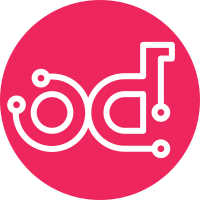
Change I583aef9735cfa5984312507f66198d009eef9ce2 moved _make_flavor_dict inside of the transaction to avoid detached instance errors on loading the relationship. However, we should avoid the pattern where we can of building the response before the changes are flushed to the database because things like default values on columns will not be evaluated yet. This moves it back out of the transaction and adds a subquery lazy load to the relationship required by the dict extend function to avoid the detached instance error. Partially-Implements blueprint: enginefacade-switch Change-Id: I5d01ca79ee2970849c3f807232f658fa19a23592
50 lines
2.2 KiB
Python
50 lines
2.2 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from neutron_lib.db import constants as db_const
|
|
from neutron_lib.db import model_base
|
|
import sqlalchemy as sa
|
|
from sqlalchemy import orm
|
|
|
|
|
|
class Flavor(model_base.BASEV2, model_base.HasId):
|
|
name = sa.Column(sa.String(db_const.NAME_FIELD_SIZE))
|
|
description = sa.Column(sa.String(db_const.LONG_DESCRIPTION_FIELD_SIZE))
|
|
enabled = sa.Column(sa.Boolean, nullable=False, default=True,
|
|
server_default=sa.sql.true())
|
|
# Make it True for multi-type flavors
|
|
service_type = sa.Column(sa.String(36), nullable=True)
|
|
service_profiles = orm.relationship("FlavorServiceProfileBinding",
|
|
cascade="all, delete-orphan", lazy="subquery")
|
|
|
|
|
|
class ServiceProfile(model_base.BASEV2, model_base.HasId):
|
|
description = sa.Column(sa.String(db_const.LONG_DESCRIPTION_FIELD_SIZE))
|
|
driver = sa.Column(sa.String(1024), nullable=False)
|
|
enabled = sa.Column(sa.Boolean, nullable=False, default=True,
|
|
server_default=sa.sql.true())
|
|
metainfo = sa.Column(sa.String(4096))
|
|
flavors = orm.relationship("FlavorServiceProfileBinding")
|
|
|
|
|
|
class FlavorServiceProfileBinding(model_base.BASEV2):
|
|
flavor_id = sa.Column(sa.String(36),
|
|
sa.ForeignKey("flavors.id",
|
|
ondelete="CASCADE"),
|
|
nullable=False, primary_key=True)
|
|
flavor = orm.relationship(Flavor)
|
|
service_profile_id = sa.Column(sa.String(36),
|
|
sa.ForeignKey("serviceprofiles.id",
|
|
ondelete="CASCADE"),
|
|
nullable=False, primary_key=True)
|
|
service_profile = orm.relationship(ServiceProfile)
|