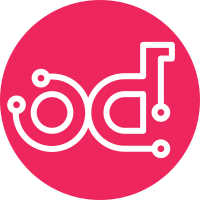
Import latest cfg from oslo-incubator with these changes: Add deprecated --logdir common opt Add deprecated --logfile common opt. Allow nova and others to override some logging defaults Fixing the trim for ListOp when reading from config file Fix set_default() with boolean CLI options Improve cfg's argparse sub-parsers support Hide the GroupAttr conf and group attributes Fix regression with cfg CLI arguments Fix broken --help with CommonConfigOpts Fix ListOpt to trim whitespace updating sphinx documentation Don't reference argparse._StoreAction Fix minor coding style issue Remove ConfigCliParser class Add support for positional arguments Use stock argparse behaviour for optional args Use stock argparse --usage behaviour Use stock argparse --version behaviour Remove add_option() method Completely remove cfg's disable_interspersed_args() argparse support for cfg The main cfg API change is that CONF() no longer returns the un-parsed CLI arguments. To handle these args, you need to use the support for positional arguments or sub-parsers. Switching nova-manage to use sub-parser based CLI arguments means the following changes in behaviour: - no more lazy matching of commands - e.g. 'nova-manage proj q' will no longer work. If we find out about common abbreviations used in peoples' scripts, we can easily add those. - the help output displayed if you run nova-manage without any args (or just a category) has changed - 'nova-manage version list' is no longer equivalent to 'nova-manage version' Change-Id: I19ef3a1c00e97af64d199e27cb1cdc5c63b46a82
83 lines
2.4 KiB
Python
Executable File
83 lines
2.4 KiB
Python
Executable File
#!/usr/bin/env python
|
|
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright (c) 2011 OpenStack, LLC.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""Admin/debug script to wipe rabbitMQ (AMQP) queues nova uses.
|
|
This can be used if you need to change durable options on queues,
|
|
or to wipe all messages in the queue system if things are in a
|
|
serious bad way.
|
|
|
|
"""
|
|
|
|
import datetime
|
|
import gettext
|
|
import os
|
|
import sys
|
|
import time
|
|
|
|
# If ../nova/__init__.py exists, add ../ to Python search path, so that
|
|
# it will override what happens to be installed in /usr/(local/)lib/python...
|
|
POSSIBLE_TOPDIR = os.path.normpath(os.path.join(os.path.abspath(sys.argv[0]),
|
|
os.pardir,
|
|
os.pardir))
|
|
if os.path.exists(os.path.join(POSSIBLE_TOPDIR, 'nova', '__init__.py')):
|
|
sys.path.insert(0, POSSIBLE_TOPDIR)
|
|
|
|
gettext.install('nova', unicode=1)
|
|
|
|
|
|
from nova import config
|
|
from nova import context
|
|
from nova import exception
|
|
from nova.openstack.common import cfg
|
|
from nova.openstack.common import log as logging
|
|
from nova.openstack.common import rpc
|
|
|
|
|
|
opts = [
|
|
cfg.MultiStrOpt('queues',
|
|
default=[],
|
|
positional=True,
|
|
help='Queues to delete'),
|
|
cfg.BoolOpt('delete_exchange',
|
|
default=False,
|
|
help='delete nova exchange too.'),
|
|
]
|
|
|
|
CONF = cfg.CONF
|
|
CONF.register_cli_opts(opts)
|
|
|
|
|
|
def delete_exchange(exch):
|
|
conn = rpc.create_connection()
|
|
x = conn.get_channel()
|
|
x.exchange_delete(exch)
|
|
|
|
|
|
def delete_queues(queues):
|
|
conn = rpc.create_connection()
|
|
x = conn.get_channel()
|
|
for q in queues:
|
|
x.queue_delete(q)
|
|
|
|
if __name__ == '__main__':
|
|
config.parse_args(sys.argv)
|
|
logging.setup("nova")
|
|
delete_queues(CONF.queues)
|
|
if CONF.delete_exchange:
|
|
delete_exchange(CONF.control_exchange)
|