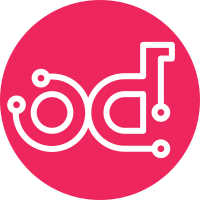
Working towards the blueprint that will add TLS protection for the external endpoints, kolla needs certificates. When kolla deploys OpenStack, the external VIP will need a server side certifcate. Clients that access those endpoints will need the public CA certificate that signed that certificate. This ansible script will create these two certificates to make it easy to use TLS in a test environment. The generated certificate files are: /etc/kolla/certificates/haproxy.pem (server side certificate) /etc/kolla/certificates/haproxy-ca.pem (CA certificate) The generated certificates are not suitable for use in a production environment, but will be useful for testing and verifying operations. Partially-implements: blueprint ssl-kolla Change-Id: I208777f9e5eee3bfb06810c7b18a2727beda234d
154 lines
3.8 KiB
Bash
Executable File
154 lines
3.8 KiB
Bash
Executable File
#!/bin/bash
|
|
#
|
|
# This script can be used to interact with kolla via ansible.
|
|
|
|
function find_base_dir {
|
|
local real_path=$(python -c "import os;print(os.path.realpath('$0'))")
|
|
local dir_name="$(dirname "$real_path")"
|
|
if [[ ${dir_name} == "/usr/bin" ]]; then
|
|
BASEDIR=/usr/share/kolla
|
|
elif [[ ${dir_name} == "/usr/local/bin" ]]; then
|
|
BASEDIR=/usr/local/share/kolla
|
|
else
|
|
BASEDIR="$(dirname ${dir_name})"
|
|
fi
|
|
}
|
|
|
|
function process_cmd {
|
|
echo "$ACTION : $CMD"
|
|
$CMD
|
|
if [[ $? -ne 0 ]]; then
|
|
echo "Command failed $CMD"
|
|
exit 1
|
|
fi
|
|
}
|
|
|
|
function usage {
|
|
cat <<EOF
|
|
Usage: $0 COMMAND [options]
|
|
|
|
Options:
|
|
--inventory, -i <inventory_path> Specify path to ansible inventory file
|
|
--playbook, -p <playbook_path> Specify path to ansible playbook file
|
|
--configdir <config_path> Specify path to directory with globals.yml
|
|
--keyfile, -k <key_file> Specify path to ansible vault keyfile
|
|
--help, -h Show this usage information
|
|
--tags, -t <tags> Only run plays and tasks tagged with these values
|
|
--verbose, -v Increase verbosity of ansible-playbook
|
|
|
|
Commands:
|
|
prechecks Do pre-deployment checks for hosts
|
|
deploy Deploy and start all kolla containers
|
|
post-deploy Do post deploy on deploy node
|
|
pull Pull all images for containers (only pulls, no runnnig container changes)
|
|
reconfigure Reconfigure OpenStack service
|
|
certificates Generate self-signed certificate for TLS *For Development Only*
|
|
EOF
|
|
}
|
|
|
|
|
|
SHORT_OPTS="hi:p:t:k:v"
|
|
LONG_OPTS="help,inventory:,playbook:,tags:,keyfile:,verbose,configdir:"
|
|
ARGS=$(getopt -o "${SHORT_OPTS}" -l "${LONG_OPTS}" --name "$0" -- "$@") || { usage >&2; exit 2; }
|
|
|
|
eval set -- "$ARGS"
|
|
|
|
find_base_dir
|
|
|
|
INVENTORY="${BASEDIR}/ansible/inventory/all-in-one"
|
|
PLAYBOOK="${BASEDIR}/ansible/site.yml"
|
|
VERBOSITY=
|
|
EXTRA_OPTS=
|
|
CONFIG_DIR="/etc/kolla"
|
|
|
|
while [ "$#" -gt 0 ]; do
|
|
case "$1" in
|
|
|
|
(--inventory|-i)
|
|
INVENTORY="$2"
|
|
shift 2
|
|
;;
|
|
|
|
(--playbook|-p)
|
|
PLAYBOOK="$2"
|
|
shift 2
|
|
;;
|
|
|
|
(--tags|-t)
|
|
EXTRA_OPTS="$EXTRA_OPTS --tags $2"
|
|
shift 2
|
|
;;
|
|
|
|
(--verbose|-v)
|
|
VERBOSITY="$VERBOSITY --verbose"
|
|
shift 1
|
|
;;
|
|
|
|
(--configdir)
|
|
CONFIG_DIR="$2"
|
|
shift 2
|
|
;;
|
|
|
|
(--keyfile|-k)
|
|
VAULT_PASS_FILE="$2"
|
|
EXTRA_OPTS="$EXTRA_OPTS --vault-password-file=$VAULT_PASS_FILE"
|
|
shift 2
|
|
;;
|
|
|
|
(--help|-h)
|
|
usage
|
|
shift
|
|
exit 0
|
|
;;
|
|
|
|
(--)
|
|
shift
|
|
break
|
|
;;
|
|
|
|
(*)
|
|
echo "error"
|
|
exit 3
|
|
;;
|
|
esac
|
|
done
|
|
|
|
case "$1" in
|
|
|
|
(prechecks)
|
|
ACTION="Pre-deployment checking"
|
|
PLAYBOOK="${BASEDIR}/ansible/prechecks.yml"
|
|
;;
|
|
(deploy)
|
|
ACTION="Deploying Playbooks"
|
|
EXTRA_OPTS="$EXTRA_OPTS -e action=deploy"
|
|
;;
|
|
(post-deploy)
|
|
ACTION="Post-Deploying Playbooks"
|
|
PLAYBOOK="${BASEDIR}/ansible/post-deploy.yml"
|
|
;;
|
|
(pull)
|
|
ACTION="Pulling Docker images"
|
|
EXTRA_OPTS="$EXTRA_OPTS -e action=pull"
|
|
;;
|
|
(upgrade)
|
|
ACTION="Upgrading OpenStack Environment"
|
|
EXTRA_OPTS="$EXTRA_OPTS -e action=upgrade"
|
|
;;
|
|
(reconfigure)
|
|
ACTION="Reconfigure OpenStack service"
|
|
EXTRA_OPTS="$EXTRA_OPTS -e action=reconfigure"
|
|
;;
|
|
(certificates)
|
|
ACTION="Generate TLS Certificates"
|
|
PLAYBOOK="${BASEDIR}/ansible/certificates.yml"
|
|
;;
|
|
(*) usage
|
|
exit 0
|
|
;;
|
|
esac
|
|
|
|
CONFIG_OPTS="-e @${CONFIG_DIR}/globals.yml -e @${CONFIG_DIR}/passwords.yml"
|
|
CMD="ansible-playbook -i $INVENTORY $CONFIG_OPTS $EXTRA_OPTS $PLAYBOOK $VERBOSITY"
|
|
process_cmd
|