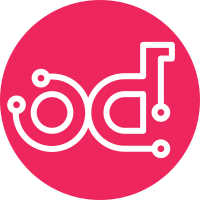
As of this commit, to change the configuration using Banana, we need to make an HTTP POST request to `/banana` REST API. This API is temporary and is likely to be changed later. The implementation is done entirely in the `banana` module. Under this module there are: * `typeck` module contains the type checker, * `grammar` module contains the parser and the AST and, * `eval` module contains the interpreter. Additionally, a test framework has been created to ease the test of particular conditions of the language. Within the banana module, there is a README.md file for each associated sub-module explaining further the details of the language. Once this commit is merged, there's still a lot that can be improved: - All components should be tested in Banana. - The 'deadpathck' pass could be improved (see TODO) - We don't support generated JSON ingestors yet. - Imports will be key for reusability (not implemented). Change-Id: I1305bdfa0606f30619b31404afbe0acf111c029f
54 lines
1.5 KiB
Python
54 lines
1.5 KiB
Python
#!/usr/bin/env python
|
|
|
|
# Copyright (c) 2016 Hewlett Packard Enterprise Development Company, L.P.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from monasca_analytics.voter import base
|
|
from test.util_for_testing import MonanasTestCase
|
|
|
|
|
|
class BaseVoterTest(MonanasTestCase):
|
|
|
|
def setUp(self):
|
|
super(BaseVoterTest, self).setUp()
|
|
self.vot = VoterBasicChild("fake_id", "fake_config")
|
|
|
|
def tearDown(self):
|
|
super(BaseVoterTest, self).tearDown()
|
|
|
|
def test_suggest_structure_no_smls_or_structures(self):
|
|
self.vot.suggest_structure("who", "struct")
|
|
|
|
|
|
class VoterBasicChild(base.BaseVoter):
|
|
|
|
def __init__(self, _id, _config):
|
|
self.elect_cnt = 0
|
|
super(VoterBasicChild, self).__init__(_id, _config)
|
|
|
|
def elect_structure(self, structures):
|
|
self.elect_cnt += 1
|
|
|
|
@staticmethod
|
|
def validate_config(_config):
|
|
pass
|
|
|
|
@staticmethod
|
|
def get_default_config():
|
|
return {"module": VoterBasicChild.__name__}
|
|
|
|
@staticmethod
|
|
def get_params():
|
|
return []
|