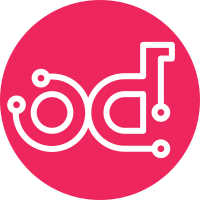
If both are run under the same process, and api_workers >= 2, the server process will instantiate two oslo_service.ProcessLauncher instances This should be avoided [0], and indeed causes issues on subprocess and signal handling: killed RPC workers not respawning, SIGHUP on master process leading to unresponsive server, signal not properly sent to all child processes, ... To avoid this, use the wsgi ProcessLauncher instance if it exists [0] https://docs.openstack.org/oslo.service/latest/user/usage.html#launchers Change-Id: Ic821f8ca84add9c8137ef712031afb43e491591c Closes-Bug: #1780139
47 lines
1.5 KiB
Python
47 lines
1.5 KiB
Python
#!/usr/bin/env python
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import eventlet
|
|
|
|
from oslo_log import log
|
|
|
|
from neutron import service
|
|
|
|
LOG = log.getLogger(__name__)
|
|
|
|
|
|
def eventlet_wsgi_server():
|
|
neutron_api = service.serve_wsgi(service.NeutronApiService)
|
|
start_api_and_rpc_workers(neutron_api)
|
|
|
|
|
|
def start_api_and_rpc_workers(neutron_api):
|
|
try:
|
|
worker_launcher = service.start_all_workers(neutron_api)
|
|
|
|
pool = eventlet.GreenPool()
|
|
api_thread = pool.spawn(neutron_api.wait)
|
|
plugin_workers_thread = pool.spawn(worker_launcher.wait)
|
|
|
|
# api and other workers should die together. When one dies,
|
|
# kill the other.
|
|
api_thread.link(lambda gt: plugin_workers_thread.kill())
|
|
plugin_workers_thread.link(lambda gt: api_thread.kill())
|
|
|
|
pool.waitall()
|
|
except NotImplementedError:
|
|
LOG.info("RPC was already started in parent process by "
|
|
"plugin.")
|
|
|
|
neutron_api.wait()
|