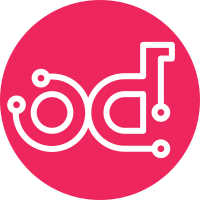
Since cellsv2 has required host mappings to be present, we have lost our automatic host registration behavior. This is a conscious choice, given that we do not want to have upcalls from the cells to the API database. This adds a periodic task to have the scheduler do the discovery routine, which for small deployments may be an acceptable amount of background overhead in exchange for the automatic behavior. There is also probably some amount of performance improvement that can be added to the host discovery routine to make it less of an issue. However, just generalizing the existing routine and letting it run periodically gives us some coverage, given where we are in the current cycle. Related to blueprint cells-scheduler-integration Change-Id: Iab5f172cdef35645bae56b9b384e032f3160e826
220 lines
8.3 KiB
Python
220 lines
8.3 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from sqlalchemy.orm import joinedload
|
|
|
|
from nova import context
|
|
from nova.db.sqlalchemy import api as db_api
|
|
from nova.db.sqlalchemy import api_models
|
|
from nova import exception
|
|
from nova.i18n import _
|
|
from nova.objects import base
|
|
from nova.objects import cell_mapping
|
|
from nova.objects import fields
|
|
|
|
|
|
def _cell_id_in_updates(updates):
|
|
cell_mapping_obj = updates.pop("cell_mapping", None)
|
|
if cell_mapping_obj:
|
|
updates["cell_id"] = cell_mapping_obj.id
|
|
|
|
|
|
def _apply_updates(context, db_mapping, updates):
|
|
db_mapping.update(updates)
|
|
db_mapping.save(context.session)
|
|
# NOTE: This is done because a later access will trigger a lazy load
|
|
# outside of the db session so it will fail. We don't lazy load
|
|
# cell_mapping on the object later because we never need a HostMapping
|
|
# without the CellMapping.
|
|
db_mapping.cell_mapping
|
|
return db_mapping
|
|
|
|
|
|
@base.NovaObjectRegistry.register
|
|
class HostMapping(base.NovaTimestampObject, base.NovaObject):
|
|
# Version 1.0: Initial version
|
|
VERSION = '1.0'
|
|
|
|
fields = {
|
|
'id': fields.IntegerField(read_only=True),
|
|
'host': fields.StringField(),
|
|
'cell_mapping': fields.ObjectField('CellMapping'),
|
|
}
|
|
|
|
def _get_cell_mapping(self):
|
|
with db_api.api_context_manager.reader.using(self._context) as session:
|
|
cell_map = (session.query(api_models.CellMapping)
|
|
.join(api_models.HostMapping)
|
|
.filter(api_models.HostMapping.host == self.host)
|
|
.first())
|
|
if cell_map is not None:
|
|
return cell_mapping.CellMapping._from_db_object(
|
|
self._context, cell_mapping.CellMapping(), cell_map)
|
|
|
|
def _load_cell_mapping(self):
|
|
self.cell_mapping = self._get_cell_mapping()
|
|
|
|
def obj_load_attr(self, attrname):
|
|
if attrname == 'cell_mapping':
|
|
self._load_cell_mapping()
|
|
|
|
@staticmethod
|
|
def _from_db_object(context, host_mapping, db_host_mapping):
|
|
for key in host_mapping.fields:
|
|
db_value = db_host_mapping.get(key)
|
|
if key == "cell_mapping":
|
|
# NOTE(dheeraj): If cell_mapping is stashed in db object
|
|
# we load it here. Otherwise, lazy loading will happen
|
|
# when .cell_mapping is accessed later
|
|
if not db_value:
|
|
continue
|
|
db_value = cell_mapping.CellMapping._from_db_object(
|
|
host_mapping._context, cell_mapping.CellMapping(),
|
|
db_value)
|
|
setattr(host_mapping, key, db_value)
|
|
host_mapping.obj_reset_changes()
|
|
host_mapping._context = context
|
|
return host_mapping
|
|
|
|
@staticmethod
|
|
@db_api.api_context_manager.reader
|
|
def _get_by_host_from_db(context, host):
|
|
db_mapping = (context.session.query(api_models.HostMapping)
|
|
.options(joinedload('cell_mapping'))
|
|
.filter(api_models.HostMapping.host == host)).first()
|
|
if not db_mapping:
|
|
raise exception.HostMappingNotFound(name=host)
|
|
return db_mapping
|
|
|
|
@base.remotable_classmethod
|
|
def get_by_host(cls, context, host):
|
|
db_mapping = cls._get_by_host_from_db(context, host)
|
|
return cls._from_db_object(context, cls(), db_mapping)
|
|
|
|
@staticmethod
|
|
@db_api.api_context_manager.writer
|
|
def _create_in_db(context, updates):
|
|
db_mapping = api_models.HostMapping()
|
|
return _apply_updates(context, db_mapping, updates)
|
|
|
|
@base.remotable
|
|
def create(self):
|
|
changes = self.obj_get_changes()
|
|
# cell_mapping must be mapped to cell_id for create
|
|
_cell_id_in_updates(changes)
|
|
db_mapping = self._create_in_db(self._context, changes)
|
|
self._from_db_object(self._context, self, db_mapping)
|
|
|
|
@staticmethod
|
|
@db_api.api_context_manager.writer
|
|
def _save_in_db(context, obj, updates):
|
|
db_mapping = context.session.query(api_models.HostMapping).filter_by(
|
|
id=obj.id).first()
|
|
if not db_mapping:
|
|
raise exception.HostMappingNotFound(name=obj.host)
|
|
return _apply_updates(context, db_mapping, updates)
|
|
|
|
@base.remotable
|
|
def save(self):
|
|
changes = self.obj_get_changes()
|
|
# cell_mapping must be mapped to cell_id for updates
|
|
_cell_id_in_updates(changes)
|
|
db_mapping = self._save_in_db(self._context, self, changes)
|
|
self._from_db_object(self._context, self, db_mapping)
|
|
self.obj_reset_changes()
|
|
|
|
@staticmethod
|
|
@db_api.api_context_manager.writer
|
|
def _destroy_in_db(context, host):
|
|
result = context.session.query(api_models.HostMapping).filter_by(
|
|
host=host).delete()
|
|
if not result:
|
|
raise exception.HostMappingNotFound(name=host)
|
|
|
|
@base.remotable
|
|
def destroy(self):
|
|
self._destroy_in_db(self._context, self.host)
|
|
|
|
|
|
@base.NovaObjectRegistry.register
|
|
class HostMappingList(base.ObjectListBase, base.NovaObject):
|
|
# Version 1.0: Initial version
|
|
VERSION = '1.0'
|
|
|
|
fields = {
|
|
'objects': fields.ListOfObjectsField('HostMapping'),
|
|
}
|
|
|
|
@staticmethod
|
|
@db_api.api_context_manager.reader
|
|
def _get_by_cell_id_from_db(context, cell_id):
|
|
return (context.session.query(api_models.HostMapping)
|
|
.options(joinedload('cell_mapping'))
|
|
.filter(api_models.HostMapping.cell_id == cell_id)).all()
|
|
|
|
@base.remotable_classmethod
|
|
def get_by_cell_id(cls, context, cell_id):
|
|
db_mappings = cls._get_by_cell_id_from_db(context, cell_id)
|
|
return base.obj_make_list(context, cls(), HostMapping, db_mappings)
|
|
|
|
|
|
def discover_hosts(ctxt, cell_uuid=None, status_fn=None):
|
|
# TODO(alaski): If this is not run on a host configured to use the API
|
|
# database most of the lookups below will fail and may not provide a
|
|
# great error message. Add a check which will raise a useful error
|
|
# message about running this from an API host.
|
|
|
|
from nova import objects
|
|
|
|
if not status_fn:
|
|
status_fn = lambda x: None
|
|
|
|
if cell_uuid:
|
|
cell_mappings = [objects.CellMapping.get_by_uuid(ctxt, cell_uuid)]
|
|
else:
|
|
cell_mappings = objects.CellMappingList.get_all(ctxt)
|
|
status_fn(_('Found %s cell mappings.') % len(cell_mappings))
|
|
|
|
host_mappings = []
|
|
for cm in cell_mappings:
|
|
if cm.is_cell0():
|
|
status_fn(_('Skipping cell0 since it does not contain hosts.'))
|
|
continue
|
|
if 'name' in cm and cm.name:
|
|
status_fn(_("Getting compute nodes from cell '%(name)s': "
|
|
"%(uuid)s") % {'name': cm.name,
|
|
'uuid': cm.uuid})
|
|
else:
|
|
status_fn(_("Getting compute nodes from cell: %(uuid)s") %
|
|
{'uuid': cm.uuid})
|
|
with context.target_cell(ctxt, cm):
|
|
compute_nodes = objects.ComputeNodeList.get_all(ctxt)
|
|
status_fn(_('Found %(num)s computes in cell: %(uuid)s') %
|
|
{'num': len(compute_nodes),
|
|
'uuid': cm.uuid})
|
|
for compute in compute_nodes:
|
|
status_fn(_("Checking host mapping for compute host "
|
|
"'%(host)s': %(uuid)s") %
|
|
{'host': compute.host, 'uuid': compute.uuid})
|
|
try:
|
|
objects.HostMapping.get_by_host(ctxt, compute.host)
|
|
except exception.HostMappingNotFound:
|
|
status_fn(_("Creating host mapping for compute host "
|
|
"'%(host)s': %(uuid)s") %
|
|
{'host': compute.host, 'uuid': compute.uuid})
|
|
host_mapping = objects.HostMapping(
|
|
ctxt, host=compute.host,
|
|
cell_mapping=cm)
|
|
host_mapping.create()
|
|
host_mappings.append(host_mapping)
|
|
return host_mappings
|