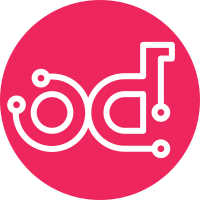
Fixes bug 1127664 Network cannot be created in NEC plugin when OFC network ID is unique inside a tenant. Some OFC implmenetations generate a network ID unique inside a tenant. In this case generated network IDs on can be duplicated in system-wide. To fix it, this changes resource ID on OFC to REST URI to make sure IDs on OFC globally unique. Fixes bug 1120962 Make sure NEC plugin creates shared networks In Quantum resource relationship is not limited inside a tenant. E.g., a non-owner tenant can create a port on a shared network. To deal with it the provider layer should not be aware of tenants each resource belongs to even when it has a kind of tenant concept. This commit changes ofc_manager to pass a parent resource for resource creation and identify a resouce by REST URI used to access OFC resources. It decouples Quantum resource access model from OFC resource models. OFC IDs created before this commit are also looked up. Primary keys of OFC ID mapping tables are changed to quantum_id because most of all accesses to these mapping tables are done by quantum_id. However the current version of alembic does not support changing primary keys, so new OFC ID mapping tables for tenant, network, port and packet filter are created. Dropping the previous mapping tables will be done along with the data migration logic. This commit also changes the following minor issues. - Make sure ID on ProgrammableFlow OpenFlow controller (PFC) is less than 32 chars. The current PFC accepts only 31 chars max as ID and 127 chars as a description string. - Some database accesses created their own session and did not support subtransactions. Make sure to use context.session passed from the API layer. - Removes Unused methods (update_network, update_port) in trema/pfc drivers. Change-Id: Ib4bb830e5f537c789974fa7b77f06eaeacb65333
136 lines
4.9 KiB
Python
136 lines
4.9 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
#
|
|
# Copyright 2012 NEC Corporation. All rights reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
# @author: Ryota MIBU
|
|
# @author: Akihiro MOTOKI
|
|
|
|
from abc import ABCMeta, abstractmethod
|
|
|
|
|
|
class OFCDriverBase(object):
|
|
"""OpenFlow Controller (OFC) Driver Specification.
|
|
|
|
OFCDriverBase defines the minimum set of methods required by this plugin.
|
|
It would be better that other methods like update_* are implemented.
|
|
"""
|
|
|
|
__metaclass__ = ABCMeta
|
|
|
|
@abstractmethod
|
|
def create_tenant(self, description, tenant_id=None):
|
|
"""Create a new tenant at OpenFlow Controller.
|
|
|
|
:param description: A description of this tenant.
|
|
:param tenant_id: A hint of OFC tenant ID.
|
|
A driver could use this id as a OFC id or ignore it.
|
|
:returns: ID of the tenant created at OpenFlow Controller.
|
|
:raises: quantum.plugin.nec.common.exceptions.OFCException
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def delete_tenant(self, ofc_tenant_id):
|
|
"""Delete a tenant at OpenFlow Controller.
|
|
|
|
:raises: quantum.plugin.nec.common.exceptions.OFCException
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def create_network(self, ofc_tenant_id, description, network_id=None):
|
|
"""Create a new network on specified OFC tenant at OpenFlow Controller.
|
|
|
|
:param ofc_tenant_id: a OFC tenant ID in which a new network belongs.
|
|
:param description: A description of this network.
|
|
:param network_id: A hint of an ID of OFC network.
|
|
:returns: ID of the network created at OpenFlow Controller.
|
|
ID returned must be unique in the OpenFlow Controller.
|
|
If a network is identified in conjunction with other information
|
|
such as a tenant ID, such information should be included in the ID.
|
|
:raises: quantum.plugin.nec.common.exceptions.OFCException
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def delete_network(self, ofc_network_id):
|
|
"""Delete a netwrok at OpenFlow Controller.
|
|
|
|
:raises: quantum.plugin.nec.common.exceptions.OFCException
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def create_port(self, ofc_network_id, portinfo,
|
|
port_id=None):
|
|
"""Create a new port on specified network at OFC.
|
|
|
|
:param ofc_network_id: a OFC tenant ID in which a new port belongs.
|
|
:param portinfo: An OpenFlow information of this port.
|
|
{'datapath_id': Switch ID that a port connected.
|
|
'port_no': Port Number that a port connected on a Swtich.
|
|
'vlan_id': VLAN ID that a port tagging.
|
|
'mac': Mac address.
|
|
}
|
|
:param port_id: A hint of an ID of OFC port.
|
|
ID returned must be unique in the OpenFlow Controller.
|
|
|
|
If a port is identified in combination with a network or
|
|
a tenant, such information should be included in the ID.
|
|
|
|
:returns: ID of the port created at OpenFlow Controller.
|
|
:raises: quantum.plugin.nec.common.exceptions.OFCException
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def delete_port(self, ofc_port_id):
|
|
"""Delete a port at OpenFlow Controller.
|
|
|
|
:raises: quantum.plugin.nec.common.exceptions.OFCException
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def convert_ofc_tenant_id(self, context, ofc_tenant_id):
|
|
"""Convert old-style ofc tenand id to new-style one.
|
|
|
|
:param context: quantum context object
|
|
:param ofc_tenant_id: ofc_tenant_id to be converted
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def convert_ofc_network_id(self, context, ofc_network_id,
|
|
tenant_id):
|
|
"""Convert old-style ofc network id to new-style one.
|
|
|
|
:param context: quantum context object
|
|
:param ofc_network_id: ofc_network_id to be converted
|
|
:param tenant_id: quantum tenant_id of the network
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def convert_ofc_port_id(self, context, ofc_port_id,
|
|
tenant_id, network_id):
|
|
"""Convert old-style ofc port id to new-style one.
|
|
|
|
:param context: quantum context object
|
|
:param ofc_port_id: ofc_port_id to be converted
|
|
:param tenant_id: quantum tenant_id of the port
|
|
:param network_id: quantum network_id of the port
|
|
"""
|
|
pass
|