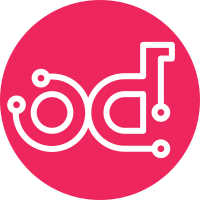
To support this, I've added a fake libvirt implementation. It's supposed to expose an API and behaviour identical to that of libvirt itself except without actually running any VM's or setting up any firewall or anything, but still responding correctly when asked for a domain's XML, a list of defined domains, running domains, etc. I've also split out everything from libvirt.connection that is potentially destructive or otherwise undesirable to run during testing, and moved it to a new nova.virt.libvirt.utils. I added tests for those things separately as well as stub version of it for testing. I hope eventually to make it similar to fakelibvirt in style (e.g. keep track of files created and deleted and attempts to open a file that it doesn't know about, you'll get proper exceptions with proper errnos set and whatnot). Change-Id: Id90b260933e3443b4ffb3b29e4bc0cbc82c19ba6
105 lines
2.0 KiB
Python
105 lines
2.0 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright (c) 2011 OpenStack LLC
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import StringIO
|
|
|
|
files = {}
|
|
disk_sizes = {}
|
|
disk_backing_files = {}
|
|
|
|
|
|
def create_image(disk_format, path, size):
|
|
pass
|
|
|
|
|
|
def create_cow_image(backing_file, path):
|
|
pass
|
|
|
|
|
|
def get_disk_size(path):
|
|
return disk_sizes.get(path, 1024 * 1024 * 20)
|
|
|
|
|
|
def get_backing_file(path):
|
|
return disk_backing_files.get(path, None)
|
|
|
|
|
|
def copy_image(src, dest):
|
|
pass
|
|
|
|
|
|
def mkfs(fs, path):
|
|
pass
|
|
|
|
|
|
def ensure_tree(path):
|
|
pass
|
|
|
|
|
|
def write_to_file(path, contents, umask=None):
|
|
pass
|
|
|
|
|
|
def chown(path, owner):
|
|
pass
|
|
|
|
|
|
def extract_snapshot(disk_path, source_fmt, snapshot_name, out_path, dest_fmt):
|
|
files[out_path] = ''
|
|
|
|
|
|
class File(object):
|
|
def __init__(self, path, mode=None):
|
|
self.fp = StringIO.StringIO(files[path])
|
|
|
|
def __enter__(self):
|
|
return self.fp
|
|
|
|
def __exit__(self, *args):
|
|
return
|
|
|
|
|
|
def file_open(path, mode=None):
|
|
return File(path, mode)
|
|
|
|
|
|
def load_file(path):
|
|
return ''
|
|
|
|
|
|
def file_delete(path):
|
|
return True
|
|
|
|
|
|
def get_open_port(start_port, end_port):
|
|
# Return the port in the middle
|
|
return int((start_port + end_port) / 2)
|
|
|
|
|
|
def run_ajaxterm(cmd, token, port):
|
|
pass
|
|
|
|
|
|
def get_fs_info(path):
|
|
return {'total': 128 * (1024 ** 3),
|
|
'used': 44 * (1024 ** 3),
|
|
'free': 84 * (1024 ** 3)}
|
|
|
|
|
|
def fetch_image(context, target, image_id, user_id, project_id,
|
|
size=None):
|
|
pass
|