasClass("rsp-alt-p2")).toBeTruthy();
|
|
});
|
|
|
|
it("sets class when item's priority is set illegally", function() {
|
|
$scope.item = {name: 'value of name'};
|
|
tableColumnInfo = [{id: 'name', priority: 0 }];
|
|
spyOn(registry, 'getResourceType').and.returnValue(testResource);
|
|
var element = $compile(
|
|
"<hz-resource-property" +
|
|
" resource-type-name='resourceTypeName' item='item' prop-name='name'>" +
|
|
"</hz-resource-property>")($scope);
|
|
$scope.$digest();
|
|
expect(element.hasClass("rsp-p1")).toBeTruthy();
|
|
});
|
|
|
|
it("sets class when item's priority is not set", function() {
|
|
$scope.item = {name: 'value of name'};
|
|
// table column doesn't have an attribute of 'priority'
|
|
tableColumnInfo = [{id: 'name'}];
|
|
spyOn(registry, 'getResourceType').and.returnValue(testResource);
|
|
var element = $compile(
|
|
"<hz-resource-property" +
|
|
" resource-type-name='resourceTypeName' item='item' prop-name='name'>" +
|
|
"</hz-resource-property>")($scope);
|
|
$scope.$digest();
|
|
expect(element.hasClass("rsp-p1")).toBeTruthy();
|
|
});
|
|
});
|
|
|
|
})();
|