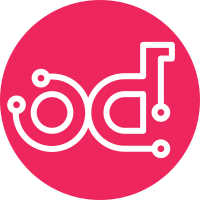
Cherry-pick check script validates the proposed patch's commit message. If a patch is not on top of the given branch then Zuul rebases it to the top and the patch becomes a merge patch. In this case the script validates the merge patch's commit message instead of the original patch's commit message and fails. This fix selects the parent of the patch if it is a merge patch. Change-Id: I8e4e5afc773d53dee9c1c24951bb07a45ddc2f1a
43 lines
1.4 KiB
Bash
Executable File
43 lines
1.4 KiB
Bash
Executable File
#!/bin/sh
|
|
#
|
|
# A tool to check the cherry-pick hashes from the current git commit message
|
|
# to verify that they're all on either master or stable/ branches
|
|
#
|
|
|
|
commit_hash=""
|
|
|
|
# Check if the patch is a merge patch by counting the number of parents.
|
|
# If the patch has 2 parents, then the 2nd parent is the patch we want
|
|
# to validate.
|
|
parent_number=$(git show --format='%P' --quiet | awk '{print NF}')
|
|
if [ $parent_number -eq 2 ]; then
|
|
commit_hash=$(git show --format='%P' --quiet | awk '{print $NF}')
|
|
fi
|
|
|
|
hashes=$(git show --format='%b' --quiet $commit_hash | sed -nr 's/^.cherry picked from commit (.*).$/\1/p')
|
|
checked=0
|
|
branches+=""
|
|
for hash in $hashes; do
|
|
branch=$(git branch -a --contains "$hash" 2>/dev/null| grep -oE '(master|stable/[a-z]+)')
|
|
if [ $? -ne 0 ]; then
|
|
echo "Cherry pick hash $hash not on any master or stable branches"
|
|
exit 1
|
|
fi
|
|
branches+=" $branch"
|
|
checked=$(($checked + 1))
|
|
done
|
|
|
|
if [ $checked -eq 0 ]; then
|
|
if ! grep -q '^defaultbranch=stable/' .gitreview; then
|
|
echo "Checked $checked cherry-pick hashes: OK"
|
|
exit 0
|
|
else
|
|
if ! git show --format='%B' --quiet | grep -qi 'stable.*only'; then
|
|
echo 'Stable branch requires either cherry-pick -x headers or [stable-only] tag!'
|
|
exit 1
|
|
fi
|
|
fi
|
|
else
|
|
echo Checked $checked cherry-pick hashes on branches: $(echo $branches | tr ' ' '\n' | sort | uniq)
|
|
fi
|