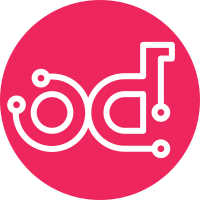
Standard practice is for doc comment strings to be enclosed in """ rather than '''. bin/nova-{novncproxy,spicehtml5proxy} were exceptions to this. Change-Id: I689063af2a51b26c971336b6fdbe60d5695b3ebc Signed-off-by: Daniel P. Berrange <berrange@redhat.com>
96 lines
3.4 KiB
Python
Executable File
96 lines
3.4 KiB
Python
Executable File
#!/usr/bin/env python
|
|
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright (c) 2012 OpenStack, LLC.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
Websocket proxy that is compatible with OpenStack Nova
|
|
noVNC consoles. Leverages websockify.py by Joel Martin
|
|
"""
|
|
|
|
import os
|
|
import sys
|
|
|
|
from nova import config
|
|
from nova.console import websocketproxy
|
|
from nova.openstack.common import cfg
|
|
|
|
|
|
opts = [
|
|
cfg.BoolOpt('record',
|
|
default=False,
|
|
help='Record sessions to FILE.[session_number]'),
|
|
cfg.BoolOpt('daemon',
|
|
default=False,
|
|
help='Become a daemon (background process)'),
|
|
cfg.BoolOpt('ssl_only',
|
|
default=False,
|
|
help='Disallow non-encrypted connections'),
|
|
cfg.BoolOpt('source_is_ipv6',
|
|
default=False,
|
|
help='Source is ipv6'),
|
|
cfg.StrOpt('cert',
|
|
default='self.pem',
|
|
help='SSL certificate file'),
|
|
cfg.StrOpt('key',
|
|
default=None,
|
|
help='SSL key file (if separate from cert)'),
|
|
cfg.StrOpt('web',
|
|
default='/usr/share/novnc',
|
|
help='Run webserver on same port. Serve files from DIR.'),
|
|
cfg.StrOpt('novncproxy_host',
|
|
default='0.0.0.0',
|
|
help='Host on which to listen for incoming requests'),
|
|
cfg.IntOpt('novncproxy_port',
|
|
default=6080,
|
|
help='Port on which to listen for incoming requests'),
|
|
]
|
|
|
|
CONF = cfg.CONF
|
|
CONF.register_cli_opts(opts)
|
|
CONF.import_opt('debug', 'nova.openstack.common.log')
|
|
|
|
|
|
if __name__ == '__main__':
|
|
if CONF.ssl_only and not os.path.exists(CONF.cert):
|
|
parser.error("SSL only and %s not found" % CONF.cert)
|
|
|
|
# Setup flags
|
|
config.parse_args(sys.argv)
|
|
|
|
# Check to see if novnc html/js/css files are present
|
|
if not os.path.exists(CONF.web):
|
|
print "Can not find novnc html/js/css files at %s." % CONF.web
|
|
sys.exit(-1)
|
|
|
|
# Create and start the NovaWebSockets proxy
|
|
server = websocketproxy.NovaWebSocketProxy(
|
|
listen_host=CONF.novncproxy_host,
|
|
listen_port=CONF.novncproxy_port,
|
|
source_is_ipv6=CONF.source_is_ipv6,
|
|
verbose=CONF.verbose,
|
|
cert=CONF.cert,
|
|
key=CONF.key,
|
|
ssl_only=CONF.ssl_only,
|
|
daemon=CONF.daemon,
|
|
record=CONF.record,
|
|
web=CONF.web,
|
|
target_host='ignore',
|
|
target_port='ignore',
|
|
wrap_mode='exit',
|
|
wrap_cmd=None)
|
|
server.start_server()
|