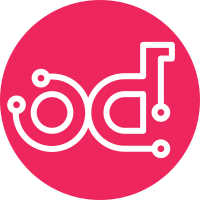
With https://review.openstack.org/#/c/144685 merged, the automatically generated configuration reference tables will be marked up as <option>option_name</option> = <replaceable>default_value</replaceable> As none of this should be translated (option_name is the same regardless of language), this patch updates generatepot to ignore option_name s tagged with <option> for translation. This should significantly reduce the number of strings required for translation from the common directory in particular, where many of the 8000-odd strings do not actually need translation. Change-Id: I7b46f4dd10c0855f86cea35417dcfde93cc113a3
93 lines
2.5 KiB
Python
Executable File
93 lines
2.5 KiB
Python
Executable File
#!/usr/bin/env python
|
|
'''
|
|
Created on 2012-7-3
|
|
|
|
@author: daisy
|
|
'''
|
|
import os, sys
|
|
from xml2po import Main
|
|
from xml2po.modes.docbook import docbookXmlMode
|
|
|
|
class myDocbookXmlMode(docbookXmlMode):
|
|
def __init__(self):
|
|
self.lists = ['itemizedlist', 'orderedlist', 'variablelist',
|
|
'segmentedlist', 'simplelist', 'calloutlist', 'varlistentry', 'userinput',
|
|
'computeroutput','prompt','command','screen']
|
|
self.objects = [ 'figure', 'textobject', 'imageobject', 'mediaobject',
|
|
'screenshot','literallayout', 'programlisting',
|
|
'option']
|
|
|
|
default_mode = 'docbook'
|
|
operation = 'pot'
|
|
options = {
|
|
'mark_untranslated' : False,
|
|
'expand_entities' : True,
|
|
'expand_all_entities' : False,
|
|
}
|
|
|
|
ignore_folder = {"docbkx-example", "training-guide"}
|
|
ignore_file = {"api-examples.xml"}
|
|
|
|
root = "./doc"
|
|
|
|
def generatePoT (folder):
|
|
if (folder==None) :
|
|
path = root
|
|
else :
|
|
generateSinglePoT(folder)
|
|
return
|
|
|
|
if (not os.path.isdir(path)) :
|
|
return
|
|
|
|
files = os.listdir(path)
|
|
for aFile in files :
|
|
if (not (aFile in ignore_folder)):
|
|
generateSinglePoT (aFile)
|
|
|
|
def generateSinglePoT(folder):
|
|
xmlfiles = []
|
|
abspath = os.path.join(root, folder)
|
|
if (os.path.isdir(abspath)) :
|
|
os.path.walk(abspath, get_all_xml, xmlfiles)
|
|
else:
|
|
return
|
|
|
|
if len(xmlfiles)>0 :
|
|
output = os.path.join(abspath,"locale")
|
|
if (not os.path.exists(output)) :
|
|
os.mkdir(output)
|
|
output = os.path.join(output, folder+".pot")
|
|
try:
|
|
xml2po_main = Main(default_mode, operation, output, options)
|
|
xml2po_main.current_mode = myDocbookXmlMode()
|
|
except IOError:
|
|
print "Error: cannot open aFile %s for writing." % (output)
|
|
sys.exit(5)
|
|
#print(xmlfiles)
|
|
#print(">>>outout: %s ", output)
|
|
xml2po_main.to_pot(xmlfiles)
|
|
|
|
def get_all_xml (sms, dr, flst):
|
|
if ((flst == "target") or (flst == "wadls")) :
|
|
return
|
|
if (dr.find("target")>-1) :
|
|
return
|
|
if (dr.find("wadls")>-1) :
|
|
return
|
|
|
|
for f in flst:
|
|
if (f.endswith(".xml") and (f != "pom.xml") and (not (f in ignore_file))) :
|
|
sms.append(os.path.join(dr,f))
|
|
|
|
def main():
|
|
try:
|
|
folder = sys.argv[1]
|
|
except:
|
|
folder = None
|
|
generatePoT(folder)
|
|
|
|
if __name__ == '__main__':
|
|
main()
|
|
|