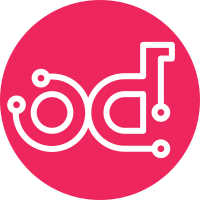
- Revised functional tests to expect auto-generated id - Revised manage.py to create users by name instead of id - Functional tests passing with User.id & User.name - Added User.id to Validate token response XSD/samples/python - Restored full scope of test discovery - Completely rewrote unit.test_users.py, because it needed some TLC - Added assertStatusCode to unit.test_users and refactored - Added assertContentType to unit.test_users and refactored - Abstracted out TestUpdateConflict - Coercing JSON responses to UTF-8, per charset - Added auto-generated PK to Tenant SQL model - Updated management API to add, list and disable tenants by name - Added get_by_name() to backend Tenant API - Implemented Tenant.get_by_name() for SQL - Simplified many of the "unit" tests further - Abstracted keystone resources and their REST verbs away from the keystone test client - Writing keystone client api for functional tests - Similar to the API "shortcuts" from the "unit" tests - Finished common FunctionalTestCase - Moved 'unit' tests over to the test.functional module... and rewrote them all - Cleaned up unnecessary debug output - Added pylint to pip-requires - Removed alterdb Change-Id: I45e689807166fc4f1cb59e385d2c7e680b36449d
69 lines
2.8 KiB
Python
69 lines
2.8 KiB
Python
from keystone import utils
|
|
from keystone.common import wsgi
|
|
import keystone.config as config
|
|
from keystone.logic.types.endpoint import EndpointTemplate
|
|
from . import get_url, get_marker_limit_and_url
|
|
|
|
|
|
class EndpointTemplatesController(wsgi.Controller):
|
|
"""Controller for EndpointTemplates related operations"""
|
|
|
|
def __init__(self, options):
|
|
self.options = options
|
|
|
|
@utils.wrap_error
|
|
def get_endpoint_templates(self, req):
|
|
marker, limit, url = get_marker_limit_and_url(req)
|
|
endpoint_templates = config.SERVICE.get_endpoint_templates(
|
|
utils.get_auth_token(req), marker, limit, url)
|
|
return utils.send_result(200, req, endpoint_templates)
|
|
|
|
@utils.wrap_error
|
|
def add_endpoint_template(self, req):
|
|
endpoint_template = utils.get_normalized_request_content(
|
|
EndpointTemplate, req)
|
|
return utils.send_result(201, req,
|
|
config.SERVICE.add_endpoint_template(utils.get_auth_token(req),
|
|
endpoint_template))
|
|
|
|
@utils.wrap_error
|
|
def modify_endpoint_template(self, req, endpoint_template_id):
|
|
endpoint_template = utils.\
|
|
get_normalized_request_content(EndpointTemplate, req)
|
|
return utils.send_result(201, req,
|
|
config.SERVICE.modify_endpoint_template(\
|
|
utils.get_auth_token(req),
|
|
endpoint_template_id, endpoint_template))
|
|
|
|
@utils.wrap_error
|
|
def delete_endpoint_template(self, req, endpoint_template_id):
|
|
rval = config.SERVICE.delete_endpoint_template(
|
|
utils.get_auth_token(req), endpoint_template_id)
|
|
return utils.send_result(204, req, rval)
|
|
|
|
@utils.wrap_error
|
|
def get_endpoint_template(self, req, endpoint_template_id):
|
|
endpoint_template = config.SERVICE.get_endpoint_template(
|
|
utils.get_auth_token(req), endpoint_template_id)
|
|
return utils.send_result(200, req, endpoint_template)
|
|
|
|
@utils.wrap_error
|
|
def get_endpoints_for_tenant(self, req, tenant_id):
|
|
marker, limit, url = get_marker_limit_and_url(req)
|
|
endpoints = config.SERVICE.get_tenant_endpoints(
|
|
utils.get_auth_token(req), marker, limit, url, tenant_id)
|
|
return utils.send_result(200, req, endpoints)
|
|
|
|
@utils.wrap_error
|
|
def add_endpoint_to_tenant(self, req, tenant_id):
|
|
endpoint = utils.get_normalized_request_content(EndpointTemplate, req)
|
|
return utils.send_result(201, req,
|
|
config.SERVICE.create_endpoint_for_tenant(
|
|
utils.get_auth_token(req), tenant_id, endpoint, get_url(req)))
|
|
|
|
@utils.wrap_error
|
|
def remove_endpoint_from_tenant(self, req, tenant_id, endpoint_id):
|
|
rval = config.SERVICE.delete_endpoint(utils.get_auth_token(req),
|
|
endpoint_id)
|
|
return utils.send_result(204, req, rval)
|