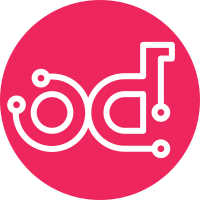
This PS provides the same level of configuration tuneability and control to the ceph chart as other charts within openstack-helm. Change-Id: I620c3fdb31abe67ee5b4b4766b1523e02bb7f814
86 lines
2.2 KiB
Smarty
86 lines
2.2 KiB
Smarty
#!/bin/bash
|
|
set -ex
|
|
|
|
# log arguments with timestamp
|
|
function log {
|
|
if [ -z "$*" ]; then
|
|
return 1
|
|
fi
|
|
|
|
TIMESTAMP=$(date '+%F %T')
|
|
echo "${TIMESTAMP} $0: $*"
|
|
return 0
|
|
}
|
|
|
|
# Given two strings, return the length of the shared prefix
|
|
function prefix_length {
|
|
local maxlen=${#1}
|
|
for ((i=maxlen-1;i>=0;i--)); do
|
|
if [[ "${1:0:i}" == "${2:0:i}" ]]; then
|
|
echo $i
|
|
return
|
|
fi
|
|
done
|
|
}
|
|
|
|
# Test if a command line tool is available
|
|
function is_available {
|
|
command -v $@ &>/dev/null
|
|
}
|
|
|
|
# Calculate proper device names, given a device and partition number
|
|
function dev_part {
|
|
local osd_device=${1}
|
|
local osd_partition=${2}
|
|
|
|
if [[ -L ${osd_device} ]]; then
|
|
# This device is a symlink. Work out it's actual device
|
|
local actual_device=$(readlink -f ${osd_device})
|
|
local bn=$(basename ${osd_device})
|
|
if [[ "${actual_device:0-1:1}" == [0-9] ]]; then
|
|
local desired_partition="${actual_device}p${osd_partition}"
|
|
else
|
|
local desired_partition="${actual_device}${osd_partition}"
|
|
fi
|
|
# Now search for a symlink in the directory of $osd_device
|
|
# that has the correct desired partition, and the longest
|
|
# shared prefix with the original symlink
|
|
local symdir=$(dirname ${osd_device})
|
|
local link=""
|
|
local pfxlen=0
|
|
for option in $(ls $symdir); do
|
|
if [[ $(readlink -f $symdir/$option) == $desired_partition ]]; then
|
|
local optprefixlen=$(prefix_length $option $bn)
|
|
if [[ $optprefixlen > $pfxlen ]]; then
|
|
link=$symdir/$option
|
|
pfxlen=$optprefixlen
|
|
fi
|
|
fi
|
|
done
|
|
if [[ $pfxlen -eq 0 ]]; then
|
|
>&2 log "Could not locate appropriate symlink for partition ${osd_partition} of ${osd_device}"
|
|
exit 1
|
|
fi
|
|
echo "$link"
|
|
elif [[ "${osd_device:0-1:1}" == [0-9] ]]; then
|
|
echo "${osd_device}p${osd_partition}"
|
|
else
|
|
echo "${osd_device}${osd_partition}"
|
|
fi
|
|
}
|
|
|
|
# Wait for a file to exist, regardless of the type
|
|
function wait_for_file {
|
|
timeout 10 bash -c "while [ ! -e ${1} ]; do echo 'Waiting for ${1} to show up' && sleep 1 ; done"
|
|
}
|
|
|
|
function get_osd_path {
|
|
echo "$OSD_PATH_BASE-$1/"
|
|
}
|
|
|
|
# Bash substitution to remove everything before '='
|
|
# and only keep what is after
|
|
function extract_param {
|
|
echo "${1##*=}"
|
|
}
|