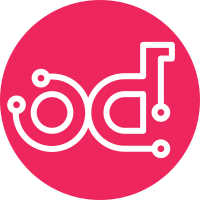
This commit adds support for cri-o by changing the binary initially used to run CNI plugin to runc and falling back to docker only in case it's not available. Also DevStack support for installing and configuring Kubernetes with cri-o is added. Implements: blueprint crio-support Depends-On: Ib049d66058429e499f5d0932c4a749820bec73ff Depends-On: Ic3c7d355a455298f43e37fb2aceddfd1e7eefaf2 Change-Id: I081edf0dbd4eb57826399c4820376381950080ed
65 lines
2.1 KiB
Bash
Executable File
65 lines
2.1 KiB
Bash
Executable File
#!/bin/bash -ex
|
|
|
|
function print_usage() {
|
|
set +ex
|
|
echo "$0" "[options]"
|
|
if [[ -n "$1" ]]; then
|
|
echo "Option $1 not found"
|
|
fi
|
|
echo "Options -----------------------------"
|
|
echo "-h/--help Displays this help message"
|
|
echo "-f/--dockerfile Specify the Dockerfile to use for building the CNI container"
|
|
echo "-b/--bin-dir Specify the path where to place the CNI executable"
|
|
echo "-c/--conf-dir Specify the path where to place the CNI configuration"
|
|
echo "-t/--tag Specify string to use as the tag part of the container image name, i.e., kuryr/cni:tag"
|
|
echo "-D/--no-daemon Do not run CNI as a daemon"
|
|
echo "-p/--podman Use podman instead of docker to build image"
|
|
}
|
|
|
|
for arg in "$@"; do
|
|
shift
|
|
case "$arg" in
|
|
"--help") set -- "$@" "-h" ;;
|
|
"--bin-dir") set -- "$@" "-b" ;;
|
|
"--conf-dir") set -- "$@" "-c" ;;
|
|
"--dockerfile") set -- "$@" "-f" ;;
|
|
"--tag") set -- "$@" "-t" ;;
|
|
"--no-daemon") set -- "$@" "-D" ;;
|
|
"--podman") set -- "$@" "-p" ;;
|
|
"--"*) print_usage "$arg" >&2; exit 1 ;;
|
|
*) set -- "$@" "$arg"
|
|
esac
|
|
done
|
|
|
|
#Default value
|
|
dockerfile="cni.Dockerfile"
|
|
image_name="kuryr/cni"
|
|
daemonized="True"
|
|
build_args=()
|
|
build_cmd="docker build"
|
|
|
|
OPTIND=1
|
|
|
|
while getopts "hf:b:c:t:D:p" opt; do
|
|
case "$opt" in
|
|
"h") print_usage; exit 0 ;;
|
|
"D") daemonized=False ;;
|
|
"f") dockerfile=${OPTARG} ;;
|
|
"b") build_args+=('--build-arg' "CNI_BIN_DIR_PATH=${OPTARG}") ;;
|
|
"c") build_args+=('--build-arg' "CNI_CONFIG_DIR_PATH=${OPTARG}") ;;
|
|
# Until https://github.com/containers/buildah/issues/1206 is resolved
|
|
# we need to use buildah directly.
|
|
"p") build_cmd="sudo buildah bud" && image_name="docker.io/kuryr/cni" ;;
|
|
"t") image_name=${image_name}:${OPTARG} ;;
|
|
"?") print_usage >&2; exit 1 ;;
|
|
esac
|
|
done
|
|
|
|
shift $((OPTIND - 1))
|
|
|
|
# create cni daemonset image
|
|
${build_cmd} -t "$image_name" \
|
|
--build-arg "CNI_DAEMON=$daemonized" \
|
|
"${build_args[@]}" \
|
|
-f "$dockerfile" .
|