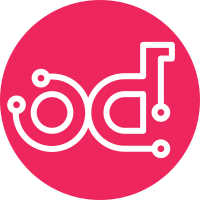
As per the new direction, we will move all the system level policies to system admin even GET policies. system reader will be added in next phase in future cycle. To dissociate the scope checks form the new defaults, check_str is added as 'admin' rule (role:admin) without 'system:all'. So that policy with that admin rule and scope_type as 'system' works like: - with enforce_scope=false, legacy or project admin still able to access the system level APIs. - with enforce_scope=True, only system user with admin role can access the system level APIs. Also modifying and adding tests for four cases: 1. enforce_scope=False + legacy rule (current default policies) 2. enforce_scope=False + No legacy rule 3. enforce_scope=True + legacy rule 4. enforce_scope=True + no legacy rule (end goal of new RBAC) Partial implement blueprint policy-defaults-refresh-2 Change-Id: I344276d2ab054311a4b6c34c6998e116e7507246
131 lines
4.0 KiB
Python
131 lines
4.0 KiB
Python
# Copyright 2016 Cloudbase Solutions Srl
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from oslo_policy import policy
|
|
|
|
from nova.policies import base
|
|
|
|
|
|
BASE_POLICY_NAME = 'os_compute_api:os-hypervisors:%s'
|
|
|
|
DEPRECATED_REASON = """
|
|
Nova API policies are introducing new default roles with scope_type
|
|
capabilities. Old policies are deprecated and silently going to be ignored
|
|
in nova 23.0.0 release.
|
|
"""
|
|
|
|
DEPRECATED_POLICY = policy.DeprecatedRule(
|
|
'os_compute_api:os-hypervisors',
|
|
base.RULE_ADMIN_API,
|
|
deprecated_reason=DEPRECATED_REASON,
|
|
deprecated_since='21.0.0'
|
|
)
|
|
|
|
|
|
hypervisors_policies = [
|
|
policy.DocumentedRuleDefault(
|
|
name=BASE_POLICY_NAME % 'list',
|
|
check_str=base.ADMIN,
|
|
description="List all hypervisors.",
|
|
operations=[
|
|
{
|
|
'path': '/os-hypervisors',
|
|
'method': 'GET'
|
|
},
|
|
],
|
|
scope_types=['system'],
|
|
deprecated_rule=DEPRECATED_POLICY),
|
|
policy.DocumentedRuleDefault(
|
|
name=BASE_POLICY_NAME % 'list-detail',
|
|
check_str=base.ADMIN,
|
|
description="List all hypervisors with details",
|
|
operations=[
|
|
{
|
|
'path': '/os-hypervisors/details',
|
|
'method': 'GET'
|
|
},
|
|
],
|
|
scope_types=['system'],
|
|
deprecated_rule=DEPRECATED_POLICY),
|
|
policy.DocumentedRuleDefault(
|
|
name=BASE_POLICY_NAME % 'statistics',
|
|
check_str=base.ADMIN,
|
|
description="Show summary statistics for all hypervisors "
|
|
"over all compute nodes.",
|
|
operations=[
|
|
{
|
|
'path': '/os-hypervisors/statistics',
|
|
'method': 'GET'
|
|
},
|
|
],
|
|
scope_types=['system'],
|
|
deprecated_rule=DEPRECATED_POLICY),
|
|
policy.DocumentedRuleDefault(
|
|
name=BASE_POLICY_NAME % 'show',
|
|
check_str=base.ADMIN,
|
|
description="Show details for a hypervisor.",
|
|
operations=[
|
|
{
|
|
'path': '/os-hypervisors/{hypervisor_id}',
|
|
'method': 'GET'
|
|
},
|
|
],
|
|
scope_types=['system'],
|
|
deprecated_rule=DEPRECATED_POLICY),
|
|
policy.DocumentedRuleDefault(
|
|
name=BASE_POLICY_NAME % 'uptime',
|
|
check_str=base.ADMIN,
|
|
description="Show the uptime of a hypervisor.",
|
|
operations=[
|
|
{
|
|
'path': '/os-hypervisors/{hypervisor_id}/uptime',
|
|
'method': 'GET'
|
|
},
|
|
],
|
|
scope_types=['system'],
|
|
deprecated_rule=DEPRECATED_POLICY),
|
|
policy.DocumentedRuleDefault(
|
|
name=BASE_POLICY_NAME % 'search',
|
|
check_str=base.ADMIN,
|
|
description="Search hypervisor by hypervisor_hostname pattern.",
|
|
operations=[
|
|
{
|
|
'path': '/os-hypervisors/{hypervisor_hostname_pattern}/search',
|
|
'method': 'GET'
|
|
},
|
|
],
|
|
scope_types=['system'],
|
|
deprecated_rule=DEPRECATED_POLICY),
|
|
policy.DocumentedRuleDefault(
|
|
name=BASE_POLICY_NAME % 'servers',
|
|
check_str=base.ADMIN,
|
|
description="List all servers on hypervisors that can match "
|
|
"the provided hypervisor_hostname pattern.",
|
|
operations=[
|
|
{
|
|
'path':
|
|
'/os-hypervisors/{hypervisor_hostname_pattern}/servers',
|
|
'method': 'GET'
|
|
}
|
|
],
|
|
scope_types=['system'],
|
|
deprecated_rule=DEPRECATED_POLICY
|
|
),
|
|
]
|
|
|
|
|
|
def list_rules():
|
|
return hypervisors_policies
|