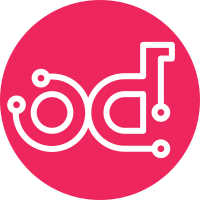
This patch added tools used for slicing and merging in translation process. The patch also includes the POT files for each documents. The PO files are not included, which can be downloaded from Transifex. Fixes: bug #1153415 Change-Id: Ia455134c99acb1129e8f10deda0d85867b1ef9a3
150 lines
4.3 KiB
Python
Executable File
150 lines
4.3 KiB
Python
Executable File
#!/usr/bin/env python
|
|
# -*- coding: utf-8 -*-
|
|
|
|
'''
|
|
Created on 2012-7-3
|
|
|
|
@author: daisy
|
|
'''
|
|
import os, sys
|
|
import tempfile
|
|
import shutil
|
|
import xml.dom.minidom
|
|
from optparse import OptionParser
|
|
import codecs
|
|
|
|
from xml2po import Main
|
|
default_mode = 'docbook'
|
|
operation = 'merge'
|
|
options = {
|
|
'mark_untranslated' : False,
|
|
'expand_entities' : True,
|
|
'expand_all_entities' : False,
|
|
}
|
|
|
|
ignore_folder = {"docbkx-example"}
|
|
root = "./doc/src/docbkx"
|
|
|
|
def mergeback (folder, language):
|
|
if (folder==None) :
|
|
path = root
|
|
else :
|
|
outputFiles = mergeSingleDocument(folder, language)
|
|
if ((outputFiles != None) and (len(outputFiles)>0)) :
|
|
for outXML in outputFiles :
|
|
changeXMLLangSetting (outXML, language)
|
|
return
|
|
|
|
if (not os.path.isdir(path)) :
|
|
return
|
|
|
|
files = os.listdir(path)
|
|
for aFile in files :
|
|
if (not (aFile in ignore_folder)):
|
|
outputFiles = mergeSingleDocument (aFile, language)
|
|
if ((outputFiles != None) and (len(outputFiles)>0)) :
|
|
for outXML in outputFiles :
|
|
changeXMLLangSetting (outXML, language)
|
|
|
|
def mergeSingleDocument(folder, language):
|
|
xmlfiles = []
|
|
outputfiles = []
|
|
abspath = os.path.join(root, folder)
|
|
if (os.path.isdir(abspath)) :
|
|
os.path.walk(abspath, get_xml_list, xmlfiles)
|
|
else:
|
|
return None
|
|
|
|
if len(xmlfiles)>0 :
|
|
popath = os.path.join(abspath,"locale",language+".po")
|
|
#generate MO file
|
|
mofile_handler, mofile_tmppath = tempfile.mkstemp()
|
|
os.close(mofile_handler)
|
|
os.system("msgfmt -o %s %s" % (mofile_tmppath, popath))
|
|
|
|
for aXML in xmlfiles :
|
|
#(filename, ext) = os.path.splitext(os.path.basename(aXML))
|
|
relpath = os.path.relpath(aXML, root)
|
|
outputpath = os.path.join(os.path.curdir, "generated", language, relpath)
|
|
try:
|
|
xml2po_main = Main(default_mode, operation, outputpath, options)
|
|
xml2po_main.merge(mofile_tmppath, aXML)
|
|
outputfiles.append(outputpath)
|
|
except IOError:
|
|
print "Error: cannot open aFile %s for writing."
|
|
sys.exit(5)
|
|
except :
|
|
print ("Exception happen")
|
|
if mofile_tmppath :
|
|
os.remove(mofile_tmppath)
|
|
|
|
return outputfiles
|
|
|
|
def changeXMLLangSetting(xmlFile, language):
|
|
dom = xml.dom.minidom.parse(xmlFile)
|
|
root = dom.documentElement
|
|
root.setAttribute("xml:lang", language[:2])
|
|
fileObj = codecs.open(xmlFile, "wb", encoding="utf-8")
|
|
|
|
#add namespace to link
|
|
nodelists = root.getElementsByTagName("link")
|
|
for node in nodelists :
|
|
if (node.hasAttribute("href")) :
|
|
node.setAttribute("xlink:href", node.getAttribute("href"))
|
|
|
|
dom.writexml(fileObj)
|
|
|
|
def get_xml_list (sms, dr, flst):
|
|
if ((flst == "target") or (flst == "wadls")) :
|
|
return
|
|
if (dr.find("target")>-1) :
|
|
return
|
|
if (dr.find("wadls")>-1) :
|
|
return
|
|
|
|
for f in flst:
|
|
if ((f.endswith(".xml") and (f != "pom.xml"))) :
|
|
sms.append(os.path.join(dr,f))
|
|
|
|
def main(argv):
|
|
|
|
usage = "usage: %prog [options] command [cmd_options]"
|
|
description = "This is the tool to generate translated docbooks, which"\
|
|
" will be stored in 'generated/[language]/"
|
|
|
|
parser = OptionParser(
|
|
usage=usage, version="0.6", description=description
|
|
)
|
|
parser.disable_interspersed_args()
|
|
parser.add_option(
|
|
"-l", "--language", dest="language", help=("specified language")
|
|
)
|
|
parser.add_option(
|
|
"-b", "--book", dest="book", help=("specified docbook")
|
|
)
|
|
(options, args) = parser.parse_args()
|
|
if (options.language == None) :
|
|
print "must specify language"
|
|
return
|
|
|
|
#change working directory
|
|
|
|
#copy folders
|
|
folder = options.book
|
|
language = options.language
|
|
sourcepath = os.path.join(root, folder)
|
|
destpath = os.path.join(os.path.curdir, "generated", language)
|
|
if (not os.path.exists(destpath)) :
|
|
os.makedirs(destpath)
|
|
|
|
destfolder = os.path.join(destpath, folder)
|
|
if (os.path.exists(destfolder)) :
|
|
shutil.rmtree(destfolder)
|
|
|
|
os.system("cp -r %s %s" % (sourcepath, destpath))
|
|
mergeback(folder, language)
|
|
|
|
if __name__ == '__main__':
|
|
main(sys.argv[1:])
|
|
|