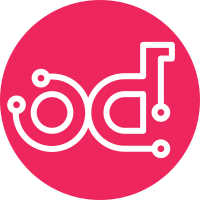
ZFS filesystem is going to be used for replication feature by Manila Generic share driver. So, add appropriate Manila image element as optional and enabled by default. Set env var 'MANILA_ENABLE_ZFS_SUPPORT' to any value other than 'yes' to disable its installation. Change-Id: If58186dc03d43430c1225e77aa80a1f85a62dd1b Closes-Bug: #1538658
189 lines
5.6 KiB
Bash
189 lines
5.6 KiB
Bash
#!/bin/bash
|
|
|
|
set -eu
|
|
set -o pipefail
|
|
|
|
SCRIPT_HOME=$(dirname $(readlink -f $0))
|
|
if [ -d $SCRIPT_HOME/../share/manila-elements ]; then
|
|
_PREFIX=$SCRIPT_HOME/../share/manila-elements
|
|
elif [ -d $SCRIPT_HOME/../../../elements ]; then
|
|
_PREFIX=$SCRIPT_HOME/../../..
|
|
else
|
|
_PREFIX=$SCRIPT_HOME/..
|
|
fi
|
|
export ELEMENTS_PATH=$_PREFIX/elements
|
|
|
|
# Collect configuration
|
|
# --------------------
|
|
# Development options:
|
|
DIB_UPDATE_REQUESTED=${DIB_UPDATE_REQUESTED:-true}
|
|
USE_OFFLINE_MODE=${USE_OFFLINE_MODE:-"yes"}
|
|
ENABLE_DEBUG_MODE=${ENABLE_DEBUG_MODE:-"no"}
|
|
DISABLE_IMG_COMPRESSION=${DISABLE_IMG_COMPRESSION:-"no"}
|
|
|
|
# Manila user settings
|
|
MANILA_USER=${MANILA_USER:-"manila"}
|
|
MANILA_PASSWORD=${MANILA_PASSWORD:-"manila"}
|
|
MANILA_USER_AUTHORIZED_KEYS="None"
|
|
|
|
# Manila image settings
|
|
MANILA_IMG_ARCH=${MANILA_IMG_ARCH:-"i386"}
|
|
MANILA_IMG_OS=${MANILA_IMG_OS:-"manila-ubuntu-core"}
|
|
MANILA_IMG_OS_VER=${MANILA_IMG_OS_VER:-"trusty"}
|
|
MANILA_IMG_NAME=${MANILA_IMG_NAME:-"manila-service-image.qcow2"}
|
|
|
|
# Manila features
|
|
MANILA_ENABLE_NFS_SUPPORT=${MANILA_ENABLE_NFS_SUPPORT:-"yes"}
|
|
MANILA_ENABLE_CIFS_SUPPORT=${MANILA_ENABLE_CIFS_SUPPORT:-"yes"}
|
|
|
|
# Manila Generic share driver replication feature requires ZFS:
|
|
MANILA_ENABLE_ZFS_SUPPORT=${MANILA_ENABLE_ZFS_SUPPORT:-"yes"}
|
|
|
|
# Verify configuration
|
|
# --------------------
|
|
REQUIRED_ELEMENTS="manila-ssh vm $MANILA_IMG_OS dhcp-all-interfaces cleanup-kernel-initrd"
|
|
OPTIONAL_ELEMENTS=
|
|
OPTIONAL_DIB_ARGS=
|
|
|
|
if [ "$MANILA_ENABLE_CIFS_SUPPORT" != "yes" ] && [ "$MANILA_ENABLE_NFS_SUPPORT" != "yes" ]; then
|
|
echo "You should enable NFS or CIFS support for manila image."
|
|
fi
|
|
|
|
if [ "$MANILA_ENABLE_NFS_SUPPORT" = "yes" ]; then
|
|
OPTIONAL_ELEMENTS="$OPTIONAL_ELEMENTS manila-nfs"
|
|
fi
|
|
|
|
if [ "$MANILA_ENABLE_CIFS_SUPPORT" = "yes" ]; then
|
|
OPTIONAL_ELEMENTS="$OPTIONAL_ELEMENTS manila-cifs"
|
|
fi
|
|
|
|
if [ "$MANILA_ENABLE_ZFS_SUPPORT" = "yes" ]; then
|
|
OPTIONAL_ELEMENTS="$OPTIONAL_ELEMENTS manila-zfs"
|
|
fi
|
|
|
|
if [ "$USE_OFFLINE_MODE" = "yes" ]; then
|
|
OPTIONAL_DIB_ARGS="$OPTIONAL_DIB_ARGS -offline"
|
|
fi
|
|
|
|
if [ "$ENABLE_DEBUG_MODE" = "yes" ]; then
|
|
OPTIONAL_DIB_ARGS="$OPTIONAL_DIB_ARGS -x"
|
|
MANILA_USER_AUTHORIZED_KEYS=${MANILA_USER_AUTHORIZED_KEYS:-"$HOME/.ssh/id_rsa.pub"}
|
|
fi
|
|
|
|
if [ "$DISABLE_IMG_COMPRESSION" = "yes" ]; then
|
|
OPTIONAL_DIB_ARGS="$OPTIONAL_DIB_ARGS -u"
|
|
fi
|
|
|
|
if [ "$MANILA_IMG_OS" = "manila-ubuntu-core" ] && [ "$MANILA_IMG_OS_VER" != "trusty" ]; then
|
|
echo "manila-ubuntu-core doesn't support '$MANILA_IMG_OS_VER' release."
|
|
echo "Change MANILA_IMG_OS to 'ubuntu' if you need another release."
|
|
fi
|
|
|
|
# Verify dependencies
|
|
# -------------------
|
|
if [ -e /etc/os-release ]; then
|
|
platform=$(cat /etc/os-release | awk -F= '/^ID=/ {print tolower($2);}')
|
|
# remove eventual quotes around ID=...
|
|
platform=$(echo $platform | sed -e 's,^",,;s,"$,,')
|
|
elif [ -e /etc/system-release ]; then
|
|
case "$(head -1 /etc/system-release)" in
|
|
"Red Hat Enterprise Linux Server"*)
|
|
platform=rhel
|
|
;;
|
|
"CentOS"*)
|
|
platform=centos
|
|
;;
|
|
*)
|
|
echo -e "Unknown value in /etc/system-release. Impossible to build images.\nAborting"
|
|
exit 2
|
|
;;
|
|
esac
|
|
else
|
|
echo -e "Unknown host OS. Impossible to build images.\nAborting"
|
|
exit 2
|
|
fi
|
|
|
|
is_installed() {
|
|
if [ "$platform" = 'ubuntu' ]; then
|
|
dpkg -s "$1" &> /dev/null
|
|
else
|
|
# centos, fedora, opensuse, or rhel
|
|
if ! rpm -q "$1" &> /dev/null; then
|
|
rpm -q "$(rpm -q --whatprovides "$1")"
|
|
fi
|
|
fi
|
|
}
|
|
|
|
need_required_packages() {
|
|
case "$platform" in
|
|
"ubuntu")
|
|
package_list="qemu kpartx"
|
|
;;
|
|
"fedora")
|
|
package_list="qemu-img kpartx"
|
|
;;
|
|
"opensuse")
|
|
package_list="qemu kpartx"
|
|
;;
|
|
"rhel" | "centos")
|
|
package_list="qemu-kvm qemu-img kpartx"
|
|
;;
|
|
*)
|
|
echo -e "Unknown platform '$platform' for the package list.\nAborting"
|
|
exit 2
|
|
;;
|
|
esac
|
|
|
|
for p in `echo $package_list`; do
|
|
if ! is_installed $p; then
|
|
return 0
|
|
fi
|
|
done
|
|
return 1
|
|
}
|
|
|
|
if need_required_packages; then
|
|
# install required packages if requested
|
|
if [ -n "$DIB_UPDATE_REQUESTED" ]; then
|
|
case "$platform" in
|
|
"ubuntu")
|
|
sudo apt-get update
|
|
sudo apt-get install $package_list -y
|
|
;;
|
|
"opensuse")
|
|
sudo zypper --non-interactive --gpg-auto-import-keys in $package_list
|
|
;;
|
|
"fedora" | "rhel" | "centos")
|
|
if [ ${platform} = "centos" ]; then
|
|
# install EPEL repo, in order to install argparse
|
|
sudo rpm -Uvh --force http://dl.fedoraproject.org/pub/epel/6/x86_64/epel-release-6-8.noarch.rpm
|
|
fi
|
|
sudo yum install $package_list -y
|
|
;;
|
|
*)
|
|
echo -e "Unknown platform '$platform' for installing packages.\nAborting"
|
|
exit 2
|
|
;;
|
|
esac
|
|
else
|
|
echo "Missing one of the following packages: $package_list"
|
|
echo "Please install manually or rerun with the update option (-u)."
|
|
exit 1
|
|
fi
|
|
fi
|
|
|
|
# Export diskimage-builder settings
|
|
# ---------------------------------
|
|
export DIB_DEFAULT_INSTALLTYPE=package
|
|
export DIB_RELEASE=$MANILA_IMG_OS_VER
|
|
|
|
# User settings
|
|
export DIB_MANILA_USER_USERNAME=$MANILA_USER
|
|
export DIB_MANILA_USER_PASSWORD=$MANILA_PASSWORD
|
|
export DIB_MANILA_USER_AUTHORIZED_KEYS=$MANILA_USER_AUTHORIZED_KEYS
|
|
|
|
# Build image
|
|
# -----------
|
|
disk-image-create -a $MANILA_IMG_ARCH $OPTIONAL_DIB_ARGS -o $MANILA_IMG_NAME\
|
|
$OPTIONAL_ELEMENTS $REQUIRED_ELEMENTS
|