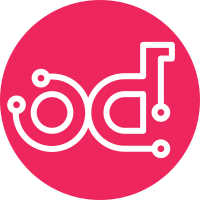
Enable users to define the policy rules on server group policy to meet more advanced policy requirement. This microversion brings the following changes in server group APIs: * Add ``policy`` and ``rules`` fields in the request of POST ``/os-server-groups``. * The ``policy`` and ``rules`` fields will be returned in response body of POST, GET ``/os-server-groups`` API and GET ``/os-server-groups/{server_group_id}`` API. * The ``policies`` and ``metadata`` fields have been removed from the response body of POST, GET ``/os-server-groups`` API and GET ``/os-server-groups/{server_group_id}`` API. Part of blueprint: complex-anti-affinity-policies Change-Id: I6911e97bd7f8df92511e90518dba21c127e106a5
86 lines
3.2 KiB
Python
86 lines
3.2 KiB
Python
# Copyright 2014 NEC Corporation. All rights reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
import copy
|
|
|
|
from nova.api.validation import parameter_types
|
|
|
|
# NOTE(russellb) There is one other policy, 'legacy', but we don't allow that
|
|
# being set via the API. It's only used when a group gets automatically
|
|
# created to support the legacy behavior of the 'group' scheduler hint.
|
|
create = {
|
|
'type': 'object',
|
|
'properties': {
|
|
'server_group': {
|
|
'type': 'object',
|
|
'properties': {
|
|
'name': parameter_types.name,
|
|
'policies': {
|
|
# This allows only a single item and it must be one of the
|
|
# enumerated values. So this is really just a single string
|
|
# value, but for legacy reasons is an array. We could
|
|
# probably change the type from array to string with a
|
|
# microversion at some point but it's very low priority.
|
|
'type': 'array',
|
|
'items': [{
|
|
'type': 'string',
|
|
'enum': ['anti-affinity', 'affinity']}],
|
|
'uniqueItems': True,
|
|
'additionalItems': False,
|
|
}
|
|
},
|
|
'required': ['name', 'policies'],
|
|
'additionalProperties': False,
|
|
}
|
|
},
|
|
'required': ['server_group'],
|
|
'additionalProperties': False,
|
|
}
|
|
|
|
create_v215 = copy.deepcopy(create)
|
|
policies = create_v215['properties']['server_group']['properties']['policies']
|
|
policies['items'][0]['enum'].extend(['soft-anti-affinity', 'soft-affinity'])
|
|
|
|
create_v264 = copy.deepcopy(create_v215)
|
|
del create_v264['properties']['server_group']['properties']['policies']
|
|
sg_properties = create_v264['properties']['server_group']
|
|
sg_properties['required'].remove('policies')
|
|
sg_properties['required'].append('policy')
|
|
sg_properties['properties']['policy'] = {
|
|
'type': 'string',
|
|
'enum': ['anti-affinity', 'affinity',
|
|
'soft-anti-affinity', 'soft-affinity'],
|
|
}
|
|
|
|
sg_properties['properties']['rules'] = {
|
|
'type': 'object',
|
|
'properties': {
|
|
'max_server_per_host':
|
|
parameter_types.positive_integer,
|
|
},
|
|
'additionalProperties': False,
|
|
}
|
|
|
|
server_groups_query_param = {
|
|
'type': 'object',
|
|
'properties': {
|
|
'all_projects': parameter_types.multi_params({'type': 'string'}),
|
|
'limit': parameter_types.multi_params(
|
|
parameter_types.non_negative_integer),
|
|
'offset': parameter_types.multi_params(
|
|
parameter_types.non_negative_integer),
|
|
},
|
|
# For backward compatible changes
|
|
'additionalProperties': True
|
|
}
|