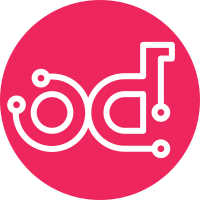
Previously we assumed that we can look up the resource provider (created by nova) to be used as the parent of the agent and physical NIC resource provider tree by the name set in the config option DEFAULT.host. This assumption was wrong. While nova-compute's DEFAULT.host and neutron-agent's DEFAULT.host must match for port binding to work, the root resource provider created by nova does not belong to the compute host (where nova-compute runs) but it belongs to the compute nodes (i.e. hypervisors). Actually there may be multiple compute nodes managed by a single nova-compute (think of ironic). Plus the value of DEFAULT.host and the compute node's ID may be different even when nova-compute manages a hypervisor on the same host because of various deployment considerations. For example when tripleo does not manage the undercloud (so a libvirt hypervisor returns the plain hostname), but the same tripleo enforces it's host naming conventions in nova's and neutron's DEFAULT.host settings. This change enables neutron to use the hypervisor name to locate the root of the resource provider tree. We introduce a new configuration option for (1) ovs-agent: resource_provider_hypervisors, for example: [ovs] bridge_mappings = physnet0:br-physnet0,... resource_provider_bandwidths = br-physnet0:10000000:10000000,... resource_provider_hypervisors = br-physnet0:hypervisor0,... (2) sriov-agent: resource_provider_hypervisors, for example: [sriov_nic] bridge_mappings = physnet1:ens5,... resource_provider_bandwidths = ens5:10000000:10000000,... resource_provider_hypervisors = ens5:hypervisor1,... For both agents 'resource_provider_hypervisors' values default to socket.gethostname() for each key in resource_provider_bandwidths. We try to not block later developments in which one neutron agent may manage devices on multiple hosts. That's why we allow the each physdev to be associated with a different hypervisor. But here we do not try to solve the problem that the natural physdev identifiers may not be unique accross multiple hosts. We leave solving this problem to whoever wants to implement an agent handling devices of multiple hosts. (3) We extend report_state message's configurations field alike: { 'bridge_mappings': {'physnet0': 'br-physnet0'}, 'resource_provider_bandwidths': { 'br-physnet0': {'egress': 10000000, 'ingress': 10000000}}, 'resource_provider_hypervisors': {'br-physnet0': 'hypervisor0'}, ... } (4) In neutron-server we use report_state.configurations.resource_provider_hypervisors.PHYSDEV when selecting parent resource provider for agent and physdev RP-tree. When not available in the message we fall back to using report_state.host as before. Since we only changed the free-format configurations field of the report_state message rpc version is not bumped and we expect this change to be backported to stein and train. Change-Id: I9b08a3a9c20b702b745b41d4885fb5120fd665ce Closes-Bug: #1853840
95 lines
3.2 KiB
Python
95 lines
3.2 KiB
Python
# Copyright 2015 Cloudbase Solutions.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import os
|
|
import socket
|
|
|
|
from neutron_lib.utils import runtime
|
|
from oslo_config import cfg
|
|
from oslo_log import log as logging
|
|
from oslo_utils import timeutils
|
|
|
|
from neutron.conf.agent import common as config
|
|
from neutron.conf.agent.database import agents_db
|
|
|
|
|
|
if os.name == 'nt':
|
|
from neutron.agent.windows import utils
|
|
else:
|
|
from neutron.agent.linux import utils
|
|
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
config.register_root_helper(cfg.CONF)
|
|
agents_db.register_db_agents_opts()
|
|
|
|
INTERFACE_NAMESPACE = 'neutron.interface_drivers'
|
|
|
|
|
|
create_process = utils.create_process
|
|
kill_process = utils.kill_process
|
|
execute = utils.execute
|
|
get_root_helper_child_pid = utils.get_root_helper_child_pid
|
|
pid_invoked_with_cmdline = utils.pid_invoked_with_cmdline
|
|
|
|
|
|
def load_interface_driver(conf, get_networks_callback=None):
|
|
"""Load interface driver for agents like DHCP or L3 agent.
|
|
|
|
:param conf: Driver configuration object
|
|
:param get_networks_callback: A callback to get network information.
|
|
This will be passed as additional keyword
|
|
argument to the interface driver.
|
|
:raises SystemExit of 1 if driver cannot be loaded
|
|
"""
|
|
|
|
try:
|
|
loaded_class = runtime.load_class_by_alias_or_classname(
|
|
INTERFACE_NAMESPACE, conf.interface_driver)
|
|
return loaded_class(conf, get_networks_callback=get_networks_callback)
|
|
except ImportError:
|
|
LOG.error("Error loading interface driver '%s'",
|
|
conf.interface_driver)
|
|
raise SystemExit(1)
|
|
|
|
|
|
def is_agent_down(heart_beat_time):
|
|
return timeutils.is_older_than(heart_beat_time,
|
|
cfg.CONF.agent_down_time)
|
|
|
|
|
|
# TODO(bence romsics): rehome this to neutron_lib.placement.utils
|
|
def default_rp_hypervisors(hypervisors, device_mappings):
|
|
"""Fill config option 'resource_provider_hypervisors' with defaults.
|
|
|
|
Default hypervisor names to socket.gethostname(). Since libvirt knows
|
|
itself by the same name, the default is good for libvirt.
|
|
|
|
:param hypervisors: Config option 'resource_provider_hypervisors'
|
|
as parsed by oslo.config, that is a dict with keys of physical devices
|
|
and values of hypervisor names.
|
|
:param device_mappings: Device mappings standardized to the list-valued
|
|
format.
|
|
"""
|
|
default_hypervisor = socket.gethostname()
|
|
rv = {}
|
|
for _physnet, devices in device_mappings.items():
|
|
for device in devices:
|
|
if device in hypervisors:
|
|
rv[device] = hypervisors[device]
|
|
else:
|
|
rv[device] = default_hypervisor
|
|
return rv
|