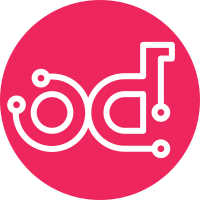
Based on keystone commit cfbc2aa30b7406b4bc77e40a55561d1f46174b5c keystone uses policy-in-code now, so there is a lot of differences. The new file was generated by oslopolicy-sample-generator. Sorted version diff is http://paste.openstack.org/show/628745/. Removed policies are: default identity:change_password identity:get_identity_providers 'identity:change_password' is used in horizon. There seems no corresponding new policy, so the corresponding horizon rules are dropped in this commit. Our UT depends on the identity policy file. This commit updates the UTs in a more robust way. Change-Id: I76eb9f95c7112bcbad75ee151f363f892298d081
135 lines
5.7 KiB
Python
135 lines
5.7 KiB
Python
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from django.test.utils import override_settings
|
|
from openstack_auth import policy as policy_backend
|
|
|
|
from openstack_dashboard import policy
|
|
from openstack_dashboard.test import helpers as test
|
|
|
|
|
|
class PolicyTestCase(test.TestCase):
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_policy_check_set(self):
|
|
value = policy.check((("identity", "admin_required"),),
|
|
request=self.request)
|
|
self.assertFalse(value)
|
|
|
|
@override_settings(POLICY_CHECK_FUNCTION=None)
|
|
def test_policy_check_not_set(self):
|
|
value = policy.check((("identity", "admin_required"),),
|
|
request=self.request)
|
|
self.assertTrue(value)
|
|
|
|
|
|
class PolicyBackendTestCase(test.TestCase):
|
|
def test_policy_file_load(self):
|
|
policy_backend.reset()
|
|
enforcer = policy_backend._get_enforcer()
|
|
self.assertEqual(2, len(enforcer))
|
|
self.assertIn('identity', enforcer)
|
|
self.assertIn('compute', enforcer)
|
|
|
|
def test_policy_reset(self):
|
|
policy_backend._get_enforcer()
|
|
self.assertEqual(2, len(policy_backend._ENFORCER))
|
|
policy_backend.reset()
|
|
self.assertIsNone(policy_backend._ENFORCER)
|
|
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_check_admin_required_false(self):
|
|
policy_backend.reset()
|
|
value = policy.check((("identity", "admin_required"),),
|
|
request=self.request)
|
|
self.assertFalse(value)
|
|
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_check_identity_rule_not_found_false(self):
|
|
policy_backend.reset()
|
|
value = policy.check((("identity", "i_dont_exist"),),
|
|
request=self.request)
|
|
# this should succeed because the default check does not exist and
|
|
# if the default check does not exist the policy check should succeed.
|
|
self.assertTrue(value)
|
|
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_check_nova_context_is_admin_false(self):
|
|
policy_backend.reset()
|
|
value = policy.check((("compute", "context_is_admin"),),
|
|
request=self.request)
|
|
self.assertFalse(value)
|
|
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_compound_check_false(self):
|
|
policy_backend.reset()
|
|
value = policy.check((("identity", "admin_required"),
|
|
("identity", "identity:default"),),
|
|
request=self.request)
|
|
self.assertFalse(value)
|
|
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_scope_not_found(self):
|
|
policy_backend.reset()
|
|
value = policy.check((("dummy", "default"),),
|
|
request=self.request)
|
|
self.assertTrue(value)
|
|
|
|
|
|
class PolicyBackendTestCaseAdmin(test.BaseAdminViewTests):
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_check_admin_required_true(self):
|
|
policy_backend.reset()
|
|
value = policy.check((("identity", "admin_required"),),
|
|
request=self.request)
|
|
self.assertTrue(value)
|
|
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_check_identity_rule_not_found_true(self):
|
|
policy_backend.reset()
|
|
value = policy.check((("identity", "i_dont_exist"),),
|
|
request=self.request)
|
|
# This assume the identity policy file does not contain the default
|
|
# check. If both a specified rule and the default rule do not exist,
|
|
# the check should succeed.
|
|
self.assertTrue(value)
|
|
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_compound_check_true(self):
|
|
policy_backend.reset()
|
|
|
|
# Check a single rule works expectly
|
|
self.assertTrue(policy.check((("identity", "admin_required"),),
|
|
request=self.request))
|
|
self.assertTrue(policy.check((("identity", "owner"),),
|
|
request=self.request,
|
|
target={'user_id': 1}))
|
|
self.assertFalse(policy.check((("identity", "owner"),),
|
|
request=self.request,
|
|
target={'user_id': 2}))
|
|
|
|
self.assertTrue(
|
|
policy.check((("identity", "admin_required"),
|
|
("identity", "owner"),),
|
|
request=self.request, target={'user_id': 1}))
|
|
self.assertFalse(
|
|
policy.check((("identity", "admin_required"),
|
|
("identity", "owner"),),
|
|
request=self.request, target={'user_id': 2}))
|
|
|
|
@override_settings(POLICY_CHECK_FUNCTION='openstack_auth.policy.check')
|
|
def test_check_nova_context_is_admin_true(self):
|
|
policy_backend.reset()
|
|
value = policy.check((("compute", "context_is_admin"),),
|
|
request=self.request)
|
|
self.assertTrue(value)
|