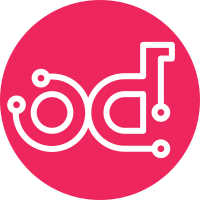
Create the base data abstraction layer into the DB in basic API service. I create a database table services as a example. This table is used to save the running status of deployed services. The command smaug-manage is used to manage the database. we can use this command 'smaug-manage db sync' to sync the smaug database up to the most recent version. smaug-manage version list: exposing the smaug codebase version. smaug-manage config list: exposing the configuration. smaug-manage service list: showing a list of all smaug services status. Change-Id: I2beeae0c0a7494a88bc4e4d143d36cee3f3f2a4e Closes-Bug: #1525794
121 lines
3.7 KiB
Python
121 lines
3.7 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""Defines interface for DB access.
|
|
|
|
Functions in this module are imported into the smaug.db namespace. Call these
|
|
functions from smaug.db namespace, not the smaug.db.api namespace.
|
|
|
|
All functions in this module return objects that implement a dictionary-like
|
|
interface. Currently, many of these objects are sqlalchemy objects that
|
|
implement a dictionary interface. However, a future goal is to have all of
|
|
these objects be simple dictionaries.
|
|
|
|
|
|
**Related Flags**
|
|
|
|
:connection: string specifying the sqlalchemy connection to use, like:
|
|
`sqlite:///var/lib/smaug/smaug.sqlite`.
|
|
|
|
:enable_new_services: when adding a new service to the database, is it in the
|
|
pool of available hardware (Default: True)
|
|
|
|
"""
|
|
|
|
from oslo_config import cfg
|
|
from oslo_db import concurrency as db_concurrency
|
|
from oslo_db import options as db_options
|
|
|
|
|
|
db_opts = [
|
|
cfg.BoolOpt('enable_new_services',
|
|
default=True,
|
|
help='Services to be added to the available pool on create'),
|
|
]
|
|
|
|
|
|
CONF = cfg.CONF
|
|
CONF.register_opts(db_opts)
|
|
db_options.set_defaults(CONF)
|
|
CONF.set_default('sqlite_db', 'smaug.sqlite', group='database')
|
|
|
|
_BACKEND_MAPPING = {'sqlalchemy': 'smaug.db.sqlalchemy.api'}
|
|
|
|
|
|
IMPL = db_concurrency.TpoolDbapiWrapper(CONF, _BACKEND_MAPPING)
|
|
|
|
# The maximum value a signed INT type may have
|
|
MAX_INT = 0x7FFFFFFF
|
|
|
|
|
|
###################
|
|
|
|
def dispose_engine():
|
|
"""Force the engine to establish new connections."""
|
|
|
|
# FIXME(jdg): When using sqlite if we do the dispose
|
|
# we seem to lose our DB here. Adding this check
|
|
# means we don't do the dispose, but we keep our sqlite DB
|
|
# This likely isn't the best way to handle this
|
|
|
|
if 'sqlite' not in IMPL.get_engine().name:
|
|
return IMPL.dispose_engine()
|
|
else:
|
|
return
|
|
|
|
|
|
###################
|
|
|
|
|
|
def service_destroy(context, service_id):
|
|
"""Destroy the service or raise if it does not exist."""
|
|
return IMPL.service_destroy(context, service_id)
|
|
|
|
|
|
def service_get(context, service_id):
|
|
"""Get a service or raise if it does not exist."""
|
|
return IMPL.service_get(context, service_id)
|
|
|
|
|
|
def service_get_by_host_and_topic(context, host, topic):
|
|
"""Get a service by host it's on and topic it listens to."""
|
|
return IMPL.service_get_by_host_and_topic(context, host, topic)
|
|
|
|
|
|
def service_get_all(context, disabled=None):
|
|
"""Get all services."""
|
|
return IMPL.service_get_all(context, disabled)
|
|
|
|
|
|
def service_get_all_by_topic(context, topic, disabled=None):
|
|
"""Get all services for a given topic."""
|
|
return IMPL.service_get_all_by_topic(context, topic, disabled=disabled)
|
|
|
|
|
|
def service_get_by_args(context, host, binary):
|
|
"""Get the state of an service by node name and binary."""
|
|
return IMPL.service_get_by_args(context, host, binary)
|
|
|
|
|
|
def service_create(context, values):
|
|
"""Create a service from the values dictionary."""
|
|
return IMPL.service_create(context, values)
|
|
|
|
|
|
def service_update(context, service_id, values):
|
|
"""Set the given properties on an service and update it.
|
|
|
|
Raises NotFound if service does not exist.
|
|
|
|
"""
|
|
return IMPL.service_update(context, service_id, values)
|