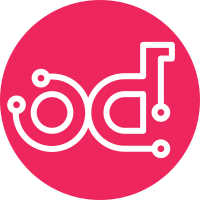
This patch makes necessary changes to ML2 type drivers and plugin manager for network segment range extension support when it is loaded. When the network segment range extension is not loaded, no impact to the current flow. When the extension is loaded, - populating a range that is managed from the configuration file [1]_, such as "VLAN IDs", "VXLAN VNI IDs", "GRE tunnel IDs", "Geneve VNI IDs" to the network segment range DB table as a "default" and "shared" entry to maintain backward compatibility; - reloading the "default" segment ranges when Neutron server starts/restarts; - creating a set of "default" network segment ranges out of the ML2-config-file-defined ranges [1]_ and the segment allocation operations are always retrieving the information from the DB to have the network segment ranges fully administered via API; - when a tenant allocates a segment, it will first allocate from an available segment range assigned to the tenant, and then a shared range if no tenant specific allocation is possible. [1] /etc/neutron/plugins/ml2/ml2_conf.ini Co-authored-by: Allain Legacy <Allain.legacy@windriver.com> Partially-implements: blueprint network-segment-range-management Change-Id: I522940fc4d054f5eec1110eb2c424e32e8ae6bad
64 lines
2.3 KiB
Python
64 lines
2.3 KiB
Python
# Copyright (c) 2013 OpenStack Foundation
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from neutron_lib import constants as p_const
|
|
from neutron_lib import exceptions as n_exc
|
|
from oslo_config import cfg
|
|
from oslo_log import log
|
|
|
|
from neutron.conf.plugins.ml2.drivers import driver_type
|
|
from neutron.db.models.plugins.ml2 import vxlanallocation as vxlan_alloc_model
|
|
from neutron.objects.plugins.ml2 import vxlanallocation as vxlan_obj
|
|
from neutron.plugins.ml2.drivers import type_tunnel
|
|
|
|
LOG = log.getLogger(__name__)
|
|
|
|
driver_type.register_ml2_drivers_vxlan_opts()
|
|
|
|
|
|
class VxlanTypeDriver(type_tunnel.EndpointTunnelTypeDriver):
|
|
|
|
def __init__(self):
|
|
super(VxlanTypeDriver, self).__init__(
|
|
vxlan_obj.VxlanAllocation, vxlan_obj.VxlanEndpoint)
|
|
self.model_segmentation_id = (
|
|
vxlan_alloc_model.VxlanAllocation.vxlan_vni)
|
|
|
|
def get_type(self):
|
|
return p_const.TYPE_VXLAN
|
|
|
|
def initialize(self):
|
|
try:
|
|
self._initialize(cfg.CONF.ml2_type_vxlan.vni_ranges)
|
|
except n_exc.NetworkTunnelRangeError:
|
|
LOG.exception("Failed to parse vni_ranges. "
|
|
"Service terminated!")
|
|
raise SystemExit()
|
|
|
|
def get_endpoints(self):
|
|
"""Get every vxlan endpoints from database."""
|
|
vxlan_endpoints = self._get_endpoints()
|
|
return [{'ip_address': vxlan_endpoint.ip_address,
|
|
'udp_port': vxlan_endpoint.udp_port,
|
|
'host': vxlan_endpoint.host}
|
|
for vxlan_endpoint in vxlan_endpoints]
|
|
|
|
def add_endpoint(self, ip, host, udp_port=p_const.VXLAN_UDP_PORT):
|
|
return self._add_endpoint(ip, host, udp_port=udp_port)
|
|
|
|
def get_mtu(self, physical_network=None):
|
|
mtu = super(VxlanTypeDriver, self).get_mtu()
|
|
return mtu - p_const.VXLAN_ENCAP_OVERHEAD if mtu else 0
|