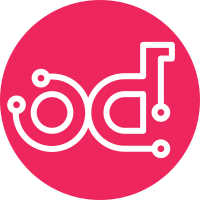
If we're booting from an existing volume but the instance is not being created in a requested availability zone, and cross_az_attach=False, we'll fail with a 400 since by default the volume is in the 'nova' AZ and the instance does not have an AZ set - because one wasn't requested and because it's not in a host aggregate yet. This refactors that AZ validation during server create in the API to do it before calling _validate_bdm so we get the pre-existing volumes early and if cross_az_attach=False, we validate the volume zone(s) against the instance AZ. If the [DEFAULT]/default_schedule_zone (for instances) is not set and the volume AZ does not match the [DEFAULT]/default_availability_zone then we put the volume AZ in the request spec as if the user requested that AZ when creating the server. Since this is a change in how cross_az_attach is used and how the instance default AZ works when using BDMs for pre-existing volumes, the docs are updated and a release note is added. Note that not all of the API code paths are unit tested because the functional test coverage does most of the heavy lifting for coverage. Given the amount of unit tests that are impacted by this change, it is pretty obvious that (1) many unit tests are mocking at too low a level and (2) functional tests are better for validating these flows. Closes-Bug: #1694844 Change-Id: Ib31ba2cbff0ebb22503172d8801b6e0c3d2aa68a
157 lines
7.8 KiB
Python
157 lines
7.8 KiB
Python
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import six
|
|
|
|
from nova import test
|
|
from nova.tests import fixtures as nova_fixtures
|
|
from nova.tests.functional.api import client as api_client
|
|
from nova.tests.functional import fixtures as func_fixtures
|
|
from nova.tests.functional import integrated_helpers
|
|
from nova.tests.unit.image import fake as fake_image
|
|
from nova.tests.unit import policy_fixture
|
|
|
|
|
|
class CrossAZAttachTestCase(test.TestCase,
|
|
integrated_helpers.InstanceHelperMixin):
|
|
"""Contains various scenarios for the [cinder]/cross_az_attach option
|
|
and how it affects interactions between nova and cinder in the API and
|
|
compute service.
|
|
"""
|
|
az = 'us-central-1'
|
|
|
|
def setUp(self):
|
|
super(CrossAZAttachTestCase, self).setUp()
|
|
# Use the standard fixtures.
|
|
self.useFixture(policy_fixture.RealPolicyFixture())
|
|
self.useFixture(nova_fixtures.CinderFixture(self, az=self.az))
|
|
self.useFixture(nova_fixtures.NeutronFixture(self))
|
|
self.useFixture(func_fixtures.PlacementFixture())
|
|
fake_image.stub_out_image_service(self)
|
|
self.addCleanup(fake_image.FakeImageService_reset)
|
|
# Start nova controller services.
|
|
self.api = self.useFixture(nova_fixtures.OSAPIFixture(
|
|
api_version='v2.1')).admin_api
|
|
self.start_service('conductor')
|
|
self.start_service('scheduler')
|
|
# Start one compute service and add it to the AZ. This allows us to
|
|
# get past the AvailabilityZoneFilter and build a server.
|
|
self.start_service('compute', host='host1')
|
|
agg_id = self.api.post_aggregate({'aggregate': {
|
|
'name': self.az, 'availability_zone': self.az}})['id']
|
|
self.api.api_post('/os-aggregates/%s/action' % agg_id,
|
|
{'add_host': {'host': 'host1'}})
|
|
|
|
def test_cross_az_attach_false_boot_from_volume_no_az_specified(self):
|
|
"""Tests the scenario where [cinder]/cross_az_attach=False and the
|
|
server is created with a pre-existing volume but the server create
|
|
request does not specify an AZ nor is [DEFAULT]/default_schedule_zone
|
|
set. In this case the server is created in the zone specified by the
|
|
volume.
|
|
"""
|
|
self.flags(cross_az_attach=False, group='cinder')
|
|
server = self._build_minimal_create_server_request(
|
|
self.api,
|
|
'test_cross_az_attach_false_boot_from_volume_no_az_specified')
|
|
del server['imageRef'] # Do not need imageRef for boot from volume.
|
|
server['block_device_mapping_v2'] = [{
|
|
'source_type': 'volume',
|
|
'destination_type': 'volume',
|
|
'boot_index': 0,
|
|
'uuid': nova_fixtures.CinderFixture.IMAGE_BACKED_VOL
|
|
}]
|
|
server = self.api.post_server({'server': server})
|
|
server = self._wait_for_state_change(self.api, server, 'ACTIVE')
|
|
self.assertEqual(self.az, server['OS-EXT-AZ:availability_zone'])
|
|
|
|
def test_cross_az_attach_false_data_volume_no_az_specified(self):
|
|
"""Tests the scenario where [cinder]/cross_az_attach=False and the
|
|
server is created with a pre-existing volume as a non-boot data volume
|
|
but the server create request does not specify an AZ nor is
|
|
[DEFAULT]/default_schedule_zone set. In this case the server is created
|
|
in the zone specified by the non-root data volume.
|
|
"""
|
|
self.flags(cross_az_attach=False, group='cinder')
|
|
server = self._build_minimal_create_server_request(
|
|
self.api,
|
|
'test_cross_az_attach_false_data_volume_no_az_specified')
|
|
# Note that we use the legacy block_device_mapping parameter rather
|
|
# than block_device_mapping_v2 because that will create an implicit
|
|
# source_type=image, destination_type=local, boot_index=0,
|
|
# uuid=$imageRef which is used as the root BDM and allows our
|
|
# non-boot data volume to be attached during server create. Otherwise
|
|
# we get InvalidBDMBootSequence.
|
|
server['block_device_mapping'] = [{
|
|
# This is a non-bootable volume in the CinderFixture.
|
|
'volume_id': nova_fixtures.CinderFixture.SWAP_OLD_VOL
|
|
}]
|
|
server = self.api.post_server({'server': server})
|
|
server = self._wait_for_state_change(self.api, server, 'ACTIVE')
|
|
self.assertEqual(self.az, server['OS-EXT-AZ:availability_zone'])
|
|
|
|
def test_cross_az_attach_false_boot_from_volume_default_zone_match(self):
|
|
"""Tests the scenario where [cinder]/cross_az_attach=False and the
|
|
server is created with a pre-existing volume and the
|
|
[DEFAULT]/default_schedule_zone matches the volume's AZ.
|
|
"""
|
|
self.flags(cross_az_attach=False, group='cinder')
|
|
self.flags(default_schedule_zone=self.az)
|
|
server = self._build_minimal_create_server_request(
|
|
self.api,
|
|
'test_cross_az_attach_false_boot_from_volume_default_zone_match')
|
|
del server['imageRef'] # Do not need imageRef for boot from volume.
|
|
server['block_device_mapping_v2'] = [{
|
|
'source_type': 'volume',
|
|
'destination_type': 'volume',
|
|
'boot_index': 0,
|
|
'uuid': nova_fixtures.CinderFixture.IMAGE_BACKED_VOL
|
|
}]
|
|
server = self.api.post_server({'server': server})
|
|
server = self._wait_for_state_change(self.api, server, 'ACTIVE')
|
|
self.assertEqual(self.az, server['OS-EXT-AZ:availability_zone'])
|
|
|
|
def test_cross_az_attach_false_bfv_az_specified_mismatch(self):
|
|
"""Negative test where the server is being created in a specific AZ
|
|
that does not match the volume being attached which results in a 400
|
|
error response.
|
|
"""
|
|
self.flags(cross_az_attach=False, group='cinder')
|
|
server = self._build_minimal_create_server_request(
|
|
self.api, 'test_cross_az_attach_false_bfv_az_specified_mismatch',
|
|
az='london')
|
|
del server['imageRef'] # Do not need imageRef for boot from volume.
|
|
server['block_device_mapping_v2'] = [{
|
|
'source_type': 'volume',
|
|
'destination_type': 'volume',
|
|
'boot_index': 0,
|
|
'uuid': nova_fixtures.CinderFixture.IMAGE_BACKED_VOL
|
|
}]
|
|
# Use the AZ fixture to fake out the london AZ.
|
|
with nova_fixtures.AvailabilityZoneFixture(zones=['london', self.az]):
|
|
ex = self.assertRaises(api_client.OpenStackApiException,
|
|
self.api.post_server, {'server': server})
|
|
self.assertEqual(400, ex.response.status_code)
|
|
self.assertIn('Server and volumes are not in the same availability '
|
|
'zone. Server is in: london. Volumes are in: %s' %
|
|
self.az, six.text_type(ex))
|
|
|
|
def test_cross_az_attach_false_no_volumes(self):
|
|
"""A simple test to make sure cross_az_attach=False API validation is
|
|
a noop if there are no volumes in the server create request.
|
|
"""
|
|
self.flags(cross_az_attach=False, group='cinder')
|
|
server = self._build_minimal_create_server_request(
|
|
self.api, 'test_cross_az_attach_false_no_volumes', az=self.az)
|
|
server = self.api.post_server({'server': server})
|
|
server = self._wait_for_state_change(self.api, server, 'ACTIVE')
|
|
self.assertEqual(self.az, server['OS-EXT-AZ:availability_zone'])
|