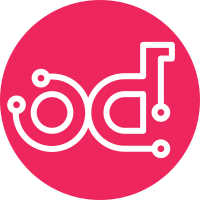
Protection plugin now needs to implement methods for each operation (protect, delete, restore). Each protection plugin is created in the context of the flow, while each operation is created in the context of the resource. A protection plugin is responsible for returning an Operation class for each operation. Such Operation class defines the behavior of the protection plugin by implementing these optional hooks: - on_prepare_begin hook is invoked before any hook of this resource and dependent resources has begun - on_prepare_finish hook is invoked after any prepare hooks of dependent resources are complete. - on_main hook is invoked after the resource prepare hooks are complete - on_complete hook is invoked once the resource's main hook is complete, and the dependent resources' on_complete hooks are complete HeatTemplate is now created and supplied from a task instead of being created and passed to resource tasks before the restore operation began. Change-Id: I847eec6990b2d24a66a12542d242fbfb682272fe Co-Authored-By: Saggi Mizrahi <saggi.mizrahi@huawei.com> Implements: blueprint protection-plugin-is-design
90 lines
3.5 KiB
Python
90 lines
3.5 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
|
|
from oslo_config import cfg
|
|
from oslo_log import log as logging
|
|
from oslo_utils import importutils
|
|
|
|
from karbor.common import constants
|
|
from karbor.i18n import _LE
|
|
from karbor.services.protection.flows import create_protection
|
|
from karbor.services.protection.flows import create_restoration
|
|
from karbor.services.protection.flows import delete_checkpoint
|
|
|
|
workflow_opts = [
|
|
cfg.StrOpt(
|
|
'workflow_engine',
|
|
default="karbor.services.protection.flows.workflow.TaskFlowEngine",
|
|
help='The workflow engine provides *flow* and *task* interface')
|
|
]
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
CONF = cfg.CONF
|
|
CONF.register_opts(workflow_opts)
|
|
|
|
|
|
class Worker(object):
|
|
def __init__(self, engine_path=None):
|
|
try:
|
|
self.workflow_engine = self._load_engine(engine_path)
|
|
except Exception:
|
|
LOG.error(_LE("load work flow engine failed"))
|
|
raise
|
|
|
|
def _load_engine(self, engine_path):
|
|
if not engine_path:
|
|
engine_path = CONF.workflow_engine
|
|
engine = importutils.import_object(engine_path)
|
|
return engine
|
|
|
|
def get_flow(self, context, operation_type, **kwargs):
|
|
if operation_type == constants.OPERATION_PROTECT:
|
|
plan = kwargs.get('plan', None)
|
|
provider = kwargs.get('provider', None)
|
|
checkpoint = kwargs.get('checkpoint')
|
|
protection_flow = create_protection.get_flow(context,
|
|
self.workflow_engine,
|
|
operation_type,
|
|
plan,
|
|
provider,
|
|
checkpoint)
|
|
return protection_flow
|
|
|
|
def get_restoration_flow(self, context, operation_type, checkpoint,
|
|
provider, restore, restore_auth):
|
|
restoration_flow = create_restoration.get_flow(context,
|
|
self.workflow_engine,
|
|
operation_type,
|
|
checkpoint,
|
|
provider,
|
|
restore,
|
|
restore_auth)
|
|
return restoration_flow
|
|
|
|
def get_delete_checkpoint_flow(self, context, operation_type, checkpoint,
|
|
provider):
|
|
delete_checkpoint_flow = delete_checkpoint.get_flow(
|
|
context,
|
|
self.workflow_engine,
|
|
operation_type,
|
|
checkpoint,
|
|
provider)
|
|
return delete_checkpoint_flow
|
|
|
|
def run_flow(self, flow_engine):
|
|
self.workflow_engine.run_engine(flow_engine)
|
|
|
|
def flow_outputs(self, flow_engine, target=None):
|
|
return self.workflow_engine.output(flow_engine, target=target)
|