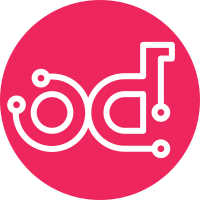
Watcher is part of the OpenStack big-tent and is formally known as "OpenStack Infrastructure Optimization service". Whilst it provides a range of default goals and strategies, the most relevant case is to enable re-balancing of the compute hosts by taking CPU usage (data from Ceilometer) into account and live migrating instances as required. Currently this only builds the Docker images for type source but binary builds are gracefully ignored. The ansible configurations will be part of a later commit. Change-Id: I9bb81ee625d9fcf6513e44e2ed20384e34da2adc Partial-bug: #1598929 Partially-implements: bp watcher Signed-off-by: Dave Walker (Daviey) <email@daviey.com>
162 lines
5.3 KiB
Python
162 lines
5.3 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import abc
|
|
import os
|
|
import sys
|
|
|
|
from mock import patch
|
|
from oslo_log import fixture as log_fixture
|
|
from oslo_log import log as logging
|
|
from oslotest import base
|
|
import six
|
|
import testtools
|
|
|
|
sys.path.append(
|
|
os.path.abspath(os.path.join(os.path.dirname(__file__), '../tools')))
|
|
from kolla.image import build
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
|
|
@six.add_metaclass(abc.ABCMeta)
|
|
class BuildTest(object):
|
|
excluded_images = abc.abstractproperty()
|
|
|
|
def setUp(self):
|
|
super(BuildTest, self).setUp()
|
|
self.useFixture(log_fixture.SetLogLevel([__name__],
|
|
logging.logging.INFO))
|
|
self.build_args = [__name__, "--debug", '--threads', '4']
|
|
|
|
@testtools.skipUnless(os.environ.get('DOCKER_BUILD_TEST'),
|
|
'Skip the docker build test')
|
|
def runTest(self):
|
|
with patch.object(sys, 'argv', self.build_args):
|
|
LOG.info("Running with args %s", self.build_args)
|
|
bad_results, good_results, unmatched_results = build.run_build()
|
|
|
|
failures = 0
|
|
for image, result in six.iteritems(bad_results):
|
|
if image in self.excluded_images:
|
|
if result is 'error':
|
|
continue
|
|
failures = failures + 1
|
|
LOG.warning(">>> Expected image '%s' to fail, please update"
|
|
" the excluded_images in source file above if the"
|
|
" image build has been fixed.", image)
|
|
else:
|
|
if result is not 'error':
|
|
continue
|
|
failures = failures + 1
|
|
LOG.critical(">>> Expected image '%s' to succeed!", image)
|
|
|
|
for image in unmatched_results.keys():
|
|
LOG.warning(">>> Image '%s' was not matched", image)
|
|
|
|
self.assertEqual(failures, 0, "%d failure(s) occurred" % failures)
|
|
|
|
|
|
class BuildTestCentosBinary(BuildTest, base.BaseTestCase):
|
|
excluded_images = ["kuryr",
|
|
"senlin-base",
|
|
"watcher-base"]
|
|
|
|
def setUp(self):
|
|
super(BuildTestCentosBinary, self).setUp()
|
|
self.build_args.extend(["--base", "centos",
|
|
"--type", "binary"])
|
|
|
|
|
|
class BuildTestCentosSource(BuildTest, base.BaseTestCase):
|
|
excluded_images = ["gnocchi-base",
|
|
"mistral-base"]
|
|
|
|
def setUp(self):
|
|
super(BuildTestCentosSource, self).setUp()
|
|
self.build_args.extend(["--base", "centos",
|
|
"--type", "source"])
|
|
|
|
|
|
class BuildTestUbuntuBinary(BuildTest, base.BaseTestCase):
|
|
excluded_images = ["zaqar"]
|
|
|
|
def setUp(self):
|
|
super(BuildTestUbuntuBinary, self).setUp()
|
|
self.build_args.extend(["--base", "ubuntu",
|
|
"--type", "binary"])
|
|
|
|
|
|
class BuildTestUbuntuSource(BuildTest, base.BaseTestCase):
|
|
excluded_images = []
|
|
|
|
def setUp(self):
|
|
super(BuildTestUbuntuSource, self).setUp()
|
|
self.build_args.extend(["--base", "ubuntu",
|
|
"--type", "source"])
|
|
|
|
|
|
class BuildTestOracleLinuxBinary(BuildTest, base.BaseTestCase):
|
|
excluded_images = ["kuryr",
|
|
"senlin-base",
|
|
"watcher-base"]
|
|
|
|
def setUp(self):
|
|
super(BuildTestOracleLinuxBinary, self).setUp()
|
|
self.build_args.extend(["--base", "oraclelinux",
|
|
"--type", "binary"])
|
|
|
|
|
|
class BuildTestOracleLinuxSource(BuildTest, base.BaseTestCase):
|
|
excluded_images = []
|
|
|
|
def setUp(self):
|
|
super(BuildTestOracleLinuxSource, self).setUp()
|
|
self.build_args.extend(["--base", "oraclelinux",
|
|
"--type", "source"])
|
|
|
|
|
|
class DeployTestCentosBinary(BuildTestCentosBinary):
|
|
def setUp(self):
|
|
super(DeployTestCentosBinary, self).setUp()
|
|
self.build_args.extend(["--profile", "gate"])
|
|
|
|
|
|
class DeployTestCentosSource(BuildTestCentosSource):
|
|
def setUp(self):
|
|
super(DeployTestCentosSource, self).setUp()
|
|
self.build_args.extend(["--profile", "gate"])
|
|
|
|
|
|
class DeployTestOracleLinuxBinary(BuildTestOracleLinuxBinary):
|
|
def setUp(self):
|
|
super(DeployTestOracleLinuxBinary, self).setUp()
|
|
self.build_args.extend(["--profile", "gate"])
|
|
|
|
|
|
class DeployTestOracleLinuxSource(BuildTestOracleLinuxSource):
|
|
def setUp(self):
|
|
super(DeployTestOracleLinuxSource, self).setUp()
|
|
self.build_args.extend(["--profile", "gate"])
|
|
|
|
|
|
class DeployTestUbuntuBinary(BuildTestUbuntuBinary):
|
|
def setUp(self):
|
|
super(DeployTestUbuntuBinary, self).setUp()
|
|
self.build_args.extend(["--profile", "gate"])
|
|
|
|
|
|
class DeployTestUbuntuSource(BuildTestUbuntuSource):
|
|
def setUp(self):
|
|
super(DeployTestUbuntuSource, self).setUp()
|
|
self.build_args.extend(["--profile", "gate"])
|