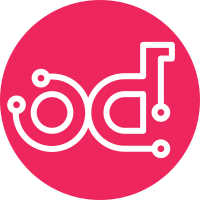
Previously when starting the dhcp agent the sync_state() process would be extremely expensive as it would query quantum server for each network. In order to improve performance a get_active_networks_info() was added so this information could be retrieved in one query rather than doing a query for each active network. The second part of this patch optimizes the logic to avoid calling get_dhcp_port(). Previously, this method was called once for each network which makes a call to get_subnets() and get_ports() unnecessarily as the dhcp agent can determine itself if it needs to update a port or create a port for dhcp. This patch also threads the inital sync process and maintains backwards compatibility with the previous rpc api. There was also a trivial change to the nvp_plugin where filters are assumed to be a dict. implements blueprint improve-dhcp-agent-performance Change-Id: I3b631057f595250dad76516faa9b421789f60953
101 lines
3.8 KiB
Python
101 lines
3.8 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright 2013 Cloudbase Solutions SRL
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
# @author: Alessandro Pilotti, Cloudbase Solutions Srl
|
|
|
|
from neutron.common import constants as q_const
|
|
from neutron.common import rpc as q_rpc
|
|
from neutron.db import dhcp_rpc_base
|
|
from neutron.db import l3_rpc_base
|
|
from neutron.openstack.common import log as logging
|
|
from neutron.plugins.hyperv import db as hyperv_db
|
|
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
|
|
class HyperVRpcCallbacks(
|
|
dhcp_rpc_base.DhcpRpcCallbackMixin,
|
|
l3_rpc_base.L3RpcCallbackMixin):
|
|
|
|
# Set RPC API version to 1.0 by default.
|
|
RPC_API_VERSION = '1.1'
|
|
|
|
def __init__(self, notifier):
|
|
self.notifier = notifier
|
|
self._db = hyperv_db.HyperVPluginDB()
|
|
|
|
def create_rpc_dispatcher(self):
|
|
'''Get the rpc dispatcher for this manager.
|
|
|
|
If a manager would like to set an rpc API version, or support more than
|
|
one class as the target of rpc messages, override this method.
|
|
'''
|
|
return q_rpc.PluginRpcDispatcher([self])
|
|
|
|
def get_device_details(self, rpc_context, **kwargs):
|
|
"""Agent requests device details."""
|
|
agent_id = kwargs.get('agent_id')
|
|
device = kwargs.get('device')
|
|
LOG.debug(_("Device %(device)s details requested from %(agent_id)s"),
|
|
{'device': device, 'agent_id': agent_id})
|
|
port = self._db.get_port(device)
|
|
if port:
|
|
binding = self._db.get_network_binding(None, port['network_id'])
|
|
entry = {'device': device,
|
|
'network_id': port['network_id'],
|
|
'port_id': port['id'],
|
|
'admin_state_up': port['admin_state_up'],
|
|
'network_type': binding.network_type,
|
|
'segmentation_id': binding.segmentation_id,
|
|
'physical_network': binding.physical_network}
|
|
# Set the port status to UP
|
|
self._db.set_port_status(port['id'], q_const.PORT_STATUS_ACTIVE)
|
|
else:
|
|
entry = {'device': device}
|
|
LOG.debug(_("%s can not be found in database"), device)
|
|
return entry
|
|
|
|
def update_device_down(self, rpc_context, **kwargs):
|
|
"""Device no longer exists on agent."""
|
|
# TODO(garyk) - live migration and port status
|
|
agent_id = kwargs.get('agent_id')
|
|
device = kwargs.get('device')
|
|
LOG.debug(_("Device %(device)s no longer exists on %(agent_id)s"),
|
|
{'device': device, 'agent_id': agent_id})
|
|
port = self._db.get_port(device)
|
|
if port:
|
|
entry = {'device': device,
|
|
'exists': True}
|
|
# Set port status to DOWN
|
|
self._db.set_port_status(port['id'], q_const.PORT_STATUS_DOWN)
|
|
else:
|
|
entry = {'device': device,
|
|
'exists': False}
|
|
LOG.debug(_("%s can not be found in database"), device)
|
|
return entry
|
|
|
|
def tunnel_sync(self, rpc_context, **kwargs):
|
|
"""Tunnel sync.
|
|
|
|
Dummy function for ovs agent running on Linux to
|
|
work with Hyper-V plugin and agent.
|
|
"""
|
|
entry = dict()
|
|
entry['tunnels'] = {}
|
|
# Return the list of tunnels IP's to the agent
|
|
return entry
|