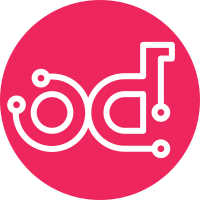
This change is a "futureproofing" thing. It has already been discussed that libvirt should not be a child of nova and should be removed out to the base docker directory (just like openvswitch isn't a child of neutron). That is not going to happen this cycle but when it does we can't change the name of the volume. This updates the volumes to the proper name of libvirtd. This is in contrast with the libvirtlogd volume that will be needed in newton due to libvirt 1.3 Of note, the container can remain named nova_libvirt since we can change that on the fly later without breaking instances. This wont break liberty as named_volumes are not backported yet. TrivialFix Change-Id: I16cf9e1b1dbba9b5a9f5cc883494580e276d4f72
73 lines
2.4 KiB
Bash
Executable File
73 lines
2.4 KiB
Bash
Executable File
#!/bin/bash
|
|
if [[ $(pgrep qemu) ]]; then
|
|
echo "Some qemu processes were detected."
|
|
echo "Docker will not be able to stop the nova_libvirt container with those running."
|
|
echo "Please clean them up before rerunning this script."
|
|
exit 1
|
|
fi
|
|
|
|
if [ -n "$1" ]; then
|
|
containers_to_kill=($(docker ps | grep -E "$1" | awk '{print $1}'))
|
|
volumes_to_remove=($(docker volume ls | grep -E "$1" | awk '{print $1}'))
|
|
else
|
|
containers_to_kill=(
|
|
bootstrap_{ceph_mon,cinder,glance,heat,heka,ironic,ironic_pxe,keystone,magnum,mistral,mongodb,murano,neutron,nova,nova_compute} \
|
|
cinder_{volume,scheduler,backup,api} \
|
|
ceph_{mon,rgw} \
|
|
cron \
|
|
elasticsearch \
|
|
glance_{api,registry} \
|
|
haproxy \
|
|
heat_{api{,_cfn},engine} \
|
|
heka \
|
|
horizon \
|
|
ironic_{inspector,conductor,api,pxe} \
|
|
keepalived \
|
|
keystone \
|
|
kibana \
|
|
kolla_toolbox \
|
|
magnum_{api,conductor} \
|
|
manila_{api,share,scheduler} \
|
|
mariadb \
|
|
memcached \
|
|
mistral_{api,engine,executor} \
|
|
murano_{api,engine} \
|
|
neutron_{server,dhcp_agent,l3_agent,linuxbridge_agent,metadata_agent,openvswitch_agent} \
|
|
nova_{scheduler,novncproxy,consoleauth,conductor,api,compute,libvirt,spicehtml5proxy,compute_ironic} \
|
|
openvswitch_{vswitchd,db} \
|
|
rabbitmq{,_bootstrap} \
|
|
swift_{account_{auditor,reaper,replicator,server},container_{auditor,replicator,server,updater},object_{auditor,expirer,replicator,server,updater},proxy_server,rsyncd}
|
|
)
|
|
ceph_osd_bootstrap=$(docker ps -a --filter "name=bootstrap_osd_*" --format "{{.Names}}")
|
|
ceph_osd_containers=$(docker ps -a --filter "name=ceph_osd_*" --format "{{.Names}}")
|
|
containers_to_kill="${containers_to_kill} ${ceph_osd_containers} ${ceph_osd_bootstrap}"
|
|
|
|
volumes_to_remove=(
|
|
ceph_mon{,_config} \
|
|
elasticsearch \
|
|
glance \
|
|
haproxy_socket \
|
|
heka{,_socket} \
|
|
ironic_pxe \
|
|
kolla_logs \
|
|
libvirtd \
|
|
mariadb \
|
|
mongodb \
|
|
neutron_metadata_socket \
|
|
nova_compute \
|
|
openvswitch_db \
|
|
rabbitmq
|
|
)
|
|
fi
|
|
|
|
echo "Stopping containers..."
|
|
(docker stop -t 2 ${containers_to_kill[@]} 2>&1) > /dev/null
|
|
|
|
echo "Removing containers..."
|
|
(docker rm -v -f ${containers_to_kill[@]} 2>&1) > /dev/null
|
|
|
|
echo "Removing volumes..."
|
|
(docker volume rm ${volumes_to_remove[@]} 2>&1) > /dev/null
|
|
|
|
echo "All cleaned up!"
|