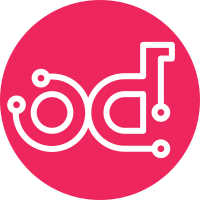
This makes scheduling weights more plugin friendly and creates shared code that can be used by the host scheduler as well as the future cells scheduler. Weighing classes can now be specified much like you can specify scheduling host filters. The new weights code reverses the old behavior where lower weights win. Higher weights are now the winners. The least_cost module and configs have been deprecated, but are still supported for backwards compatibility. The code has moved to nova.scheduler.weights.least_cost and been modified to work with the new loadable-class code. If any of the least_cost related config options are specified, this least_cost weigher will be used. For those not overriding the default least_cost config values, the new RamWeigher class will be used. The default behavior of the RamWeigher class is the same default behavior as the old least_cost module. The new weights code introduces a new config option 'scheduler_weight_classes' which is used to specify which weigher classes to use. The default is 'all classes', but modified if least_cost deprecated config options are used, as mentioned above. The RamWeigher class introduces a new config option 'ram_weight_multiplier'. The default of 1.0 causes weights equal to the free memory in MB to be returned, thus hosts with more free memory are preferred (causes equal spreading). Changing this value to a negative number such as -1.0 will cause reverse behavior (fill first). DocImpact Change-Id: I1e5e5039c299db02f7287f2d33299ebf0b9732ce
72 lines
2.3 KiB
Python
72 lines
2.3 KiB
Python
# Copyright (c) 2011-2012 OpenStack, LLC.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""
|
|
Pluggable Weighing support
|
|
"""
|
|
|
|
from nova import loadables
|
|
|
|
|
|
class WeighedObject(object):
|
|
"""Object with weight information."""
|
|
def __init__(self, obj, weight):
|
|
self.obj = obj
|
|
self.weight = weight
|
|
|
|
def __repr__(self):
|
|
return "<WeighedObject '%s': %s>" % (self.obj, self.weight)
|
|
|
|
|
|
class BaseWeigher(object):
|
|
"""Base class for pluggable weighers."""
|
|
def _weight_multiplier(self):
|
|
"""How weighted this weigher should be. Normally this would
|
|
be overriden in a subclass based on a config value.
|
|
"""
|
|
return 1.0
|
|
|
|
def _weigh_object(self, obj, weight_properties):
|
|
"""Override in a subclass to specify a weight for a specific
|
|
object.
|
|
"""
|
|
return 0.0
|
|
|
|
def weigh_objects(self, weighed_obj_list, weight_properties):
|
|
"""Weigh multiple objects. Override in a subclass if you need
|
|
need access to all objects in order to manipulate weights.
|
|
"""
|
|
for obj in weighed_obj_list:
|
|
obj.weight += (self._weight_multiplier() *
|
|
self._weigh_object(obj.obj, weight_properties))
|
|
|
|
|
|
class BaseWeightHandler(loadables.BaseLoader):
|
|
object_class = WeighedObject
|
|
|
|
def get_weighed_objects(self, weigher_classes, obj_list,
|
|
weighing_properties):
|
|
"""Return a sorted (highest score first) list of WeighedObjects."""
|
|
|
|
if not obj_list:
|
|
return []
|
|
|
|
weighed_objs = [self.object_class(obj, 0.0) for obj in obj_list]
|
|
for weigher_cls in weigher_classes:
|
|
weigher = weigher_cls()
|
|
weigher.weigh_objects(weighed_objs, weighing_properties)
|
|
|
|
return sorted(weighed_objs, key=lambda x: x.weight, reverse=True)
|