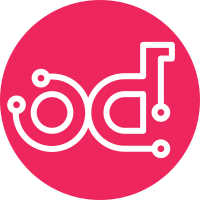
After port of extensions to core API we need to rename their URLs. So, rename URLs and bump microversion for it. Make new URLs work only with new microversion(s) 2.7+ and old with old microversions 1.0-2.6. Add separate API router for v2 API as now we should split v1 and v2 logic. Also, move updated APIs under v2 directory that will be used by both API routers - v1 and v2. List of updated collections is following: - os-availability-zone -> availability-zones - os-services -> services - os-quota-sets -> quota-sets - os-quota-class-sets -> quota-class-sets - os-share-manage -> shares/manage - os-share-unmanage -> shares/%s/action List of updated member actions is following: - os-share-unmanage/%(share_id)s/unmanage -> shares/%(share_id)s/action - types/%(id)s/os-share-type-access -> types/%(id)s/share_type_access List of updated action names is following: - os-access_allow -> access_allow - os-access_deny -> access_deny - os-access_list -> access_list - os-reset_status -> reset_status - os-force_delete -> force_delete - os-migrate_share -> migrate_share - os-extend -> extend - os-shrink -> shrink List of updated attribute names is following: - os-share-type-access -> share-type-access Partially implements bp ext-to-core Change-Id: I82f00114db985b4b3bf4db0a64191559508ac600
68 lines
2.2 KiB
Python
68 lines
2.2 KiB
Python
# Copyright (c) 2013 OpenStack Foundation
|
|
# Copyright (c) 2015 Mirantis inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from manila.api.openstack import wsgi
|
|
from manila.api.views import availability_zones as availability_zones_views
|
|
from manila import db
|
|
|
|
|
|
class AvailabilityZoneMixin(object):
|
|
"""The Availability Zone API controller common logic.
|
|
|
|
Mixin class that should be inherited by Availability Zone API controllers,
|
|
which are used for different API URLs and microversions.
|
|
"""
|
|
|
|
resource_name = "availability_zone"
|
|
_view_builder_class = availability_zones_views.ViewBuilder
|
|
|
|
@wsgi.Controller.authorize("index")
|
|
def _index(self, req):
|
|
"""Describe all known availability zones."""
|
|
views = db.availability_zone_get_all(req.environ['manila.context'])
|
|
return self._view_builder.detail_list(views)
|
|
|
|
|
|
class AvailabilityZoneControllerLegacy(AvailabilityZoneMixin, wsgi.Controller):
|
|
"""Deprecated Availability Zone API controller.
|
|
|
|
Used by legacy API v1 and v2 microversions from 2.0 to 2.6.
|
|
Registered under deprecated API URL 'os-availability-zone'.
|
|
"""
|
|
|
|
@wsgi.Controller.api_version('1.0', '2.6')
|
|
def index(self, req):
|
|
return self._index(req)
|
|
|
|
|
|
class AvailabilityZoneController(AvailabilityZoneMixin, wsgi.Controller):
|
|
"""Availability Zone API controller.
|
|
|
|
Used only by API v2 starting from microversion 2.7.
|
|
Registered under API URL 'availability-zones'.
|
|
"""
|
|
|
|
@wsgi.Controller.api_version('2.7')
|
|
def index(self, req):
|
|
return self._index(req)
|
|
|
|
|
|
def create_resource_legacy():
|
|
return wsgi.Resource(AvailabilityZoneControllerLegacy())
|
|
|
|
|
|
def create_resource():
|
|
return wsgi.Resource(AvailabilityZoneController())
|